Question
Python 3 Class Problem import numpy as np class Deck(): def __init__(self): Initializes the deck of cards >>> cards = Deck() >>> len(deck.deck) 52 >>>
Python 3 Class Problem
import numpy as np
class Deck(): def __init__(self): """Initializes the deck of cards >>> cards = Deck() >>> len(deck.deck) 52 >>> cards = Deck() >>> len(deck.dealt_cards) 0 >>> cards = Deck() >>> print(cards) In Deck: A of Clubs 2 of Clubs 3 of Clubs 4 of Clubs 5 of Clubs 6 of Clubs 7 of Clubs 8 of Clubs 9 of Clubs 10 of Clubs J of Clubs Q of Clubs K of Clubs A of Diamonds 2 of Diamonds 3 of Diamonds 4 of Diamonds 5 of Diamonds 6 of Diamonds 7 of Diamonds 8 of Diamonds 9 of Diamonds 10 of Diamonds J of Diamonds Q of Diamonds K of Diamonds A of Hearts 2 of Hearts 3 of Hearts 4 of Hearts 5 of Hearts 6 of Hearts 7 of Hearts 8 of Hearts 9 of Hearts 10 of Hearts J of Hearts Q of Hearts K of Hearts A of Spades 2 of Spades 3 of Spades 4 of Spades 5 of Spades 6 of Spades 7 of Spades 8 of Spades 9 of Spades 10 of Spades J of Spades Q of Spades K of Spades Dealt Out: """ ### your code here return
def shuffle(self): """ This method shuffles the deck using np.random.choice >>> deck = Deck() >>> np.random.seed(5) >>> deck.shuffle() >>> print(deck.cards[:5]) [9 of Hearts, 4 of Hearts, 7 of Spades, 7 of Diamonds, 6 of Hearts] >>> deck = Deck() >>> hand = deck.deal_cards(5) >>> np.random.seed(5) >>> deck.shuffle() >>> deck.cards[:5] [A of Spades, 9 of Hearts, Q of Spades, Q of Diamonds, J of Hearts] """ ### your code here return
def deal_cards(self,n): """ This method deals out n cards and sends them all to the dealt cards list. It also returns the list of the cards. >>> cards = Deck() >>> deck.deal_cards(5) [A of Clubs, 2 of Clubs, 3 of Clubs, 4 of Clubs, 5 of Clubs] >>> cards = Deck() >>> hand = cards.deal_cards(5) >>> cards.deal_cards(5) [6 of Clubs, 7 of Clubs, 8 of Clubs, 9 of Clubs, 10 of Clubs] >>> cards = Deck() >>> hand = deck.deal_cards(5) >>> print(cards) In Deck: 6 of Clubs 7 of Clubs 8 of Clubs 9 of Clubs 10 of Clubs J of Clubs Q of Clubs K of Clubs A of Diamonds 2 of Diamonds 3 of Diamonds 4 of Diamonds 5 of Diamonds 6 of Diamonds 7 of Diamonds 8 of Diamonds 9 of Diamonds 10 of Diamonds J of Diamonds Q of Diamonds K of Diamonds A of Hearts 2 of Hearts 3 of Hearts 4 of Hearts 5 of Hearts 6 of Hearts 7 of Hearts 8 of Hearts 9 of Hearts 10 of Hearts J of Hearts Q of Hearts K of Hearts A of Spades 2 of Spades 3 of Spades 4 of Spades 5 of Spades 6 of Spades 7 of Spades 8 of Spades 9 of Spades 10 of Spades J of Spades Q of Spades K of Spades Dealt Out: A of Clubs 2 of Clubs 3 of Clubs 4 of Clubs 5 of Clubs """ ### your code here
def __repr__(self): ### your code here return def __str__(self): ### your code here return
class Card(): ''' This class represents a single card Also compares two cards
>>> c1 = Card(4, "Clubs") >>> print(c1) 4 of Clubs
>>> c2 = Card(3, "Hearts") >>> print(c2) 3 of Hearts
>>> c1
>>> c1 > c2 False
>>> c1 == c2 False '''
def __init__(self, rank, suit): ### your code here return
def __repr__(self): ### your code here return
def __str__(self): ### your code here return def __eq__(self,other): ### your code here return
def __ne__(self,other): ### your code here return
def __lt__(self,other): ### your code here return
def __gt__(self,other): ### your code here return
def __le__(self,other): ### your code here return
def __ge__(self,other): ### your code here return
For this part, you will write a program that acts as a deck of cards A regular deck contains 52 cards . There are 4 suits: Hearts, Diamonds, Spades and Clubs . There are 13 ranks: Ace, King, Queen, Jack, 10, 9, 8, 7,6, 5, 4, 3, 2. You will need two create two classes, Card and Deck Class Ca The purpose of this class is to represent a single card. Also you should be able to compare two cards using symbols There are multiple ways to compare the cards, we will use this order: . Suits: Clubs (lowest), followed by Diamonds, Hearts, and Spades (highest) Ranks: Ace, 2, 3, 4, 5, 6,7, 8, 9, 10, Jack, Queen, King (from smallest to highest) For example: King of Clubs is smaller (>>cl-Card (4, "Clubs") >>> print (c1) 4 of Clubs >>> c2 = Card (3, "Hearts") >>> print (c2) 3 of Hearts >>c1 >>c1 c2 False False For this part, you will write a program that acts as a deck of cards A regular deck contains 52 cards . There are 4 suits: Hearts, Diamonds, Spades and Clubs . There are 13 ranks: Ace, King, Queen, Jack, 10, 9, 8, 7,6, 5, 4, 3, 2. You will need two create two classes, Card and Deck Class Ca The purpose of this class is to represent a single card. Also you should be able to compare two cards using symbols There are multiple ways to compare the cards, we will use this order: . Suits: Clubs (lowest), followed by Diamonds, Hearts, and Spades (highest) Ranks: Ace, 2, 3, 4, 5, 6,7, 8, 9, 10, Jack, Queen, King (from smallest to highest) For example: King of Clubs is smaller (>>cl-Card (4, "Clubs") >>> print (c1) 4 of Clubs >>> c2 = Card (3, "Hearts") >>> print (c2) 3 of Hearts >>c1 >>c1 c2 False FalseStep by Step Solution
There are 3 Steps involved in it
Step: 1
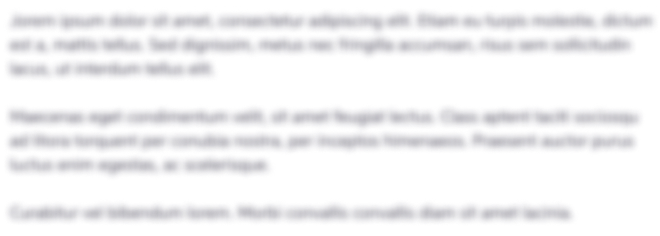
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started