Question
Python Code plz # Given Code class AD(dict): def __init__(self, *args, **kwargs): This initializer simply passes all arguments to dict, so that we can create
Python Code plz
# Given Code
class AD(dict):
def __init__(self, *args, **kwargs):
"""This initializer simply passes all arguments to dict, so that
we can create an AD with the same ease with which we can create
a dict. There is no need, indeed, to repeat the initializer,
but we leave it here in case we want to create attributes specific
to an AD later."""
super().__init__(*args, **kwargs)
def __add__(self, other):
return AD._binary_op(self, other, lambda x, y: x + y, 0)
def __sub__(self, other):
return AD._binary_op(self, other, lambda x, y: x - y, 0)
@staticmethod
def _binary_op(left, right, op, neutral):
r = AD()
l_keys = set(left.keys()) if isinstance(left, dict) else set()
r_keys = set(right.keys()) if isinstance(right, dict) else set()
for k in l_keys | r_keys:
# If the right (or left) element is a dictionary (or an AD),
# we get the elements from the dictionary; else we use the right
# or left value itself. This implements a sort of dictionary
# broadcasting.
l_val = left.get(k, neutral) if isinstance(left, dict) else left
r_val = right.get(k, neutral) if isinstance(right, dict) else right
r[k] = op(l_val, r_val)
return r
class Pantry(AD):
def __init__(self, ingredients):
"""We initialize the Pantry class by passing the
ingredients that were passed in to the initializer
for the superclass, AD"""
super().__init__(ingredients)
class Recipe:
def __init__(self, name, ingredients):
"""We initialize the Recipe class, which stores the
name of a recipe as well as the needed ingredients
in the form of an AD"""
self.name = name
self.ingredients = AD(ingredients)
def __repr__(self):
"""To have a better representation of the recipe"""
return f'{self.name}: {self.ingredients}'
def __hash__(self):
"""Allows us to use a Recipe object inside a set or as
a dictionary key. We assume that each recipe will have
a unique name, and therefore we can simply use the built-in
hash function on the name and return it as the hash id."""
return hash(self.name)
def __eq__(self,other):
"""For a recipe to equal another, their name and ingredients
must match"""
return self.name == other.name and dict(self.ingredients) == dict(other.ingredients)
def individually_feasible(self, recipes):
"""Returns a list of recipes that could be
individually made using the ingredients in the pantry.
Can be implemented in 9 lines of code."""
# create an empty output list
feasible_recipe = []
# loop over the recipes list
for recipe in recipes:
allPresent = True # set the flag to True at the beginning
# loop over the ingredients of the recipe
for key in recipe.ingredients:
# if ingredient is not in Pantry or quantity of ingredient in Pantry < quantity of ingredient required in Recipe
if key not in self or self[key] < recipe.ingredients[key]:
allPresent = False # then set allPresent to False and exit the loop
break
# if all ingredients are present in enough quantity in Pantry, insert the recipe in the output list
if allPresent:
feasible_recipe.append(recipe)
return feasible_recipe # return the list if recipes that could be made
raise NotImplementedError()
Pantry.individually_feasible = individually_feasible
def simul_feasible_nr(self, recipes):
"""Returns a list containing different combinations
of recipes that could be made simultaneously using
the ingredients available in the pantry."""
raise NotImplementedError
Pantry.simul_feasible_nr = simul_feasible_nr
p1 = Pantry({"egg":6, "beans":11, "rice":7, "onion": 1,
"mushrooms":1, "spinach":1, "cheese":1,
"soy sauce":1, "butter":1, "oil":2})
fried_rice = Recipe("Fried Rice", {"rice":4, "egg":2, "onion":1, "soy sauce":1, "oil":1})
rice_and_beans = Recipe("Rice and Beans", {"rice":4, "beans":4, "oil":1})
spinach_mushroom_scrambled_eggs = Recipe("Spinach-Mushroom Scrambled Eggs",
{"egg":4, "mushrooms":1, "spinach":0.2, "cheese":1, "butter":1})
watermelon = Recipe("Watermelon", {"watermelon":1})
p1_simul_feasible_nr = p1.simul_feasible_nr([fried_rice, rice_and_beans, spinach_mushroom_scrambled_eggs, watermelon])
for collection in p1_simul_feasible_nr:
print("Recipe(s) that can be made simultaneously:")
for recipe in collection:
print(" * {}".format(recipe.name))
print("")
Arithmetic Dictionaries In this assignment, we will be using the arithmetic dictionary class shown during lecture: class AD(dict): def _init_(self, *args, **kwargs): """This initializer simply passes all arguments to dict, so that we can create an AD with the same ease with which we can create a dict. There is no need, indeed, to repeat the initializer, but we leave it here in case we want to create attributes specific to an AD later.""" super().__init__(*args, **kwargs) def _add_(self, other): return AD._binary_op (self, other, lambda x, y: x + y, 0) def __sub_(self, other): return AD._binary_op (self, other, lambda x, y: xy, 0) @staticmethod def _binary_op(left, right, op, neutral): r = AD() 1_keys r_keys = = set (left.keys()) if isinstance (left, dict) else set() set (right.keys()) if isinstance (right, dict) else set() for k in 1 keys | r_keys: # If the right (or left) element is a dictionary (or an AD), # we get the elements from the dictionary; else we use the right # or left value itself. This implements a sort of dictionary # broadcasting. 1_val = r_val r[k] return r = = left.get(k, neutral) if isinstance (left, dict) else left right.get(k, neutral) if isinstance (right, dict) else right op (1 val, r_val) For the first three problems, we will be writing methods to extend the Pantry class, defined below, that holds cooking ingredients. Notice that Pantry is a subclass of AD. Additionally, we will be using a Recipe class that specifies the ingredients that are required to make a dish.
Step by Step Solution
3.38 Rating (148 Votes )
There are 3 Steps involved in it
Step: 1
Thank you for providing the AD Arithmetic Dictionary class and introducing the Pantry class To extend the Pantry class well need to define additional ...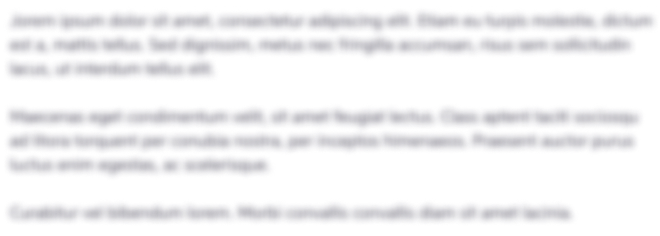
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started