Purpose: To write an Object-Oriented application that creates a Java class with several instance variables, a constructor to initialize the instance variables, and several methods
Purpose: To write an Object-Oriented application that creates a Java class with several instance variables, a constructor to initialize the instance variables, and several methods to access the instance variables values. Also, write a test class that instantiates the first class and tests the classs constructor and methods.
Details:
Create a class called Employee containing the following:
- Three instance variables,
- An instance variable of type String used to hold the employees first name.
- An instance variable of type String used to hold the employees last name.
- An instance variable of type double used to hold the employees monthly salary.
- Provide a constructor with three parameters used to initializes each instance variable. The constructor should check the specified monthly salary to ensure that it is positive. If it not, set it to 0.0.
- Provide get methods that return the values of each instance variables.
- No set methods are needed. Do not include them in the Employee class.
Create a second class called EmployeeTest that contains the main method. The method should prompt for an employees first name, last name, and monthly salary, and use the values to create an Employee object. The class should then prompt for the values a second time to create a second Employee object. Note: After asking for the first employees monthly salary, call the Sanner.nextLine method to clear the Sanners input buffer before asking for the second employees first name.
The EmployeeTest class should then display the following:
Employee Ones name: last_name, first_name
Employee Ones yearly salary: $ nn.nn
Employee Twos name: last_name, first_name
Employee Twos yearly salary: $ nn.nn
So far I have this
public class Employee {
private String firstName;
private String lastName;
private double monthlySalary;
public Employee(String firstName, String lastname, double monthlySalary) {
this.firstName = firstName;
this.lastName = lastName;
// ensure the monthlySalary is a positive value
if(monthlySalary> 0) {
this.monthlySalary = monthlySalary;
}
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName= lastName;
}
public double getSalary() {
return monthlySalary;
}
public void setSalary(double salary) {
if (monthlySalary>0) {
}
this.monthlySalary = 0.0;
this.monthlySalary = salary;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
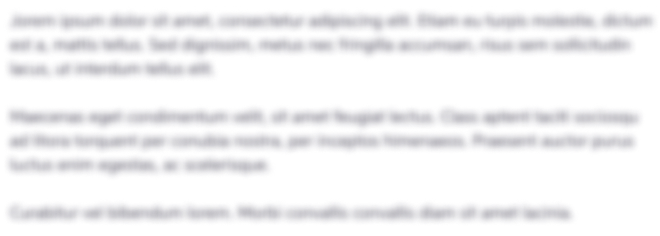
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started