Question
PYTHON ASSIGNMENT To make this project easier, you can utilize the Fraction module which will have a much higher resolution then using floating point types.
PYTHON ASSIGNMENT
To make this project easier, you can utilize the Fraction module which will have a much higher resolution then using floating point types. So, use the following import command:
from Fraction import *
Additionally, we will use the Decimal data type which has more precision than floats:
from decimal import *
Next, we will need some module-level variables for calculations:
Pi out to 50 digits:
pi50 = Decimal("3.14159265358979323846264338327950288419716939937510")
Number of iterations to perform:
iterations = 1000000
Create an iterator class to calculate the value of pi using the Leibniz series:
class LeibnizPiIterator:
- __init__() signature:
def __init__(self):
- For this method, simply use a pass command. All of the setup will occur in __iter__.
- __iter__() signature:
def __iter__(self):
- This method initializes the values we will need for the iterator
- Create an instance variable called self.fraction and assign to it a Fraction object with a numerator of 0 and denominator of 1. This will represent the running total for the series.
- Create an instance variable called self.n and assign 1 to it. This will represent the denominator to be used in the next iteration (see documentation above).
- Create an instance variable called self.add_next and assign to it True. This is a Boolean value indicating if the next iteration will be an add or subtract.
- Return self
- __next__() signature:
def __next__(self):
- This method is where the work is done for each iteration.
- If self.add_next is True then the next value is to be added to self.fraction. Otherwise, subtract it.
- The next value is a new Fraction object with the value 4 / self.n
- After updating self.fraction do the following:
- Change the value of self.add_next to its opposite.
- Add 2 to self.n
- Return self.fraction.value
Test the Iterator:
- Set iterations to 100,000 (dont use the thousands separator)
- Create a new LeibnizPiIterator object and loop through it iterations times.
- You can implement using a for loop or while loop.
- Report the result of the final value to the terminal
- Then calculate the difference between the final value from the iterator and pi50 and report that value to the terminal as well.
- Change the iterations variable to 10,000,000 and re-run the test (it will take much more time, maybe get a coffee!)
Sample Output:
pi after 100000 iterations: 3.14158265358985315979006807645440766789826367641700
Difference: 0.00000999999994007867257530682509500000000000000000
pi after 10000000 iterations: 3.14159255358913974898979182536369204750639121844740 Difference: 0.00000010000065348947285155791581080000000000000000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
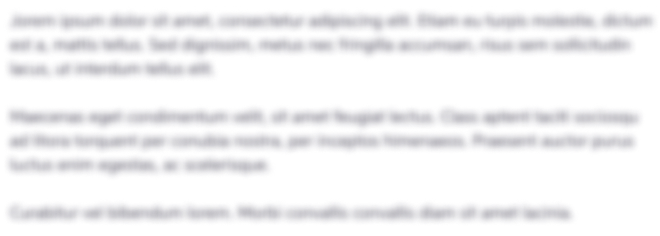
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started