Question
public static void main (String[] args) throws Exception { // ALWAYS TEST FIRST TO VERIFY USER PUT REQUIRED INPUT FILE NAME ON THE COMMAND LINE
public static void main (String[] args) throws Exception
{
// ALWAYS TEST FIRST TO VERIFY USER PUT REQUIRED INPUT FILE NAME ON THE COMMAND LINE
if (args.length < 1 )
{
System.out.println(" usage: C:\\> java Project6 dictionary.txt ");
System.exit(0);
}
String[] wordList = new String[INITIAL_CAPACITY];
int wordCount = 0;
BufferedReader infile = new BufferedReader( new FileReader(args[0]) );
int numOfUpsizes=0;
while ( infile.ready() ) // i.e. while there are more lines of text in the file
{
String word = infile.readLine();
if ( wordCount == wordList.length ) // an array's .length() is its CAPACITY, not count
{
wordList = upSize( wordList );
System.out.format( "after resize#%d length=%d, count=%d ",++numOfUpsizes,wordList.length,wordCount );
}
wordList[wordCount++] = word; // Now wordList has enough capacity
} //END WHILE INFILE READY
infile.close();
System.out.format( "after file closed: length=%d, count=%d ",wordList.length,wordCount );
wordList = downSize( wordList, wordCount );
System.out.format( "after final down size: length=%d, count=%d ",wordList.length,wordCount );
printFirstTenWords( wordList ); // IF THERE ARE LESS THAN 10 IN THE ARRAY THEN JUST PRINT THOSE
printLastTenWords( wordList ); // IF THERE ARE LESS THAN 10 IN THE ARRAY THEN JUST PRINT THOSE
} // END MAIN
// = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = = =
// DO NOT MODIFY THESE TWO METHODS
static void printFirstTenWords( String[] wordList )
{ final int MAX_WORDS_PER_LINE = 10;
int count = wordList.length for (int i=0 ; i System.out.print( wordList[i] + " "); System.out.println(); } static void printLastTenWords( String[] wordList ) { final int MAX_WORDS_PER_LINE = 10; int count = wordList.length for (int i=0 ; i System.out.print( wordList[wordList.length-count+i] + " "); System.out.println(); } // YOU FILL IN THESE METHODS BELOW static String[] upSize( String[] fullArr ) { return null; // REPLACE WITH YOUR CODE } static String[] downSize( String[] arr, int count ) { return null; // REPLACE WITH YOUR CODE }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
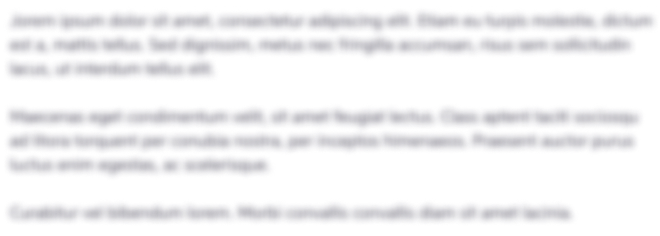
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started