Question
Python Instructions - Add code to the program so the table includes its hexadecimal equivalents by using a do-while loop. The program is correct, all
Python
Instructions - Add code to the program so the table includes its hexadecimal equivalents by using a "do-while" loop. The program is correct, all it needs is the hexadecimal equivalents column. Guidelines to follow:
- Must use a "do-while" loop to generate the hexadecimal numbers
- Program should print results to Console without using any of the Python conversion functions
*NOTE* Comments with explanation to fully understand the process of the program will be much appreciated
Output Example:
Enter the low number:
1
Enter the high number:
10
Decimal Binary Octal Hexadecimal
1 1 1 1
2 10 2 2
3 11 3 3
4 100 4 4
5 101 5 5
6 110 6 6
7 111 7 7
8 1000 10 8
9 1001 11 9
10 1010 12 A
Source Code to modify to add hexadecimal equivalents:
def main(): low = int(input("Enter low number: ")) high = int(input("Enter high number: ")) print("Decimal\t\tBinary\t\tOctal") for i in range(start, end + 1): x = decToBinary(i) y = decToOctal(i) print(i, "\t\t", end=" ") print("".join(str(j) for j in x), "\t\t", end=" ") print("".join(str(q) for q in y))
def decToBinary(decimal): a = [] while(decimal > 0): dig = decimal % 2 a.append(dig) decimal = decimal // 2 a.reverse() return a
def decToOctal(decimal): a = [] octalNum = [0] * 100 i = 0 while(decimal != 0): octalNum[i] = decimal % 8 decimal = int(decimal / 8) i += 1 for j in range(i - 1, -1, -1): a.append(octalNum[j]) return a
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
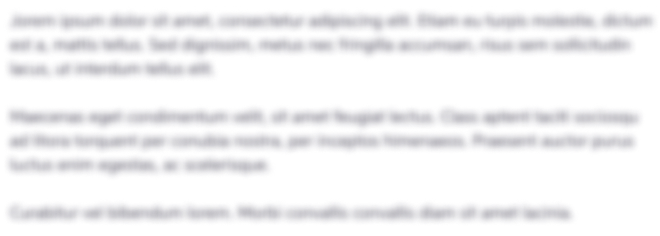
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started