Question
Python 3.7 Problem - ErrorHandling.py Create a class called BankAccount with the following attributes: Variables Methods balance transaction_history get_balance set_balance (balance) get_transaction_history set_transaction_history (transaction_history) get_avg_transaction
Python 3.7 Problem - ErrorHandling.py
Create a class called BankAccount with the following attributes:
Variables | Methods |
balance | get_balance set_balance (balance) get_transaction_history set_transaction_history (transaction_history) get_avg_transaction deposit (amount) withdraw (amount) |
The __init__ method should take an initial balance and default to 0 if nothing is entered transaction_history should be initialized as a blank list
Using whichever method you prefer (property/decorator) setup the get_balance, set_balance, get_transaction_history, and set_transaction_history methods as getters and setters for their respective variables
set_balance should assert that whatever balance is being set is greater than -1
set_transaction_history should set data to the transaction_history variable
deposit and withdraw should assert that whatever amount thats given is greater than 0
All three should ensure that a number is passed, and if not then raise a TypeError along with a message
withdraw should assert that whatever amount is being withdrawn is not greater than the balance
The transaction_history variable should be a list that records every transaction that is made (IE, when an amount is deposited, append the deposited amount, when an amount is withdrawn, append the negative withdrawn amount)
get_avg_transaction should first assert that the transaction_history is not empty. From there it should cycle through the history, adding up all of the numbers, and then find and return the average.
Create a second .py file called main and use the following code to check your program.
from ErrorHandling import BankAccount if __name__ == '__main__': # Create the bank account print("Creating the bank account:") try: bank_account = BankAccount(-100) except AssertionError: print("Error: Not greater than 0") except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") try: bank_account = BankAccount("100") except TypeError as e: print(e) except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") bank_account = BankAccount(100) # Attempt to get average transactions print(" Getting the average transactions (first time)") try: print(bank_account.get_avg_transaction()) except AssertionError: print("Error: No transactions have been made") except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") # Deposit money print(" Depositing money") try: bank_account.deposit(-200) except AssertionError: print("Error: Not greater than 0") except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") try: bank_account.deposit("200") except TypeError as e: print(e) except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") bank_account.deposit(200) # Withdraw money print(" Withdrawing money") try: bank_account.withdraw(-100) except AssertionError: print("Error: Not greater than 0") except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") try: bank_account.withdraw("100") except TypeError as e: print(e) except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS") bank_account.withdraw(100) # Get average transactions print(" Getting the average transactions (second time)") try: print(bank_account.get_avg_transaction()) except AssertionError: print("Error: No transactions have been made") except: print("YOU HAVE DONE SOMETHING WRONG; YOU SHOULD NOT BE SEEING THIS")
Your output should look like the following:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
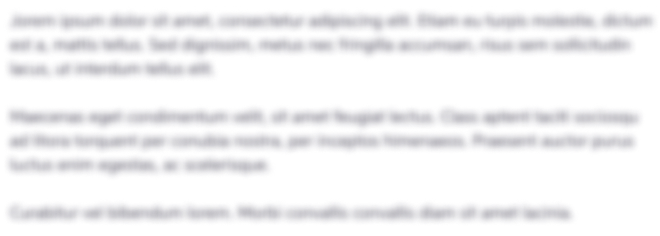
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started