Question
Python I'm not sure what an algorithm is supposed to look like please show me what an algorithm for this would look like! Greedy Coins
Python I'm not sure what an algorithm is supposed to look like please show me what an algorithm for this would look like!
Greedy Coins Game
Greedy Coins is a simple two player coin game, played by flipping 3 coins. The first player to reach or surpass 20 is the winner. Each player takes turns flipping the 3 coins, they add to the pot with each turn, having to decide to flip again and increase the pot, or cash out. The risk being they could lose the amount theyve accumulated into the pot.
The Rules for each player flip.
Flip the 3 coins.If there are no heads ( all tails ),
The pot gets set to zero
The other player goes to step 1.
The number of heads is added to the pot.
User can choose to hold or flip again.
Choice FLIP. Return to Step 1.
Choice HOLD.
Increment Player score by the pot amount.
Pot gets set to 0.
Second player gets to flip and goes to Step 1.
Program Requirements:
Before each opponent begins flipping.
Output the score for the person and the computer.
Output the opponents whose turn is beginning and ask the user to hit enter to continue.
With each flip of the coins.
Output the result, and amount of the round pot.
If its the users flip ask if they want to Flip again ( F ) or Hold ( H ). Your program should allow r, R, h or H as valid input.
The AI will continue playing until the round pot is 8 or more.
Once a players pot is greater or equal to 20 then they have won, it will no longer ask if they want to keep playing.
Once there is a winner
Score totals are output along with who the winner was. User or Computer
Player is asked if they want to play again Y or N. Valid input should be y, Y, yes, YES, or n, N, no, NO.
When a new game starts the starting flip goes to the player that did not flip last. If the User flipped last in the previous game, then the computer flips first and vice versa.
Development Notes:
You will need a way to flip coins in your program. Python has a module just for random numbers. The module is called random
import random #Make this your first line of code. Allows you to use the random module.
To Get a coin flip you will want a number from 0 to 1 :
coin = random.randint(0, 1)
flip is the variable name. Obviously, you can use whatever name you like.
Strings have two methods that could be useful. .lower() and .upper(). These methods return a string that is lowercased or upper cased respectively.
abcLower = "ABC".lower() # abcLower is "abc"
abcUpper = "abc".upper() # abcUpper is "ABC"
The membership operator in can be useful as well, but is not required.
Example
>>> ================================ RESTART ================================
>>>
WELCOME TO GREEDY DICE
SCORE You : 0 AI : 0
Your turn. Hit enter to continue.
COINS : HHH Pot : 3 (F)lip Again or (H)old? f
COINS : TTH Pot : 4 (F)lip Again or (H)old? f
COINS : HHH Pot : 7 (F)lip Again or (H)old? f
COINS : TTH Pot : 8 (F)lip Again or (H)old? f
COINS : THT Pot : 9 (F)lip Again or (H)old? f
COINS : THH Pot : 11 (F)lip Again or (H)old? f
COINS : THH Pot : 13 (F)lip Again or (H)old? f
COINS : TTH Pot : 14 (F)lip Again or (H)old? h
SCORE You : 14 AI : 0
Its the computers turn. Hit enter to continue.
COINS : TTH Pot : 1
COINS : TTT Pot : 1 BUST
SCORE You : 14 AI : 0
Your turn. Hit enter to continue.
COINS : HTT Pot : 1 (F)lip Again or (H)old? f
COINS : HHT Pot : 3 (F)lip Again or (H)old? f
COINS : TTH Pot : 4 (F)lip Again or (H)old? f
COINS : TTT Pot : 4 BUST
SCORE You : 14 AI : 0
Its the computers turn. Hit enter to continue.
COINS : HHH Pot : 3
COINS : HTH Pot : 5
COINS : HHT Pot : 7
COINS : THH Pot : 9
SCORE You : 14 AI : 9
Your turn. Hit enter to continue.
COINS : THT Pot : 1 (F)lip Again or (H)old? f
COINS : HHH Pot : 4 (F)lip Again or (H)old? f
COINS : HHT Pot : 6
Congratulations, you won
SCORE You 20 AI 9
Do you want to play Greedy Coin again? (YES/Y/NO/N) e
Do you want to play Greedy Coin again? (YES/Y/NO/N) y
SCORE You : 0 AI : 0
Its the computers turn. Hit enter to continue.
COINS : TTH Pot : 1
COINS : TTH Pot : 2
COINS : TTT Pot : 2 BUST
SCORE You : 0 AI : 0
Your turn. Hit enter to continue.
COINS : TTT Pot : 0 BUST
SCORE You : 0 AI : 0
Its the computers turn. Hit enter to continue.
COINS : TTH Pot : 1
COINS : HTH Pot : 3
COINS : TTH Pot : 4
COINS : HTH Pot : 6
COINS : THH Pot : 8
SCORE You : 0 AI : 8
Your turn. Hit enter to continue.
COINS : THT Pot : 1 (F)lip Again or (H)old? e
COINS : THT Pot : 1 (F)lip Again or (H)old? f
COINS : TTT Pot : 1 BUST
SCORE You : 0 AI : 8
Its the computers turn. Hit enter to continue.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
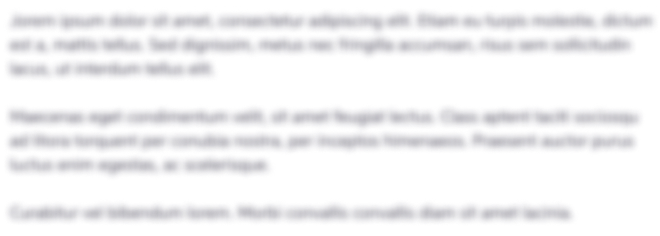
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started