Question
Python Codewriting Question . NEED SOME EDITING to my solution =) some lines could be done in pseuvdocode if I forgot to specify something. The
Python Codewriting Question. NEED SOME EDITING to my solution =)
some lines could be done in pseuvdocode if I forgot to specify something. The changes can only be made withing "#BEGIN EC and END EC", and next to most of the I say ***This part should be good, I think****, which means it shoulldn't need any editing, unless it's really wrong in your opinion.
**** I will also add a screenshot of the error I get now when testing in the end of the post***
I coded most the question already, but need some help putting it all together. Thank you!
Here's the question:
Implement two final thrower ants that do zero damage, but instead produce a temporary "effect" on the action_method of a Bee instance that they throw_at. This effect is an alternative action that lasts for a certain number of .action(colony) calls, after which the Bee's action reverts to its original behavior.
We will be implementing two new ants that subclass ThrowerAnt.
SlowThrower throws sticky syrup at a bee, applying a slow effect for 3 turns.
ScaryThrower intimidates a nearby bee, causing it to back away instead of advancing. (If the bee is already right next to the Hive and cannot go back further, it should not move.) The scare effect lasts for 2 turns. Once a bee has been scared once, it can't be scared again.
Class | Food Cost | Armor |
SlowThrower | 4 | 1 |
ScaryThrower | 6 | 1 |
In order to complete the implementations of these two ants, you will need to set their class attributes appropriately and implement the following three functions:
make_slow is an effect that takes an action method and a bee, and returns a new action method that performs the new action on turns where colony.time is even and does nothing on other turns.
make_scare is an effect that takes an action method and a bee, and returns a new action method that makes the bee go backwards.
apply_effect takes an effect (either make_slow or make_scare), a Bee, and a duration. It uses the effect on the bee.action method to produce a new action method, and then arranges to have the new method become the bee's action method for the next duration times that .action is called, after which the previous .action method is restored.
Hint: to make a bee go backwards, consider adding an instance variable indicating its current direction. Where should you change the bee's direction? Once the direction is known, how can you modify the action method of Bee to move appropriately? ***I'm using self.move_to(self.place.entrance) here for moving backwards and self.move_to(self.place.exit) for moving forward normally, when those two special ants are not attacking the bees.***
Hint: To prevent the same bee from being scared twice, you will also need to add an instance variable to Bee and set it appropriately! ***I create a instance attribute in the Bee class bee.scared_once and set it to False at first, and then to True***
class Bee(Insect): """A Bee moves from place to place, following exits and stinging ants."""
name = 'Bee' damage = 1 is_watersafe = True #don't mind this scared_once = False currently_in_scare_1 = 0 # durat = 0 # OVERRIDE CLASS ATTRIBUTES HERE
def action(self, colony): """A Bee's action stings the Ant that blocks its exit if it is blocked, or moves to the exit of its current place otherwise.
colony -- The AntColony, used to access game state information. """ destination = self.place.exit # NORMAL WAY # Extra credit: Special handling for bee direction # BEGIN EC ***This needs some editing*** if self.scared_once and self.durat: if self.place.entrance is not colony.hive: self.move_to(self.place.entrance) elif not self.durat or not self.scared_once: # END EC if self.blocked(): self.sting(self.place.ant) elif self.armor > 0 and destination is not None: self.move_to(destination)
def make_slow(action, bee): #(action_method, bee_instance) """Return a new action method that calls ACTION every other turn.
action -- An action method of some Bee """ # BEGIN Problem EC ***This part should be good, I think**** def new_action_method_sl(colony, duration=3): # used to be (colony) only #??? what is best to take in??? if not colony.time % 2: #performs new_action on even colony.time return action(colony) return #or do nothing othervise return new_action_method_sl #just a method # END Problem EC
def make_scare(action, bee): #(bee.action, bee_instance) """Return a new action method that makes the bee go backwards.
action -- An action method of some Bee """ # BEGIN Problem EC *** This needs a little review/correction *** def new_action_method_sc(colony, duration=2): #added duration #??? what is best to take in??? #commented out while bee.duration: if bee.scared_once == True: if bee.currently_in_scare_1: apply_effect(make_slow, bee, duration) bee.currently_in_scare_1 -= 1 # might be DECREMENTING TWICE DELETE return # otherwise if bee.scared_once == False: # bee.currently_in_scare_1 = 2 #did it in ScaryThrower if next_to_hive: #### Need to edit pseudocode! return apply_effect(make_slow, bee, duration) else: go_backwards #### Need to edit pseudocode! #not sure this should be here bee.duration -=1 return new_action_method_sc #just a method # END Problem EC
def apply_effect(effect, bee, duration): #(slow OR scare, bee, 3 or 2) """Apply a status effect to a BEE that lasts for DURATION turns.""" # BEGIN Problem EC *** This needs most review/correction, can't figure out how to make sure it tracks duration for multiple function calls and how to make sure apply_effect handles both make_slow and make_scare simultaneously but with separate duration's*** original_action = bee.action # preserve original method new_action = effect(bee.action, bee) # new__action method duration = duration # prevents mutation of bee.currently_in_scare_1 #### /|\ might be Just for clarity, remember to Delete!!!! this silly line ***I'm very confused here, haha
def inner(colony): nonlocal duration # bee.durat = duration while duration: bee.action = new_action bee.action(colony) # do IIII need to call .action or smth else, like return ??? duration -= 1 # new_action(colony) # 3.2 on my diagram, check it again later bee.action = original_action # after that original restored bee.action(colony) # do I???? # bee.durat = duration return inner # bee.action = inner # END Problem EC
class SlowThrower(ThrowerAnt): """ThrowerAnt that causes Slow on Bees."""
name = 'Slow' food_cost = 4 armor = 1 # BEGIN Problem EC ***This part should be good, I think**** implemented = True # Change to True to view in the GUI
def throw_at(self, target):
if target: apply_effect(make_slow, target, 3)
# END Problem EC
class ScaryThrower(ThrowerAnt): """ThrowerAnt that intimidates Bees, making them back away instead of advancing."""
name = 'Scary' food_cost = 6 armor = 1 # BEGIN Problem EC implemented = True # Change to True to view in the GUI # END Problem EC
def throw_at(self, target): # BEGIN Problem EC **** This part needs review, I might be decrementing duration twice: once here and once somewhere above, and I don't understand how to call this function multiple times with its separate duraion's**** ### if target.scared_once == False: target.currently_in_scare_1 = 2 target.scared_once = True if target.currently_in_scare_1: ## make_scare will decrement it apply_effect(make_scare, target, target.currently_in_scare_1) # return or just call??? # if target.scared_once and target.currently_in_scare_1 == 0: #can take out later # return # do nothing else: #idk return # do nothing
# back away / (stay if at the back) + still for 2 turns # END Problem EC
CLARIFICATION ***Bees start at the Hive and move forward from tonnel_9 to tonnel_0 (where they win) or when moowing backwards they should move from lower number to higher, or stand still if the Hive is behing them***
With that in mind, here's the error:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
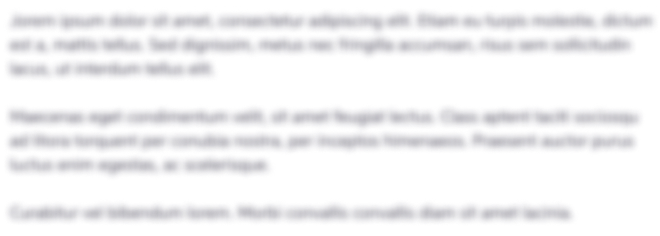
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started