Question
python please Overview In this project, you will create a program to generate and output customer receipts as well as a summary of daily operations
python please
Overview
In this project, you will create a program to generate and output customer receipts as well as a summary of daily operations for a restaurant.
Objectives
The objective of this project is to create a solution combining multiple concepts that have been presented in class, demonstrating an understanding of:
list indexing
list methods
string manipulation
string formatting
while loops
for loops
In addition, you will need to use concepts you have used previously, including
input and output
variables
arithmetic expressions
branching statements (if-else)
Problem Description
Fictional restaurant Sparty Burger needs you to write a program that can handle its daily cash register operations. The program should allow the user to open new orders, add items to that order, and generate a receipt for the transaction which is then printed on the screen. In addition to managing individual orders, the program should also create a summary of the total transactions performed during the program's run.
When the program starts, the program will print a command menu asking the user what they would like to do. The menu is as follows (a blank line should be printed after the colon):
(1) Open a new order (2) Finish operating Enter a number to choose a command:
If an invalid command (not 1 or 2) is entered, a blank line, an error message, and another blank line should be printed, followed by printing the menu again. (Note the blank lines do not show in zyBooks in the example below)
ERROR: Invalid command. Please try again.
If the user enters 1, the program will then print another menu that the user can then use to select items to add to a customer's order. A newline should be printed with the input prompt (where it's asking to enter a selection number). The order menu is as follows:
------------------------------------------------------- Welcome to Sparty Burger! ------------------------------------------------------- (1) Hamburger $6.99 (2) Cheeseburger $7.69 (3) Sparty Melt $8.39 (4) Beaumont Burger $8.69 ------------------------------------------------------- (5) Small Fries $3.09 (6) Medium Fries $4.19 (7) Large Fries $5.79 ------------------------------------------------------- (8) Regular Drink $2.39 (9) Large Drink $2.69 ------------------------------------------------------- (-1) Complete Order (-2) Preview Cart -------------------------------------------------------
In order to make your life less complicated, we have written a function that you can use to print your menu. You do not need to worry about the code for the function (you'll learn how to make your own functions in a future chapter); for now, you just need to know how to use the function. The function is imported in the template starter code, much like you imported the math and random libraries in order to use functions that were defined there. Remember the 3 things you need to know in order to use a function: (1) the name of the function (2) what arguments you need to give it, (3) what it returns. In this case, the name of the function is menuPrint, and you need to give it 2 things: the menu list, and the price list, which are also given in the starter code. The menuPrint function does not return a value, rather it just prints the menu of items and choices to complete the order and preview the cart.
You will need to call the function so that it prints the menu before you ask for the selection number.
If the number provided is invalid (none of the presented options), a blank line, an error message, and a blank line should be printed as follows:
ERROR: Invalid selection. Please try again.
Upon entering a valid number representing a menu item, a prompt should appear asking the user for the item quantity. The prompt is as shown below:
Please enter item quantity:
Valid item quantities are greater than or equal to zero; you cannot have a negative quantity. If a valid, non-zero item quantity is given by the user, the Items menu should be reprinted and ready to accept another item selection. If a non-valid item quantity is given the following error should be printed (Note the blank lines before and after again):
ERROR: Invalid quantity. No items were added to the cart.
Note that the operator is able to reselect an option that was previously entered. For instance, the operator could have just finished entering a quantity for item (2) Cheeseburger. The operator should have the ability to choose item (2) Cheeseburger again when the menu is reprinted. Reselected items should also prompt for a quantity, and for reselected items, the new quantity will overwrite the previous quantity. If the operator entered 1 for the quantity of a given item, reselected the same item later on, and then input a quantity of 2, then the receipt should print a quantity of 2 for that item.
The operator is able to preview what is in the customer's cart by entering a selection of -2. The preview should print each item's quantity, item name, and prices associated with the item and its quantity. Note that the items in the preview appear in the order that they appear in the menu! Print a blank line before and after the previews. An example is shown below: You don't need to worry about plural vs. singular.
3 Hamburger $20.97 1 Large Fries $5.79 1 Large Drink $2.69
After printing the cart preview, the program will then reprint the item menu and prompt the operator for another item selection after previewing.
When there are no more items to be added to the customer's order, the user should input -1 to complete the order. If there were no items added to the customer's cart, no receipts should be printed and the empty order should not be counted towards the orders taken during operations.
If the order was non-empty, the program should generate the customer's receipt showing the order subtotal, collected tax (6% sales tax in Michigan), and full total. The program should add the subtotal to a running count of total revenue, add the tax collected to the running count of total collected tax, and increment the number of orders taken by one. The program will print the receipt in the following manner:
------------------------------------------- | | | Receipt | | | | | | 3x Cheeseburger $ 23.07 | | 2x Sparty Melt $ 16.78 | | 6x Beaumont Burger $ 52.14 | | 4x Regular Drink $ 9.56 | | | ------------------------------------------- | | | Subtotal $ 101.55 | | Tax $ 6.09 | | Total $ 107.64 | | | -------------------------------------------
Another example is:
------------------------------------------- | | | Receipt | | | | | | 3x Hamburger $ 20.97 | | 2x Sparty Melt $ 16.78 | | 4x Large Fries $ 23.16 | | 2x Regular Drink $ 4.78 | | | ------------------------------------------- | | | Subtotal $ 65.69 | | Tax $ 3.94 | | Total $ 69.63 | | | -------------------------------------------
Notice that the receipt lines are all the same length so that the edges of the receipt are nicely aligned. This means that you'll be using string formatting with pre-determined field sizes to create these receipts. Details on field sizes can be found below under 'Details for Receipt Output'.
After the receipt is printed, a blank line should be printed and the user will then be again prompted with the original command menu to either open a new order or end operations.
If the user enters 2 on the command menu prompt, the program will print a blank line and then a summary of the day's operation before the program ends. The summary should appear as follows:
Operation Summary Total revenue: $1456.23 Total tax collected: $87.37 Number of orders taken: 89
Details for Receipt Output
Every line of the receipt should contain exactly 43 characters. The top, bottom, and dividing line of the receipt consist only of the - character 43 times.
Every line beside the starting, ending, and dividing lines should start and end with a | character. These | characters should always have exactly 5 spaces of padding from any proceeding or preceding text.
Blank lines in the receipt (with the starting and ending characters) should be placed:
Before and after the line containing the word 'Receipt'
Before and after the list of items with prices
Before and after the totals (Note this means there are two blank lines after the word 'Receipt' - one after the word 'Receipt' and another one before the items with prices)
The word 'Receipt' should be centered in its line.
For the list of items with prices, each line of the list should have the following fields:
6-character field for a | character followed by 5 spaces.
3-character field for the number of items (includes the x character that precedes the item name), right-aligned
1 space after the number of items field and before the item name field
18-character field for the name of the item (15 characters representing the item name), left-aligned
2-character field for a space and a dollar sign character
7-character field for the price (in dollars and cents), right-aligned
6-character field for 5 spaces followed by a | character
You should have a total of 6 + 3 + 1 + 18 + 2 + 7 + 6 = 43 characters per line.
For the bottom portion of the receipt detailing totals, you should have:
6-character field for a | character followed by 5 spaces of padding
18-character Field for Subtotal, Tax, or Total, right-aligned
6-character field for five spaces and a dollar sign character
7-character field for the amount in dollars and cents (no fractional cents), right-aligned
6-character field for 5 spaces of padding followed by a | character
Again, 6 + 18 + 6 + 7 + 6 = 43 characters
Helpful tips and tricks:
This project is challenging. If you do not start right away and work steadily, you will be in trouble come week 3. To stay on track, you should have the first checkpoint done by the end of the first week, the second checkpoint done by the end of the second week, and the third checkpoint done by the end of the third week. Then take the fourth week to finish things up.
Start by writing out a plan for the steps you will need to take to solve this problem. Write the plan in English, not in Python. Refer to the videos on how to start a project that were posted on the D2L home page for project 1.
Get the main program logic working before you worry about what's happening within each of the branches. For instance, it's a good idea to make sure that you can get the command menus and item menus to open, close, and reopen before you worry about things like adding items to your cart and calculating totals. You can pass the first 10 test cases with just the menus, error messages, and correct control of a path through the program, without worrying about adding any items to the cart, computing anything, or printing the receipt. This should be the focus of your first checkpoint.
Think about what kind of loops you would want to use to accomplish various parts of the program. For instance, what kind of loop would you use if you wanted to keep doing something and don't know for sure when you should stop doing it? To that end, what would make it stop, and what wouldn't? What kind of loop would you use if you do know exactly how many times you had to do something or exactly how many things you have to work with?
Plan out where and how you should have your data so that you can have order-specific totals and items as well as the totals for the full program operation. Each new order should start off empty but you don't want to forget about some information from the orders before.
The starter code includes parallel lists for the menu item names and the menu prices. Parallel lists means that the same index into the menu item names is also the index for the corresponding price. For example, a Beaumont Burger is index 3 in the MenuList, and its price is index 3 in the PriceList. You may find it convenient to use a parallel list for the shopping cart.
Do not work on formatting the receipt before you have the main logic of the program working. While working on the logic, you can just print an "ugly" receipt that has the same info, but just might not be formatted perfectly, or even just a message that says "print receipt here".
When printing an item on the receipt or in the cart preview, you'll have to make sure it's actually in the cart. There are a few ways to do this, so think about how you would tell if the cart is empty, or if an item has not been ordered. And remember that only non empty carts count as an "order" when you are keeping track of the number of orders for the day.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
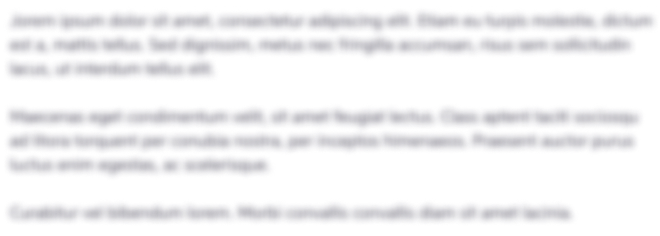
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started