Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Python Part 2: Guess the song For the second part of this project, you will write code that implements a song-guessing game. Part 2.1: Pick
Python
Part 2: Guess the song For the second part of this project, you will write code that implements a song-guessing game. Part 2.1: Pick a random song After creating a list of songs (part 1), your code should pick a song at random from that list. You should use the random.choice () method for this (be sure to import the random module). Part 2.2: Play a song preview After picking a random song (2.1), your code should play an audio preview of the song. To do this, you must import a few features from the IPython.display module: from IPython.display import display, Audio, clear_output After you do this, if you are given a URL for an audio file (suppose it is in the variable audio_url ), you can play it with: display (Audio (audio_url, autoplay=True)) For example, you can try this with the audio url: 'https://audio-ssl.itunes.apple.com/itunes-assets/Music7/v4/cd/a8/4c/cda84ca4-3ef1-d2b5-6f88-3640e5db33fc/mzaf_1355556193908011287.plus.aac.p.m4a' Note: If your audio does not play, try adding the following code snippet: import mimetypes mimetypes.init() mimetypes.add_type('audio/mp4', .m4a') Part 2.3: Print a "blanked out" version of the song: After picking a random song (2.1) and playing a song preview (2.2), your code should Replace every alphanumeric character (meaning a-z, 0-9) in the track name with an underscore ('_'). For example, a song titled "(I Can't Get No) Satisfaction" should print out: "(_ ___'_ (I Can't Get No) Satisfaction (___ You can check if a character is alphanumeric with the .isalnum() method: 'a'.isalnum() is True. '#'.isalnum() is False. Part 2.4: Allow the user to guess the track name (or pass) After your code picks a random song (2.1), plays the song preview (2.2), and prints a "blanked out" version of the song (2.3), your code should repeatedly ask the user to guess the song. Every time, your code should: Ask the user to enter a guess (using input()) If the user guessed the correct track name, print "You got it!". If the user guessed incorrectly, print "It's not ' '" If the user enters 'pass' then your code should display the correct answer ("The song was ' '") and stop asking for guesses. This part should not be case sensitive; if the correct answer is 'Thriller' and the user guesses 'THRILLER', this should count as being correct. You can use Python strings.upper() method to achieve this. Part 2.5: Indefinite rounds Finally, your code should repeat parts 2.1--2.4 indefinitely (until the user types 'exit' ). Note that this means you will need to have nested while loops (one for 2.4 and one for this part). One way to achieve this would be to put the code for part 2.4 into a separate function. Every round, your code should: 1. Print == ROUND ==', replacing with the round number ( 1, 2, 3,...) 2. Pick a random song (as implemented for 2.1) 3. Play a preview of the song (as implemented for 2.2) 4. Print a "blanked out" version of the song (as ipmlemented for 2.3) 5. Allow the user to guess the song with an indefinite number of chances (as implemented for 2.4), with the following modification: If the user types 'exit', your code should display the correct answer, wait 3 seconds, and clear the output (see #6 for how to do this) The user should still be able to enter 'skip' to skip to the next round. The difference between 'pass' and 'exit' is that 'pass' should move on to the next round. 'exit' should exit the guessing game entirely. 6. After the user guesses correctly (or passes), your code should: Display the correct track name (if the user passed) . . Wait 3 seconds (you can do this by importing the time module and calling time.sleep (3) Call clear_output() (imported from IPython.display earlier) to clean up the output. 7. Increment the round and start up again at step 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
e implementation for the songguessing game python Copy code import random from IPythondisplay import ...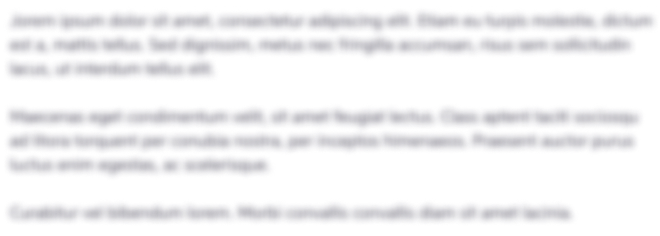
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started