Purpose: Demonstrate the ability to create and manipulate classes, data members, and member functions. This assignment also aims at creating a C++ project to handle
Purpose: Demonstrate the ability to create and manipulate classes, data members, and member functions. This assignment also aims at creating a C++ project to handle multiple files (one header file and two .cpp files) at the same time. Remember to follow documentation and variable name guidelines.
Create a C++ project to implement a simplified banking system.
Step 1:
Create a header file Bank.h to define a Bank class with the following members:
Data members: The program should implement data hiding, so please define data members accordingly.
- accountNumber
- name
- balance
Note: The data types are not specified for this problem, so you have flexibility to design the program in your way.
Member functions: Please include only the declaration of the member functions in the header file. The implementation of member functions will later be done in the Bank.cpp file. (You may inline get and set functions.)
Bank() constructor with no arguments creates a bank account with a default accountNumber as 9999, a name of an empty string, and balance value of zero.
Bank(param1, param2, param3) constructor with three parameters creates a bank account with a specified accountNumber, name and balance.
withdraw(param1) function to withdraw a specified amount (param1) from the account. The function should first check if there is sufficient balance in the account. If the balance is sufficient, withdrawal is processed and the current balance is returned as a string. Otherwise return a string to the caller that says Insufficient balance and leave the balance unchanged.
deposit(param1) function to deposit a specified amount of money (param1) to the account.
setName(param1) mutator function that changes the name on the account.
getAccountNumber(): An accessor function that returns the account number. This function is later called by the displayAccountInfo() function from inside another independent function called displayAccountInfo().
getName(): An accessor function that returns the name on the account.
getBalance(): An accessor function that returns the account balance. This function is later called by the displayAccountInfo() function from inside another independent function called displayAccountInfo().
Step 2:
Create a Bank.cpp file and implement the member functions of the Bank class.
Step 3:
Also define an independent displayAccountInfo(Bank obj) function which takes a Bank object (obj) as a parameter and displays the accountNumber, name, and balance of the bank account specified by this Bank object. The displayAccountInfo() function is later called by the main function.
Please note: The displayAccountInfo() function IS NOT a member function of the Bank class. It is an independent function that is called from inside the main function.
Step 4:
Finally, create a main.cpp file to do the following:
Display a menu with the following options:
Create Bank object with values for accountNumber, name, and balance
Create Bank object with no parameters.
Deposit to Bank account (request account number and amount)
Withdraw from Bank account (request account number and amount)
Display information for all accounts
Exit the program.
To test:
Create a bank account say accnt1- with no parameters. Try to withdraw $500 from this account.
Create another bank account say accnt2- with accountNumber: 1111, your name, and $1000 balance. Withdraw $600, then withdraw $300, and then deposit $400 to this account.
Display all account information, per Option 5.
Assumptions: There will never be more than 10 accounts. Show an error message if the user tries to deposit to or withdraw from an account number that doesnt exist. Show an error message if the user attempts to create an account number that already exists. Show any messages returned from the withdraw() function.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
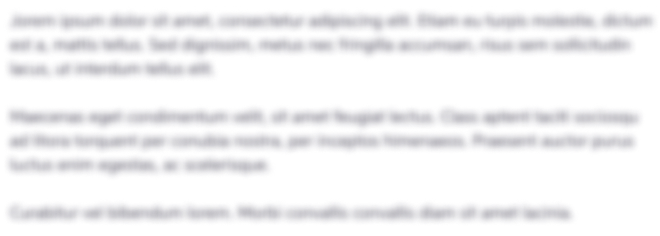
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started