Question
Python: create a complete program that uses classes to store, search, remove, and filter country data. The two major tasks are outlined below 1.? ?Implement
Python: create a complete program that uses classes to store, search, remove, and filter country data. The two major tasks are outlined below
1.? ?Implement a class Country Instance Variables:
i. Name: string
ii. Population: integer
iii. Area: float
iv. Continent: string
Methods:
i. constructor
ii. Getter Methods: getName, getPopulation, getArea, getContinent,
iii. getPopDensity: This calculates and returns the population density for the country. Population density is the population divided by the area.
iv. Setter Methods: setPopulation
v. def ?__repr__(self):generate a string representation for the class Name in Continent
e.g China in Asia class ?Country :
def ?__init__(self, name, pop, area, continent) :
def ?__repr__(self):
def ?setPopulation(self, pop):
def ?getName(self) :
def ?getArea(self) :
def ?getPopulation(self) :
def ?getContinent(self):
def ?getPopDensity(self) :
2. Implement a class called CountryCatalogue This class has two instance variables, catalogue (?that is a set or dictionary or list of countries) and cDictionary? (a dictionary).
This class will have the following methods.
Constructor:? this method will open the specified files and then first will create the cDictionary and then create countries and add them to catalogue. The constructor is given additional parameters, continentFileName and countryFileName, both strings. Major Steps
1. Fill the Dictionary:? open the file continentFileName and fill cDictionary. The key is the country name, while the continent is the value.
2. Fill the Catalogue:? Open the file countryFileName, then read each line of the file and from that create a country and add it to catalogue.
A sample data file has been included data.txt (Note that both files have headers). Do not forget to close any files that are opened in your methods.
addCountry:? given four parameters countryName (a string), countryPopulation (an integer), countryArea (a float), countryContinent (a string), first create a country using this information. If there is already a country with the same name, return False. Otherwise, there is a new country to add, add the newly created country to catalogue, make sure you add the continent to the cDictionary. Return True after successfully adding the new country. Hint: you may find the keys of cDictionary useful.
deleteCountry:? given a parameter countryName (a string), if there is a country with this name then it should be removed from the country catalogue entirely (both cDictionary and catalogue). Print a message informing the user whether the country has been successfully removed from the country catalogue or not. Do not return anything.
findCountry:? given a parameter countryName (a string), if this country exists then return the country from catalogue. If the country does not exist, return None.
filterCountriesByContinent:? given a parameter continentName (a string), return a list containing all the countries that are on continents that are continentName.
printCountryCatalogue:? print the countries of catalogue to the screen using the default print for the Country Class. This method takes no additional parameters.
setPopulationOfASelectedCountry?: Given two parameters countryName (a string) and countryPopulation (an integer), set the population of the country named countryName (if it is in the catalogue) to the value countryPopulation. Return True if the population is updated, and return False otherwise.
findCountryWithLargestPop?: find the country with the largest population, return the name of this country. This method takes no additional parameters.
findCountryWithSmallestArea:? find the country with the smallest area, return the name of this country. This method takes no additional parameters.
filterCountriesByPopDensity:? given two parameters lowerBound and upperBound (both integers), find all the countries (, i.e. country objects) that have a population density that falls within these two numeric bounds inclusively. Return a list that contains all of these countries.
findMostPopulousContinent?: find the continent with the most number of people living in it. Return together the name of this continent with its total population. That is, if mostPopCont is the name of the continent found above and popMaxCont is the total population of this continent, use return?(mostPopCont, popMaxCont).
saveCountryCatalogue:? given a parameter filename (a string), write the country data of the catalogue as specified below to file filename. Each entry should be formatted as below, one country per line. Return the number of lines written to filename.
Format: Name|Continent|Population|PopulationDensity
For example:? China|Asia|1200000000|14.56
class ?CountryCatalogue: def ?__init__(self, continentFileName, countryFileName):
def ?filterCountriesByContinent(self,continentName): def ?printCountryCatalogue(self):
def ?findCountry(self, countryName):
def ?deleteCountry(self, countryName):
def ?addCountry(self, countryName, countryPopulation, countryArea, countryContinent):
def ?setPopulationOfASelectedCountry(self, countryName, countryPopulation):
def ?saveCountryCatalogue(self, filename):
def ?findCountryWithLargestPop(self):
def ?findCountryWithSmallestArea(self):
def ?findMostPopulousContinent(self):
def ?filterCountriesByPopDensity(self, lowerBound, upperBound):
Test your CountryCatalogue class to make sure all functionality is working.
continenet.txt
Country,Continent
China,Asia
United States of America,North America
Brazil,South America
Japan,Asia
Canada,North America
Indonesia,Asia
Nigeria,Africa
Mexico,North America
Egypt,Africa
France,Europe
Italy,Europe
South Africa,Africa
South Korea,Asia
Colombia,South America
data.txt
Country|Population|Area
China|1,339,190,000|9,596,960.00
United States of America|309,975,000|9,629,091.00
Brazil|193,364,000|8,511,965.00
Japan|127,380,000|377,835.00
Canada|34,207,000|9,976,140.00
Indonesia|260,581,100|1,809,590.97
Nigeria|186,987,563|912,134.45
Mexico|128,632,004|1,969,230.76
Egypt|93,383,574|1,000,000.00
France|64,668,129|541,656.76
Italy|59,801,004|300,000.00
South Africa|54,978,907|1,222,222.22
South Korea|50,503,933|98,076.92
Colombia|48,654,392|1,090,909.09
Step by Step Solution
There are 3 Steps involved in it
Step: 1
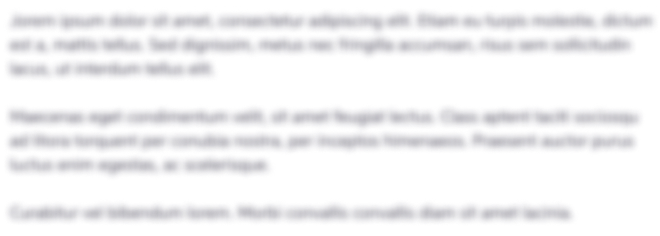
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started