Question
Pyhton ATM Simulation - GUI OBJECTIVES The main objectives of this project are to: Use the Python syntax to read user input, process information and
Pyhton ATM Simulation - GUI
OBJECTIVES
The main objectives of this project are to:
Use the Python syntax to read user input, process information and display output.
Declare and initialize variables using the Python variable naming conventions and keywords.
Understand the different data types used with the Python programming language.
Code decision-making structures and Boolean logic using Python.
Code repetition structures using Python.
Code selection and sequence structures using Python.
Define, declare and call void and value returning functions.
Pass arguments to functions.
Implement file input and output functions.
Use loops to process content of files.
Implement exception-handling constructs in Python.
Perform operations on sequences, lists and tuples.
Perform basic string operations using built-in string functions.
Test, search and manipulate strings.
Demonstrate and understanding of dictionaries and sets as Python objects.
Apply object oriented programming concepts to Python programming.
Use the Python functions, features and libraries to create graphical user interfaces.
Key Functions
The main functions of the ATM simulator may be summarized as follows:
Before performing any transaction, the user must enter his or her username and PIN (personal identification number) at an input screen/prompt (GUI or otherwise).
The system must validate the user information against the information that is pre-built into the system. For the purposes of this project, the system will have the following three username: PIN pairings to start.
User1: 1234
User2: 2222
User3: 3333
These can be stored in volatile (temporary) storage or in a file or series of files depending on whether you opt for the Basic Functionality or the Extended Functionality.
The prompt for the user information should allow for three attempts. If the user does not enter a valid username : PIN combination, the system must display an appropriate error message after each attempt and re-prompt. After the third invalid attempt, the system simply ends the program.
The names and PINs of users must be validated using data contained in the appropriate lists or files using the following criteria:
Username (String)
PIN (String 4 characters)
Once access has been authorized, the main transaction screen of the application should allow the user to carry out one of the following transactions:
Functionality of atm simulator
-Use files to store Usernames and PINS
-Must have an administrator login feature
-If user is administrator
-Menu options include :
Add new user
Delete user
Plot Account Balances
Exit
-If the user is a regular user
-The Menu options are
Account Balance
Withdraw
Deposit
Change PIN
Exit
all transactions will be carried out on a single account per user. There is no distinction between a chequing and a savings account.
After each transaction, with the exception of Exit, the system must return to the menu display and prompt the user for another transaction choice. In the case of the Administrator, upon exiting, the system should prompt for a new user and PIN
Deposit,
The user must enter the amount to be deposited into his or her account. The amount of the deposit must be added to the current balance into the account. Appropriate confirmation messages must be provided indicating the amount of the deposit and the new current balance. Proper data input validation to ensure that numerical data is input must be used along with error handling.
Keep in mind that if you are working with the Basic Functionality model, the balances are stored in the list, which will be lost once the program ends. If you use files to store the information, then the new balances should be saved and available when the program runs subsequent times.
Withdrawal,
The user must enter the amount to withdraw. Each withdrawal transaction is subject to a maximum of $1,000 per transaction. If the user enters an amount greater than $1000, the system must present a message indicating that $1000 is the maximum per withdrawal. The ATM accepts only transactions for which the amount entered is a multiple of $10. There is no daily maximum amount apart
from the users account balance in which case the system must also inform the user if the account balance is less than the transaction amount.
Account Balance
When the user selects this option, the system simply checks the data for that user and displays the current balance. Checking the account balance does not alter the balance itself.
Changing the PIN
Changing the PIN is available to all users. The difference in how this functions is dependant on how you set up the program. If you are using the Basic Functionality model using lists to store user information, the change in PIN will only be effective during the current running of the program. Once you quit the program and start it again all data values return to the original values. However if you are working with the Extended Functionality model and you are using files to store the information, the change in PIN will be committed to the file and be available for subsequent accesses using this new value.
Administrator Functions
The system administrator, as any other user, must enter his or her name and PIN (personal identification number) on the same input screen. The system administrator may perform only system transactions (he or she has no personal account).
The System Administrator must login with the unique User Name of SysAdmin and the PIN 1357. When these credentials are entered, the system must automatically display only the Administrator screen with its options
Add new user
Delete user
Exit
Add a new user
The administrator is the only user wo can create new user accounts. When this option is selected, the system must create a file or files for the new user to store the user information and account transactions and balances.
Error and exception handling
error and exception handling must be implemented wherever possible. Data validation must also be implemented. For example, when the system requires the user to enter a dollar amount, the system should make sure that the input is numeric and not alphabetic.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
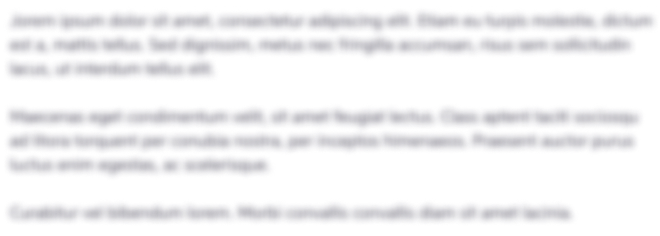
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started