Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Python code for part d Use stem import copy as CP def FirstNonZero_Index(R): #finds pivot for a row vector (R) m=-1 for n in range(len(R)):
Python code for part d
Use stem
import copy as CP def FirstNonZero_Index(R): #finds pivot for a row vector (R) m=-1 for n in range(len(R)): if R[n] != 0.0: m=n break return m #if the whole row is zeros, returns -1 #the elementary operations for Gaussian elimination are # 1. Swap the positions of two rows # 2. Multiply a row by a non-zero scalar # 3. Add to one row, a scalar multiple of another row def SwapRows(A, r1, r2): #if I do this, it is known as a partial pivot ''' One of the elementary row operations in Gaussian elimination. :param A: A matrix :param r1: index of row 1 :param r2: index of row 2 :return: ''' rmax=max(r1,r2) #the larger index rmin=min(r1,r2) #the smaller index RMax=A[rmax] #temporarily store the row vector at rmax in a variable RMin=A.pop(rmin) #pop function removes this row from the matrix and shifts all larger indices by -1 A.insert(rmin,RMax) #insert the row RMax at the location rmin. All higher index rows increase index by +1 A[rmax]=RMin #now, replace row rmax with RMin return A #done def MultRow(R,s=1): ''' #used to multiply a row vector by a scalar value :param R: the row vector :param s: the scalar with default value = 1 :return: a new row vector multiplied by the scalar (s*R) ''' # a short way to build the list, element by element is called a list comprehension. # https://docs.python.org/3/tutorial/datastructures.html#list-comprehensions return [round(i*s,4) for i in R] def AddRows(R1, R2, s=1.0): ''' Adds a scalar multiple of row vector R2 to row vector R1. :param R1: a row vector :param R2: another row vector :param s: a scalar :return: a new row vector (R1+s*R2) ''' #use a list comprehension to build the return list #https://docs.python.org/3/tutorial/datastructures.html#list-comprehensions #zip function: Make an iterator that aggregates elements from each of the iterables. #https://docs.python.org/3.3/library/functions.html#zip return [round(i+s*j,4) for i, j in zip(R1, R2)] #the Echelon form of a matrix is when I produce an upper triangular matrix by Gaussian elimination def EchelonForm(A): ''' I'm expecting a Matrix of m rows by n columns. This function performs row operations (Gauss elimination) to produce echelon form matrix. :param Matrix: the matrix :return: the echelon form of the matrix ''' m=len(A) #number of rows of A n=len(A[0]) #number of columns of A Ech = CP.deepcopy(A) #make a deep copy of A so that I don't actually change A #order the rows by first non-zero in each column for i in range(m): #iterate through all rows for r in range(i,m): #iterate through all rows below row i p=FirstNonZero_Index(Ech[r]) #find column index in row r that is non-zero if p==i: #found a row with non-zero in ith position Ech = SwapRows(Ech, r,i) #move this row to the ith row break #stops iterating through rows below i since I found a suitable row to put in position i if(Ech[i][i] != 0.0): #if I found a non-zero value for the [i][i] pivot for r in range(i+1,m): #now add multiples of row i to rows i+1 to m in order to make column i values zero below row i p=FirstNonZero_Index(Ech[r]) if p==i: #found row p has a nonzero element in column i Row=Ech[r] s=-Ech[r][p]/Ech[i][i] Ech[r] = AddRows(Row,Ech[i],s) return Ech #the reduced echelon form of a matrix is when the numbers along the diagonal are all 1's and rows above all other #numbers int he column are zero def ReducedEchelonForm(A): REF=EchelonForm(A) #first reduce to echelon form for i in range(len(A)-1,-1,-1): #iterate from last row to row 1 R=REF[i] j=FirstNonZero_Index(R) #find the first non-zero column in r R=MultRow(R,1.0/R[j]) #make jth value equal to 1.0 REF[i]=R for ii in range(i-1,-1,-1): #remember, end index in range is non-inclusive RR=REF[ii] if(RR[j]!=0): RR = AddRows(RR,R,-RR[j]) REF[ii]=RR return REF def GaussElim(Aaug): RREF=ReducedEchelonForm(Aaug) x=[] for r in range(len(RREF)): c=len(RREF[0])-1 a=RREF[r][c]/RREF[r][r] x.append(a) return x #produce and identity matrix of the same size as A def IDMatrix(A): pass #produce an augmented matrix from A and B def AugmentMatrix(A,B): pass #remove the jth column from matrix A def RemoveColumn(A,j): pass #uses the matrix A augmented with the identity matrix and Gaussian elimination def Inverse(A): pass #use this to multiply matrices of correct dimensions def MatrixMultiply(A,B): pass def main(): MyA = [[3, 1, -1], [1, 4, 1], [2, 1, 2]] MyB=[2, 12, 10] AnotherA = [[1, -10, 2, 4], [3, 1, 4, 12], [9, 2, 3, 4], [-1, 2, 7, 3]] AnotherB=[2, 12, 21, 37] InvMyA = Inverse(MyA) InvAnotherA = Inverse(AnotherA) main()c) Write a function defined as: def GaussSeidel (Aaug, x, Niter = 15): Purpose: use the Gauss-Seidel method to estimate the solution to a set of N linear equations expressed in matrix form as Ax = b. Both A and b are contained in the function argument - Aaug. Aaug an augmented matrix containing [A | bl having N rows and N+1 columns, where N is the number of equations in the set. X: a vector (array) contain the values of the initial guess Niter: the number of iterations (new x vectors) to compute return value the final new x vector. N Write and call a main function that uses your GaussSeidel function to estimate and print the solutions to the following sets of linear equations: 3 1 -1 x 2 1 4 1 X2 1 10 2 2 x 1 -10 2 X1 2 3 1 4 12 X2 12 9 2 3 4 X3 21 -1 2 7 3 X4 37 d) Write a function defined as: def Inverse (A): Purpose of Inverse (A): use the Gauss-Jordan Elimination to find the inverse of the A matrix. A is a square matrix of n-rows by n-cols. return value: A-is the inverse of matrix A Write and call a main() function that uses your Inverse function to calculate and print the solution A-1 matrix for the A matrices of partc). Also, verify that A-is in fact the inverse of A by performing the operation A-1A and obtaining the proper identity matrix. Use the inverse matricies to solve the equations in part c) and output the result to the console. Output statements should be informative and nicely formatted. *note: you may use my code hw2d-stem.py as a starting point for part d. c) Write a function defined as: def GaussSeidel (Aaug, x, Niter = 15): Purpose: use the Gauss-Seidel method to estimate the solution to a set of N linear equations expressed in matrix form as Ax = b. Both A and b are contained in the function argument - Aaug. Aaug an augmented matrix containing [A | bl having N rows and N+1 columns, where N is the number of equations in the set. X: a vector (array) contain the values of the initial guess Niter: the number of iterations (new x vectors) to compute return value the final new x vector. N Write and call a main function that uses your GaussSeidel function to estimate and print the solutions to the following sets of linear equations: 3 1 -1 x 2 1 4 1 X2 1 10 2 2 x 1 -10 2 X1 2 3 1 4 12 X2 12 9 2 3 4 X3 21 -1 2 7 3 X4 37 d) Write a function defined as: def Inverse (A): Purpose of Inverse (A): use the Gauss-Jordan Elimination to find the inverse of the A matrix. A is a square matrix of n-rows by n-cols. return value: A-is the inverse of matrix A Write and call a main() function that uses your Inverse function to calculate and print the solution A-1 matrix for the A matrices of partc). Also, verify that A-is in fact the inverse of A by performing the operation A-1A and obtaining the proper identity matrix. Use the inverse matricies to solve the equations in part c) and output the result to the console. Output statements should be informative and nicely formatted. *note: you may use my code hw2d-stem.py as a starting point for part d
Step by Step Solution
There are 3 Steps involved in it
Step: 1
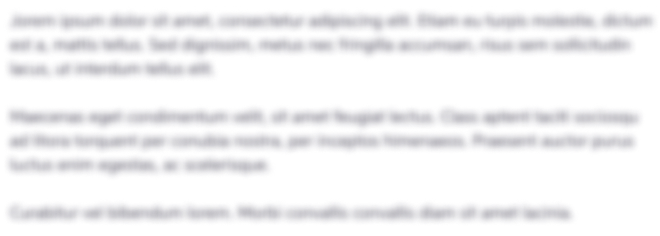
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started