Question
(Python) I am having an issue with calculating the average. Apparently, my output for the average is incorrect. Where did I go wrong? Here is
(Python)
I am having an issue with calculating the average. Apparently, my output for the average is incorrect. Where did I go wrong?
Here is my work:
import csv
def calculateAverageSalary(filename): '''Your code here''' avg = 0 with open(str(filename), 'r') as file: reader = file.readlines() for row in reader: line = row.split(',') if 'Salary' not in line[2]: if avg != 0: avg = (float(line[2]) + avg) / 2 elif avg == 0: avg = float(line[2]) return round(avg, 2)
def getMonth(date): """ Takes in input date as a string in format MM/DD/YY Returns some representation of the string """ newdate = date.split('/') month = newdate[0] return int(month)
def highestHireMonth(filename): '''Your code here''' calculateAverageSalary('employee_info.csv') max = 0 sddict = {} with open(str(filename), 'r') as file: reader = file.readlines() for row in reader: line = row.split(',') if 'Salary' not in (line[2]): salary = float(line[2]) if 'Hire-Date' not in (line[1]): date = str(line[1]) if ('Hire-Date' not in (line[1])) and ('Salary' not in (line[2])): sddict[salary] = date max = 0 for i in sddict: if float(i) > max: max = float(i) out = (getMonth(str(sddict[max]))) return out
if __name__ == "__main__": """Test your functions here""" print(calculateAverageSalary('employee_info.csv')) print(highestHireMonth('employee_info.csv'))
9.15 Breakout Room Activity 2 Many applications require parsing through and processing csv files to extract information. This lab will serve as an introduction for file parsing and will require you to figure out 2 pieces of information You're given a csv file employee_info.csv with the following structure: Name, Hire-Date, Salary Graham Chapman, 03/15/14, 50000.0 John Cleese, 06/01/15, 65000.0 Eric Idle, 05/12/14, 45000.0 Terry Jones, 11/01/13, 70000.0 Terry Gilliam, 08/12/14, 48000.0 Michael Palin, 05/23/13, 66000.0 Write functions: calculateAverage Salary(filename): Returns the average salary of all employees rounded down to 2 decimal points highestHireMonth(filename): Returns the month (integer) during which most hires were made over the years getMonth(date): Helper function to extract and return the month number from a date given as a string input of format MM/DD/YY. Return type should be an intStep by Step Solution
There are 3 Steps involved in it
Step: 1
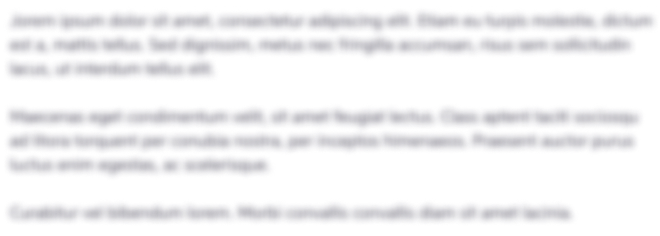
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started