Question
Purpose Purpose of this lab is for you to develop a class that is used by another class. Problem specification The TravelWallet class represents a
Purpose
Purpose of this lab is for you to develop a class that is used by another class.
Problem specification
The TravelWallet class represents a wallet for overseas travelers.
Instance variables
A travel wallet holds some amount of money in US dollars. It also holds some amount of money in the local currency. A wallet has an exchange rate, for when converting dollars to the local currency.
Methods
Every new wallet must always be created containing $100.0, 10,000.0 in the local currency and the exchange rate must be set.
Local currency can be spent from the wallet. A traveler can exchange dollars for the local currency. The total amount of money in the wallet in dollars can be calculated.
The TravelWallet class
You will write the new TravelWallet class. Here is a pseudocode outline:
3 private instance variables are required
-dollars
-local currency
-exchange rate
The exchange rate above is expressed as $1.0 will buy this much local currency. For example, an exchange rate of 15.0 means that $1.0 will buy 15.0 of the local currency.
4 public methods are required
constructor() method
-initializes ALL instance variables to the required starting values
-takes one parameter the exchange rate, that comes from Tester. See your lecture notes, example programs and textbook on how to make this parameter work
-(be careful initializing dollars and local for every new wallet)
spendLocal() method
-represents spending the local currency
-takes one parameter from Tester, the amount of local currency spent
-decreases the amount of the local currency
-must update the appropriate instance variable
exchangeDollarsToLocal() method
-represents exchanging dollars for the local currency
-takes one parameter from Tester, the amount in dollars to exchange
-decreases the amount of dollars
-increases the amount of local currency. Use the exchange rate instance variable to do this
-must update the two appropriate instance variables
totalAmountInDollars() method
-calculates the total amount of money in the wallet in dollars
-takes no parameters
-returns the calculated value to Tester. Use the exchange rate instance variable to calculate this. See your lecture notes, example programs and textbook on how to make return work
-(must not update any instance variables)
The Tester class
A Tester class is given to you to run your code. Tester uses TravelWallet, creating TravelWallet objects and calling TravelWalletmethods. You may not alter Tester in any way.
Tester class code below.
public class Tester { /** * main() method */ public static void main(String[] args) { // Tom visits Bali. Local currency is the Indonesian rupiah (Rp) TravelWallet tom = new TravelWallet(13563.0); // 1 US$ buys 13563.0 Rp tom.spendLocal(2500.0); // spend 2500.0 Rp tom.exchangeDollarsToLocal(75.0); // exchange $75.0 to rupiah // print money remaining, in US$ System.out.println("Tom has US$" + tom.totalAmountInDollars());
// Kay visits Hungary. Local currency is the forint (Ft) TravelWallet kay = new TravelWallet(250.77); // 1 US$ buys 250.77 Ft kay.spendLocal(7500.0); // spend 7500.0 Ft kay.exchangeDollarsToLocal(25.0); // exchange $25.0 to forints // print money remaining, in US$ System.out.println("Kay has US$" + kay.totalAmountInDollars()); } }
now use BlueJ to write and test your TravelWallet methods one at a time
-in the new TravelWallet class, first declare your instance variables
-finally, you will be able to compile Tester when all TravelWallet methods have been written and tested. Then run the Tester main()method, which runs your class, and output from the program appears in the Terminal Window. Check that this output is correct, then use Options | Save to file to save your output file as output.txt
if possible please post an image of code used or written if image is not readible. thanks
Step by Step Solution
There are 3 Steps involved in it
Step: 1
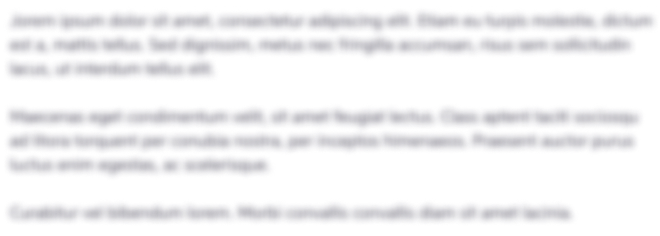
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started