Question
PYTHON CODE (PLEASE MAKE IT SIMPLE AND WITH FULL COMMENTS AT EACH STEP) Define a Python Class named Steam. The attributes and methods are defined
PYTHON CODE
(PLEASE MAKE IT SIMPLE AND WITH FULL COMMENTS AT EACH STEP)
Define a Python Class named Steam. The attributes and methods are defined below. The behavior of this class will be demonstrated with an example main program that uses the class. The class named Steam has 8 attributes, an three methods: __init__, calc, and print, as defined below:
import numpy as np from scipy.interpolate import griddata
class Steam: def __init__(self, pressure, T=None, h=None, s=None, x=None, v=None, name=None): self.p = pressure # pressure - kPa self.T = T # Temperature - degrees C self.x = x # quality (a value between 0 and 1) self.v = v # specific volume - m^3/kg self.h = h # enthalpy - kJ/kg self.s = s # entropy - kJ/(kg K) self.name = name # a useful identifier self.region = None # 'superheated' or 'saturated' if T==None and x==None and v==None and h==None and s==None: return self.calc() def calc(self): # calculate the missing steam properties using griddata() and the steam tables given earlier.
IMPORTANT:
a)look carefully at the PRESSURE units in the two tables. They are DIFFERENT.
b) Beware of the GAP IN THE DATA problem!
def print(self): # print the available (not None) Steam values
Note: calc(self) should raise errors if asked to do things in cannot handle such as:
raise ValueError('Error - this function cannot operate in the sub-cooled region') raise ValueError('Not enough properties specified')
def main():
inlet=Steam(7350, name='Turbine Inlet')
inlet.x =0.9 # 90 percent quality - accessed
inlet.calc() inlet.print() h1 = inlet.h; s1 = inlet.s
print(h1,s1,' ')
outlet=Steam(100, s=inlet.s, name='Turbine Exit')
#notice - s=inlet.s
outlet.print()
another=Steam(8575, h=2050, name='State 3')
another.print()
yetanother=Steam(8900, h=41250, name='State 4')
yetanother.print()
#uncommenting the next two lines will cause a ValueError error
#final1 = Steam(7000, T=250, name='State 5')
#final1.print()
if __name__ == "__main__":
main()
sat_water_table
Temperature, degC Pressure, bar hf, kJ/kg hg, kJ/kg sf, kJ/(kg K) sg, kJ/(kg K) vf, m^3/kg vg, m^3/kg 0.01 0.00611657 0.000611783 2500.9109946395 0 9.1554914737 0.0010002063 205.9974594854 4 0.0081354938 16.8127172951 2508.237464527 0.0611009773 9.0505562347 0.0010000743 157.1213322514 5 0.0087257486 21.019355831 2510.0717168774 0.0762516518 9.0248586894 0.0010000825 147.0168645061 6 0.0093535306 25.2236629605 2511.9051298992 0.091339574 8.999396572 0.0010001061 137.6381802847 8 0.0107298775 33.6260165856 2515.5693278065 0.1213315653 8.9491672418 0.0010001979 120.8344299395 10 0.0122818387 42.0210886485 2519.2298389404 0.1510850863 8.8998459206 0.0010003464 106.3086968846 11 0.0131294913 46.2162428415 2521.0586437675 0.1658747009 8.8755189732 0.0010004409 99.7926628861 12 0.0140282237 50.4099715858 2522.8864451461 0.1806073323 8.8514109625 0.0010005485 93.7242909414 13 0.0149806377 54.602383983 2524.7132158716 0.1952837607 8.8275192845 0.0010006688 88.0697806593 14 0.0159894406 58.7935801452 2526.5389287185 0.2099047263 8.8038413744 0.0010008015 82.7980991212 15 0.0170574487 62.9836520805 2528.3635564137 0.2244709337 8.7803747058 0.0010009463 77.8807375687 16 0.0181875904 67.1726844956 2530.1870716086 0.2389830549 8.7571167899 0.0010011029 73.2914908852 17 0.0193829088 71.3607555214 2532.0094468513 0.2534417318 8.7340651746 0.0010012711 69.0062576126 18 0.0206465653 75.5479373698 2533.8306545571 0.2678475797 8.7112174441 0.0010014506 65.0028584761 19 0.0219818425 79.7342969275 2535.6506669793 0.282201189 8.6885712175 0.0010016412 61.2608716021 20 0.0233921477 83.919896293 2537.4694561791 0.2965031278 8.6661241488 0.0010018426 57.761482803 21 0.0248810157 88.1047932619 2539.2869939962 0.3107539435 8.6438739255 0.0010020546 54.4873494688 22 0.0264521125 92.2890417649 2541.1032520186 0.3249541649 8.6218182683 0.0010022771 51.4224767527 23 0.0281092382 96.4726922639 2542.918201553 0.3391043037 8.5999549303 0.0010025098 48.5521048757 24 0.0298563307 100.6557921095 2544.7318135952 0.3532048559 8.5782816961 0.0010027526 45.8626064902 25 0.0316974685 104.8383858627 2546.5440588015 0.3672563028 8.5567963813 0.0010030052 43.3413931491 26 0.0336368748 109.0205155861 2548.35490746 0.3812591128 8.5354968318 0.0010032675 40.976830026 27 0.0356789202 113.2022211055 2550.1643294633 0.3952137419 8.5143809231 0.0010035393 38.7581581114 28 0.0378281263 117.3835402458 2551.9722942811 0.4091206349 8.4934465597 0.0010038205 36.6754231912 29 0.0400891693 121.5645090436 2553.7787709345 0.4229802263 8.4726916745 0.001004111 34.7194109793 30 0.0424668834 125.7451619381 2555.5837279712 0.4367929406 8.452114228 0.0010044105 32.8815878383 31 0.0449662639 129.9255319425 2557.387133441 0.4505591938 8.4317122079 0.001004719 31.1540465764 32 0.0475924712 134.1056507988 2559.1889548734 0.4642793933 8.4114836286 0.0010050363 29.5294568599 33 0.0503508338 138.285549116 2560.9891592559 0.4779539388 8.3914265303 0.0010053623 28.0010198235 34 0.053246852 142.465256494 2562.7877130134 0.4915832228 8.3715389789 0.0010056968 26.5624265007 35 0.0562862014 146.6448016353 2564.5845819893 0.5051676309 8.3518190649 0.0010060399 25.2078197317 36 0.0594747366 150.8242124447 2566.3797314275 0.5187075423 8.3322649036 0.0010063913 23.9317592425 38 0.0663236963 159.1827392211 2569.9647295706 0.5456553625 8.293646418 0.0010071187 21.5954108675 40 0.0738442749 167.5410472587 2573.542416808 0.5724296025 8.2556689123 0.0010078784 19.5170436718 45 0.0959438884 188.437174006 2582.4526465914 0.6386242256 8.1634367692 0.001009914 15.2534355388 50 0.1235127043 209.3362003949 2591.3102638166 0.7037939047 8.0749091813 0.0010121368 12.0278641466 55 0.1576141353 230.2410056178 2600.1098173886 0.7679786179 7.9898850157 0.0010145386 9.5649212154 60 0.1994580192 251.1543931381 2608.845404702 0.8312162521 7.9081744097 0.0010171128 7.6676563233 65 0.250410979 272.0791243694 2617.5106723753 0.8935428243 7.8295978595 0.0010198541 6.1938277991 70 0.312006357 293.0179366828 2626.0988205609 0.95499264 7.7539854176 0.001022758 5.0397327017 75 0.3859536269 313.9735540674 2634.6026071001 1.01559842 7.681175975 0.0010258211 4.1290813065 80 0.4741471993 334.9486951434 2643.0143499475 1.0753914097 7.6110166122 0.0010290408 3.4052654064 85 0.5786745487 355.9460810347 2651.3259287174 1.1344014808 7.5433620113 0.001032415 2.825933479 90 0.7018236074 376.9684443135 2659.5287883637 1.1926572289 7.478073931 0.0010359426 2.3591493948 95 0.846089384 398.0185394747 2667.6139493866 1.2501860688 7.4150207449 0.0010396228 1.9806484371 100 1.0141797792 419.0991549977 2675.5720292208 1.3070143278 7.354077051 0.0010434555 1.6718606011 110 1.4337596724 461.3633531387 2691.0676333138 1.4186695174 7.238045797 0.0010515803 1.209389605 120 1.9866539974 503.784567107 2705.934247417 1.5278150478 7.1290908569 0.0010603266 0.8913039522 130 2.7025960656 546.3878367749 2720.0878257369 1.6346312703 7.0264078298 0.0010697113 0.6680844514 140 3.6150096198 589.2002596436 2733.4439437081 1.739289066 6.9292723061 0.0010797596 0.5085191744 150 4.7610138108 632.2515601107 2745.9191425853 1.8419520428 6.8370333416 0.0010905047 0.3925024138 160 6.1813919672 675.5746798636 2757.4305309693 1.9427785229 6.7491038453 0.0011019885 0.3068184475 170 7.9205318369 719.2063979309 2767.8936550359 2.041923368 6.6649484232 0.0011142624 0.2426157955 180 10.0263456881 763.1879981829 2777.2194106819 2.1395396975 6.5840707717 0.001127389 0.1938616052 190 12.5501792086 807.5660111798 2785.3110035082 2.23578057 6.5060028407 0.0011414429 0.1563767894 200 15.5467186827 852.3930680834 2792.0615640123 2.3308007061 6.430296772 0.0011565139 0.1272223214 210 19.0739066433 897.7289197645 2797.3523490814 2.4247583526 6.3565190579 0.0011727089 0.1043019481 220 23.1928772773 943.6416987006 2801.051000399 2.5178174199 6.2842455211 0.0011901563 0.0861007204 230 27.9679245577 990.2095414473 2803.0092956133 2.6101500866 6.2130558601 0.0012090108 0.0715102348 240 33.4665187151 1037.5227548257 2803.0599721382 2.7019401597 6.1425269673 0.0012294605 0.0597101233 250 39.7593907084 1085.6868126801 2801.0120704016 2.7933876369 6.0722241011 0.0012517369 0.050086565 260 46.9207105436 1134.8266316172 2796.6436363951 2.8847151531 6.0016880237 0.001276129 0.0421754868 270 55.0283947409 1185.0928237803 2789.6898612305 2.9761773555 5.9304151362 0.0013030052 0.0356224479 280 64.1645928168 1236.6710007035 2779.8244623369 3.0680747776 5.8578276097 0.0013328453 0.0301539651 290 74.4164254362 1289.7957210967 2766.6326458614 3.1607744802 5.7832318823 0.0013662912 0.0255568267 300 85.8770832956 1344.7713390197 2749.573742544 3.2547405505 5.7057636168 0.001404223 0.0216630647 320 112.8385588655 1462.0510091193 2700.6676873413 3.4491160973 5.5373194499 0.0014990631 0.015475927 340 146.0018105681 1594.4465698708 2622.0667476473 3.6599493787 5.3359115648 0.0016375137 0.0107838325 360 186.6637110275 1761.4917585529 2481 3.916357556 5.0526 0.0018945141 0.006945 374.14 220.9 2099.3 2099.3 4.4298 4.4298 0.003155 0.003155
superheated_water_table
temp h s p kpa 36.16 2567.40 8.33 6.00 80.00 2650.10 8.58 6.00 120.00 2726.00 8.78 6.00 160.00 2802.50 8.97 6.00 200.00 2879.70 9.14 6.00 240.00 2957.80 9.30 6.00 280.00 3036.80 9.45 6.00 320.00 3116.70 9.59 6.00 360.00 3197.70 9.72 6.00 400.00 3279.60 9.84 6.00 440.00 3362.60 9.96 6.00 500.00 3489.10 10.13 6.00 89.95 2660.00 7.48 70.00 100.00 2680.00 7.53 70.00 120.00 2719.60 7.64 70.00 160.00 2798.20 7.83 70.00 200.00 2876.70 8.00 70.00 240.00 2955.50 8.16 70.00 280.00 3035.00 8.32 70.00 320.00 3115.30 8.45 70.00 360.00 3196.50 8.58 70.00 400.00 3278.60 8.71 70.00 440.00 3361.80 8.83 70.00 500.00 3488.50 9.00 70.00 111.37 2693.60 7.22 150.00 120.00 2711.40 7.27 150.00 160.00 2792.80 7.47 150.00 200.00 2872.90 7.64 150.00 240.00 2952.70 7.81 150.00 280.00 3032.80 7.96 150.00 320.00 3113.50 8.10 150.00 360.00 3195.00 8.23 150.00 400.00 3277.40 8.36 150.00 440.00 3360.70 8.48 150.00 500.00 3487.60 8.65 150.00 600.00 3704.30 8.91 150.00 151.86 2748.70 6.82 500.00 180.00 2812.00 6.97 500.00 200.00 2855.40 7.06 500.00 240.00 2939.90 7.23 500.00 280.00 3022.90 7.39 500.00 320.00 3105.60 7.53 500.00 360.00 3188.40 7.67 500.00 400.00 3271.90 7.79 500.00 440.00 3356.00 7.92 500.00 500.00 3483.90 8.09 500.00 600.00 3701.70 8.35 500.00 700.00 3925.90 8.60 500.00 179.91 2778.10 6.59 1000.00 200.00 2827.90 6.69 1000.00 240.00 2920.40 6.88 1000.00 280.00 3008.20 7.05 1000.00 320.00 3093.90 7.20 1000.00 360.00 3178.90 7.33 1000.00 400.00 3263.90 7.47 1000.00 440.00 3349.30 7.59 1000.00 500.00 3478.50 7.76 1000.00 540.00 3565.60 7.87 1000.00 600.00 3697.90 8.03 1000.00 640.00 3787.20 8.13 1000.00 212.42 2799.50 6.34 2000.00 240.00 2876.50 6.50 2000.00 280.00 2976.40 6.68 2000.00 320.00 3069.50 6.85 2000.00 360.00 3159.30 6.99 2000.00 400.00 3247.60 7.13 2000.00 440.00 3335.50 7.25 2000.00 500.00 3467.60 7.43 2000.00 540.00 3556.10 7.54 2000.00 600.00 3690.10 7.70 2000.00 640.00 3780.40 7.80 2000.00 700.00 3917.40 7.95 2000.00 250.40 2801.40 6.07 4000.00 280.00 2901.80 6.26 4000.00 320.00 3015.40 6.46 4000.00 360.00 3117.20 6.62 4000.00 400.00 3213.60 6.77 4000.00 440.00 3307.10 6.90 4000.00 500.00 3445.30 7.09 4000.00 540.00 3536.90 7.21 4000.00 600.00 3674.40 7.37 4000.00 640.00 3766.60 7.47 4000.00 700.00 3905.90 7.62 4000.00 740.00 3999.60 7.71 4000.00 295.06 2758.00 5.74 8000.00 320.00 2877.20 5.95 8000.00 360.00 3019.80 6.18 8000.00 400.00 3138.30 6.36 8000.00 440.00 3246.10 6.52 8000.00 480.00 3348.40 6.66 8000.00 520.00 3447.70 6.79 8000.00 560.00 3545.30 6.91 8000.00 600.00 3642.00 7.02 8000.00 640.00 3738.30 7.13 8000.00 700.00 3882.40 7.28 8000.00 740.00 3978.70 7.38 8000.00 324.75 2684.9 5.4924 12000 360 2895.7 5.8361 12000 400 3051.3 6.0747 12000 440 3178.7 6.2586 12000 480 3293.5 6.4154 12000 520 3401.8 6.5555 12000 560 3506.2 6.684 12000 600 3608.3 6.8037 12000 640 3709 6.9164 12000 700 3858.4 7.0749 12000 740 3957.4 7.1746 12000 347.44 2580.6 5.2455 16000 360 2715.8 5.4614 16000 400 2947.6 5.8175 16000 440 3103.7 6.0429 16000 480 3234.4 6.2215 16000 520 3353.3 6.3752 16000 560 3465.4 6.5132 16000 600 3573.5 6.6399 16000 640 3678.9 6.758 16000 700 3833.9 6.9224 16000 740 3935.9 7.0251 16000 365.81 2409.7 4.9269 20000 400 2818.1 5.554 20000 440 3019.4 5.845 20000 480 3170.8 6.0518 20000 520 3302.2 6.2218 20000 560 3423 6.3705 20000 600 3537.6 6.5048 20000 640 3648.1 6.6286 20000 700 3809 6.7993 20000 740 3914.1 6.9052 20000 800 4069.7 7.0544 20000 400 2330.7 4.7494 28000 440 2812.6 5.4494 28000 480 3028.5 5.7446 28000 520 3192.3 5.9566 28000 560 3333.7 6.1307 28000 600 3463 6.2823 28000 640 3584.8 6.4187 28000 700 3758.4 6.6029 28000 740 3870 6.7153 28000 800 4033.4 6.872 28000 900 4298.8 7.1084 28000 72.69 2631.40 7.72 35.00 80.00 2645.60 7.76 35.00 120.00 2723.10 7.96 35.00 160.00 2800.60 8.15 35.00 200.00 2878.40 8.32 35.00 240.00 2956.80 8.48 35.00 280.00 3036.00 8.63 35.00 320.00 3116.10 8.77 35.00 360.00 3197.10 8.90 35.00 400.00 3279.20 9.03 35.00 440.00 3362.20 9.15 35.00 500.00 3488.80 9.32 35.00 99.63 2675.50 7.36 100.00 100.00 2676.20 7.36 100.00 120.00 2716.60 7.47 100.00 160.00 2796.20 7.66 100.00 200.00 2875.30 7.83 100.00 240.00 2954.50 7.99 100.00 280.00 3034.20 8.14 100.00 320.00 3114.60 8.28 100.00 360.00 3195.90 8.42 100.00 400.00 3278.20 8.54 100.00 440.00 3361.40 8.66 100.00 500.00 3488.10 8.83 100.00 133.55 2725.30 6.99 300.00 160.00 2782.30 7.13 300.00 200.00 2865.50 7.31 300.00 240.00 2947.30 7.48 300.00 280.00 3028.60 7.63 300.00 320.00 3110.10 7.77 300.00 360.00 3192.20 7.91 300.00 400.00 3275.00 8.03 300.00 440.00 3358.70 8.15 300.00 500.00 3486.00 8.33 300.00 600.00 3703.20 8.59 300.00 164.97 2763.50 6.71 700.00 180.00 2799.10 6.79 700.00 200.00 2844.80 6.89 700.00 240.00 2932.20 7.06 700.00 280.00 3017.10 7.22 700.00 320.00 3100.90 7.37 700.00 360.00 3184.70 7.51 700.00 400.00 3268.70 7.64 700.00 440.00 3353.30 7.76 700.00 500.00 3481.70 7.93 700.00 600.00 3700.20 8.20 700.00 700.00 3924.80 8.44 700.00 198.32 2792.20 6.44 1500.00 200.00 2796.80 6.45 1500.00 240.00 2899.30 6.66 1500.00 280.00 2992.70 6.84 1500.00 320.00 3081.90 6.99 1500.00 360.00 3169.20 7.14 1500.00 400.00 3255.80 7.27 1500.00 440.00 3342.50 7.39 1500.00 500.00 3473.10 7.57 1500.00 540.00 3560.90 7.68 1500.00 600.00 3694.00 7.84 1500.00 640.00 3783.80 7.94 1500.00 233.90 2804.20 6.19 3000.00 240.00 2824.30 6.23 3000.00 280.00 2941.30 6.45 3000.00 320.00 3043.40 6.62 3000.00 360.00 3138.70 6.78 3000.00 400.00 3230.90 6.92 3000.00 440.00 3321.50 7.05 3000.00 500.00 3456.50 7.23 3000.00 540.00 3546.60 7.35 3000.00 600.00 3682.30 7.51 3000.00 640.00 3773.50 7.61 3000.00 700.00 3911.70 7.76 3000.00 275.64 2784.30 5.89 6000.00 280.00 2804.20 5.93 6000.00 320.00 2952.60 6.18 6000.00 360.00 3071.10 6.38 6000.00 400.00 3177.20 6.54 6000.00 440.00 3277.30 6.69 6000.00 500.00 3422.20 6.88 6000.00 540.00 3517.00 7.00 6000.00 600.00 3658.40 7.17 6000.00 640.00 3752.60 7.27 6000.00 700.00 3894.10 7.42 6000.00 740.00 3989.20 7.52 6000.00 311.06 2724.70 5.61 10000.00 320.00 2781.30 5.71 10000.00 360.00 2962.10 6.01 10000.00 400.00 3096.50 6.21 10000.00 440.00 3213.20 6.38 10000.00 480.00 3321.40 6.53 10000.00 520.00 3425.10 6.66 10000.00 560.00 3526.00 6.79 10000.00 600.00 3625.30 6.90 10000.00 640.00 3723.70 7.01 10000.00 700.00 3870.50 7.17 10000.00 740.00 3968.10 7.27 10000.00 336.75 2637.6 5.3717 14000 360 2816.5 5.6602 14000 400 3001.9 5.9448 14000 440 3142.2 6.1474 14000 480 3264.5 6.3143 14000 520 3377.8 6.461 14000 560 3486 6.5941 14000 600 3591.1 6.7172 14000 640 3694.1 6.8326 14000 700 3846.2 6.9939 14000 740 3946.7 7.0952 14000 357.06 2509.1 5.1044 18000 360 2564.5 5.1922 18000 400 2887 5.6887 18000 440 3062.8 5.9428 18000 480 3203.2 6.1345 18000 520 3378 6.296 18000 560 3444.4 6.4392 18000 600 3555.6 6.5696 18000 640 3663.6 6.6905 18000 700 3821.5 6.858 18000 740 3925 6.9623 18000 400 2639.4 5.2393 24000 440 2923.4 5.6506 24000 480 3102.3 5.895 24000 520 3248.5 6.0842 24000 560 3379 6.2448 24000 600 3500.7 6.3875 24000 640 3616.7 6.5174 24000 700 3783.8 6.6947 24000 740 3892.1 6.8038 24000 800 4051.6 6.9567 24000 400 2055.9 4.3239 32000 440 2683 5.2327 32000 480 2949.2 5.5968 32000 520 3133.7 5.8357 32000 560 3287.2 6.0246 32000 600 3424.6 6.1858 32000 640 3552.5 6.329 32000 700 3732.8 6.5203 32000 740 3847.8 6.6361 32000 800 4015.1 6.7966 32000 900 4285.1 7.0372 32000
Step by Step Solution
There are 3 Steps involved in it
Step: 1
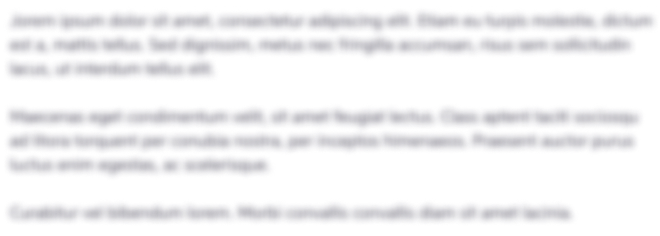
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started