Question
Python: Computer card games are more fun if you can see the images of the cards in a window, as shown in the screen shot
Python: Computer card games are more fun if you can see the images of the cards in a window, as shown in the screen shot in Figure 9-8.
Assume that the 52 images for a deck of cards are in a DECK folder, with the file naming scheme
import random
class Card(object): """ A card object with a suit and rank."""
RANKS = (1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13)
SUITS = ('Spades', 'Diamonds', 'Hearts', 'Clubs')
def __init__(self, rank, suit): """Creates a card with the given rank and suit.""" self.rank = rank self.suit = suit def __str__(self): """Returns the string representation of a card.""" if self.rank == 1: rank = 'Ace' elif self.rank == 11: rank = 'Jack' elif self.rank == 12: rank = 'Queen' elif self.rank == 13: rank = 'King' else: rank = self.rank return str(rank) + ' of ' + self.suit
import random
class Deck(object): """ A deck containing 52 cards."""
def __init__(self): """Creates a full deck of cards.""" self.cards = [] for suit in Card.SUITS: for rank in Card.RANKS: c = Card(rank, suit) self.cards.append(c)
def shuffle(self): """Shuffles the cards.""" random.shuffle(self.cards)
def deal(self): """Removes and returns the top card or None if the deck is empty.""" if len(self) == 0: return None else: return self.cards.pop(0)
def __len__(self): """Returns the number of cards left in the deck.""" return len(self.cards)
def __str__(self): """Returns the string representation of a deck.""" result = '' for c in self.cards: result = self.result + str(c) + ' ' return result
def main(): """A simple test.""" deck = Deck() print("A new deck:") while len(deck) > 0: print(deck.deal()) deck = Deck() deck.shuffle() print("A deck shuffled once:") while len(deck) > 0: print(deck.deal())
if __name__ == "__main__": main()
Cards Demo 0 Cards Demo 2 of Spades Queen of Hearts Deal Shuffle New deck Dea Shuffle New deck Figure 9-8 Viewing images of playing cards 2 GPL 1c.gif 1d.gif 1h.gif 2 2 2d.gif 2h.gif 2s.gif Cards Demo 0 Cards Demo 2 of Spades Queen of Hearts Deal Shuffle New deck Dea Shuffle New deck Figure 9-8 Viewing images of playing cards 2 GPL 1c.gif 1d.gif 1h.gif 2 2 2d.gif 2h.gif 2s.gifStep by Step Solution
There are 3 Steps involved in it
Step: 1
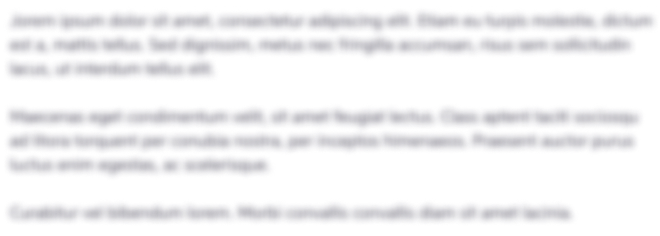
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started