Question
Python Coding: 1. Write a recursive function for OurList of integers that returns the largest value of them. 2. Write a recursive function for OurList
Python Coding:
1. Write a recursive function for OurList of integers that returns the largest value of them.
2. Write a recursive function for OurList of characters that reverses their order.
3. Modify the program of drawing bullseye (bullseyeDemo.py) to draw Pyramid. Specify the number of levels and
the overall width (which can be the same as the height). Unlike the outer circle and inner
circle bullseye were concentric, you will need to relocate the bottom rectangle and the upper
pyramid to achieve the desired effect. Move the components so that the completed pyramid
sits in the middle of the canvas (the default reference point).
***Bullseye Demo***
from cs1graphics import *
class Bullseye(Drawable):
"""Represent a bullseye with an arbitrary number of bands."""
def __init__(self, numBands, radius, primary='black',
secondary='white'):
"""Create a bullseye object with alternating colors.
The reference point for the bullseye will be its center.
numBands the number of desired bands (must be at least 1)
radius the total radius for the bullseye (must be positive)
primary the color of the outermost band (default black)
secondary the color of the secondary band (default white)
"""
if numBands <= 0:
raise ValueError('Number of bands must be positive')
if radius <= 0:
raise ValueError('radius must be positive')
Drawable.__init__(self) # must call parent constructor
self._outer = Circle(radius)
self._outer.setFillColor(primary)
if numBands == 1:
self._rest = None
else: # create new bullseye with one less band, reduced radius, and inverted colors
innerR = float(radius) * (numBands-1) / numBands
self._rest = Bullseye(numBands-1, innerR, secondary, primary)
def _draw(self):
self._beginDraw() # required protocol for Drawable
self._outer._draw() # draw the circle
if self._rest:
self._rest._draw() # recursively draw the rest
self._completeDraw() # required protocol for Drawable
paper = Canvas(400, 400)
simple = Bullseye(3, 60)
simple.move(65, 80)
paper.add(simple)
blue = Bullseye(10, 120, 'darkblue', 'skyblue')
paper.add(blue)
blue.move(195,120)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
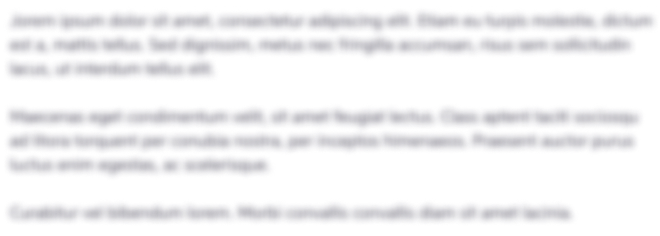
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started