Question
PURPOSE: The purpose of this lab is to develop hands-on familiarity with generic constructs. PROCEDURES: STEP 1: findMax implementation for all Numbers Consider the code,
PURPOSE: The purpose of this lab is to develop hands-on familiarity with generic constructs.
PROCEDURES: STEP 1: findMax implementation for all Numbers Consider the code, below, that calculates the maximum value in an array of integers. Implement a single method that will allow the user to find the maximum value of an array of Integers, Doubles, and other Numbers. For this step, dont use generics. Instead, write the method such that it takes an array of Numbers and returns a Number. Note that you cannot use the > operator on non-primitive values, and the Number class does not implement Comparable. One way to find out which of two Numbers is larger is to use the doubleValue method that is part of that class (see https://docs.oracle.com/javase/8/docs/api/java/lang/Number.html#doubleValue--). Name your method findMaxLab05Step01 and show its use in your driver/main method over different data types.
SAMPLE DEMONSTRATION CODE:
public static int findMax(int[] inputArray) {
int maxV = inputArray[0];
for (int element : inputArray) {
if (element > maxV) {
maxV = element;
}
// end-if }
// end-for
return maxV;
}
// end-method
STEP 2: Generic findMax implementation
Step 1 was an improvement over the integer-only version, but it is still less than ideal it would be better if our findMax method could find the maximum value amount any list of comparable objects rather than just Numbers. In this step, use generics to create a method called findMaxLab05Step02() that will find the maximum of any array of Comparable objects. In your driver class, show that your method works on at least two different types of Comparable objects including an example that uses type Integer and an example that uses a non-Number Comparable object that you define/create yourself.
STEP 3: Implementing Sequence data type Implement and create code that demonstrates/tests the Sequence class. The Sequence class will support the creation of a generic sequence of items. The items can be of any data type. A sequence is a simple object that allows a user to add items to the end of the sequence and retrieve an item or a subsequence of items. Your concrete implementation must use an array as the underlying data type, not an ArrayList or other pre-generated library solution. You may not use any API constructs that perform the functionality of Sequence.
The minimum set of public operations for a Sequence includes: (You are permitted to add helper methods as needed)
+Sequence(int capacity) // Constructs a sequence with a fixed maximum size (capacity)
+void add(Item item) // Adds item to the end of the sequence. Generates a // RuntimeException if called on a full Sequence.
+Item get(int pos) // Retrieve an item at position pos.
+Sequence getSequence(int pos, int len) // Retrieve a portion of a sequence // beginning at position pos for a length of len.
+int getCount(); // Returns the count of items in the sequence
+int getCapacity(); // Returns the capacity of the sequence +boolean equals(Sequence seq); // Returns true if this sequence and seq is // the same size and contain the same items in // the same order.
+Item findMax(); // Returns the largest item in Item[] +void print(); // Prints [ item1, item2, , itemn ] // Add other private methods as needed.
Note on testing: You can test your code either using functions calls in a main/driver method OR through JUnit testing. There will be no difference in evaluation/grading, but you are encouraged to use Junit tests, particularly if you have never used Junit tests. In Netbeans, you can create Junit test stubs by selecting a source file (or source/package directory), right clicking, and selecting Tools / Create Update Tests.
SAMPLE DEMONSTRATION/DRIVER CODE:
Sequence s1 = new Sequence(10); // One example of many
s1.add(a);
s1.add(c);
s1.add(b);
s1.add(s);
s1.add(a):
s1.add(c);
s1.add(b);
// Sequence s1 contains the strings a, c, b, s, a, c, b
String item = s1.get(3); // returns s
Sequence s2 = s1.getSequence(4,3); // returns a, c, b
Sequence s3 = s1.getSequence(0,3); // returns a, c, b
Sequence s4 = s1.getSequence(1,3); // returns c, b, s
System.out.println( s2.equals(s3) ); // displays true
System.out.println( s1.equals(s2) ); // displays false s2.print(); // prints [ a, c, b ]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
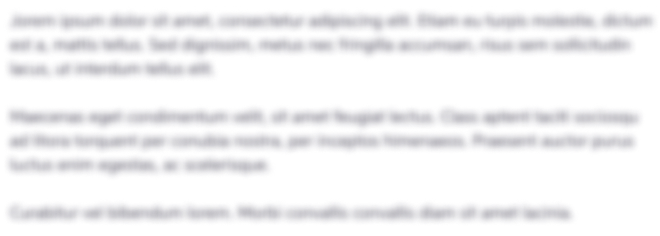
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started