Question
Python: In this assignment youll create a script that combine modules and classes. The classes will be created in separate files and imported into the
Python:
In this assignment youll create a script that combine modules and classes. The classes will be created in separate files and imported into the main program, lab4.1.py. Your lab should generate plain text output that is organized and readable. This problem requires modifying the Shape and Circle classes found in Chapter 3.
lab4.1.py uses the Shape and Circle classes found in Chapter 3.
shape.py is a module that contains the Shape class, the parent class of geometric shapes such as Circle.
circle.py is a module that contains the Circle class. Class Circle is a subclass of Shape. As a subclass, Circlewill import shape.py.
lab4.1.py will:
Import several modules:
shape.py
circle.py
Create two Circle objects
Move the two circle objects around the x,y co-ordinate system by changing their x and y coordinates
Determine whether the circles collide with each other. Collide means the if two circles occupy the same space at the same time, they are colliding. This means that if they intersect at any point or points, they are colliding. This animated example will help you visualize how your script will generate a series
of circles and report whether they are colliding.
Here is the code for shape.py:
#import math class Shape: def __init__(self, x, y): self.x = x self.y = y
def move(self, deltaX, deltaY): ''' Moves Shape object by adding values to the object's x and y coordinates. ''' self.x = self.x + deltaX self.y = self.y + deltaY def __str__(self): ''' Return class name, x, and y ''' return "{}, x:{}, y:{}".format(type(self).__name__, self.x, self.y)
def location(self): ''' Returns a tuple containing the (x,y) coordinates of a Shape object ''' tup = (self.x, self.y) return tup
def _main(): ''' Testing Shape class move(), location(), and __str__() methods ''' print("--- START ---") i2 = 0 c1 = Shape(0, 5) c2 = Shape(5, 5) for i in range(10): i2 += i c1.move(i, i) c2.move(i, i2) print('--') print('Shape 1: ',c1) print('Shape 2: ',c2) print(c1.location()) print(c2.location()) print("--- END ---") _main() # The Shape._main() function will be executed when this file is run as a standalone script. #if __name__ == '_main': # _main()
Here is the code for circle.py :
import math from shape import Shape
class Circle(Shape): pi = 3.14159265359 def __init__(self, x=0, y=0, radius=1): Shape.__init__(self, x, y) self.radius = radius
def area(self): return math.pi * self.radius ** 2
def __str__(self): return Shape.__str__(self) + ", radius: {radius}, area: {area:.2f}".format(radius=self.radius, area = self.area())
@classmethod def is_collision(self,c1, c2): ''' Test whether two circle objects are occupying the same space. YOUR CODE GOES HERE ''' ########################################## # TODO TODO TODO --- COMPLETE THIS METHOD ########################################## a = self.distance(c1,c2) if a <= (c1.radius + c2.radius): return True return None
@classmethod def distance(self,c1,c2): a = c1.location() b = c2.location() #return math.sqrt((c1.x -c2.x) * (c1.x -c2.x) + (c1.y -c2.y) * (c1.y -c2.y)) return math.sqrt((a[0] - b[0]) * (a[0] - b[0]) + (a[1] - b[1]) * (a[1] - b[1]))
def _main():
print("--- START ---") c1x = -10 c1y = 0 c2x = 10 c2y = 0 i1 = 0 i2 = 0 c1 = Circle(0, 0, 5) c2 = Circle(0, 0, 5) for i in range(10): print('--') print('Circle 1: ',c1) print('Circle 2: ',c2) print("Collision: ",Circle.is_collision(c1, c2)) i1 += i i2 -= i c1.move(i, i1) c2.move(i, i2) print("c1 Final location: ", c1.location()) print("c2 Final location: ", c2.location()) print(c1) print(c2) print("--- END ---")
_main()
# Testing code: run this module as a standalone script #if __name__ == '_main': # _main()
Heres some code to get you started with lab4.1.py. Feel free to modify and improve upon it.
#!/usr/local/bin/python3 # NAME: YOUR NAME # FILE: lab4.1.py # DATE: <2016-07-01 fri> # DESC: Exercise in modules from shape import Shape from circle import Circle c1 = Circle(100, 100, 100) c2 = Circle(150, 150, 100) c1_xdelta = 2 c1_ydelta = 3 c2_xdelta = 1 c2_ydelta = -1 # Test 20 (or more) times for i in range(1,20): # c1 c1.move(c1_xdelta, c1_ydelta) c2.move(c2_xdelta, c2_ydelta) # Print c1.__str__() print(c1) # Print c2.__str__() print(c2) # Print collision True or None print("Collision: {collision}".format(collision = Circle.is_collision()) # Change the xdelta, ydeltas for each object
You output might resemble this (it may look a lot like the module test output).
% python3 circle.py -- ~Circle~ 1: Circle: x,y; coordinates: 0, 0; radius: 5. ~Circle~ 2: Circle: x,y; coordinates: 0, 0; radius: 5. Collision: True -- ~Circle~ 1: Circle: x,y; coordinates: 0, 0; radius: 5. ~Circle~ 2: Circle: x,y; coordinates: 0, 0; radius: 5. Collision: True -- ~Circle~ 1: Circle: x,y; coordinates: 1, 1; radius: 5. ~Circle~ 2: Circle: x,y; coordinates: 1, 1; radius: 5. Collision: True ...
Step by Step Solution
There are 3 Steps involved in it
Step: 1
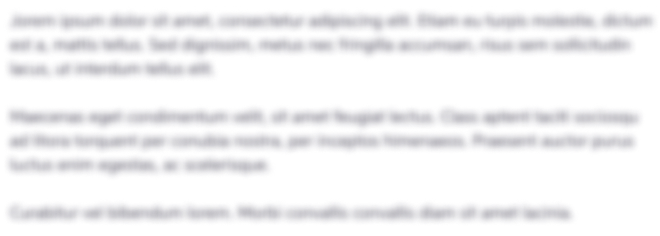
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started