Answered step by step
Verified Expert Solution
Question
1 Approved Answer
python pls! For this assignment, you'll implement Dijkstra's algorithm on adjacency list representations of directed, weighted graphs by adapting your MinHeap class from the previous
python pls!
For this assignment, you'll implement Dijkstra's algorithm on adjacency list representations of directed, weighted graphs by adapting your MinHeap class from the previous heap coding assignment. This assignment is a pure programuning assignment. Implementation Your code must be in a file called dijkstra.py. Your algorithm must be in a function called dijkstra. Starter code is attached to this assignment. Your dijkstra function will take three arguments: an adjacency list representation of a directed, weighted graph; an edge weight dictionary mapping node tuples (edges) to integers (edge weights); and a source node label. Your code should not modify any of the arguments that are passed to it (i.c. it shouldn't mutate the original graph objects). Your function should return a dictionary mapping each node label to its minimum distance from the source node. The source node's distance to itself should be 0. If a node was unreachable from the source node, its distance should be math.inf (from the math standard library module). Your algorithm must run in O(F.log()) time, where is the number of edges and is the number of nodes. Your function must use an adapted version of your MinHeap class from the previous hcap coding assignment. Il you weren't able to create a working version of MinHeap for the previous assignincnt, message Professor Long. Graph representation Graphs will be represented as dictionaries where each key is a node label and each value is a list of labels, representing the node's outgoing edges. For example, the dictionary entry 'a': ['b', 'c'] means the node with label a has two outgoing edges: one to b, and one to c. Labels may be strings, integers. ctc. Edge weights will be represented as dictionaries where each key is a tuple of node labels and each value is an integer weight. For example, the dictionary entry 'c', 'b'): 7 means the cdge from c to b has weight 7. All cdge weights will be non-negative. Your min heap will need to be modified to store both the node labels and their priorities (minimum distances found so far). We recommend storing [label, priority] pairs in your heap array. You'll need to make sure you use the priority for all comparisons when deciding whether to swap. If a shorter path to a node is found, you'll need to update your distance dictionary, but you'll also need to reduce the node's priority in the heap and upheap it. To upheap an arbitrary node efficiently, you'll need to be able to get the index it's stored at in O(1) time (instead of having to search through the entire heap array). We recommend that your class maintains a dictionary mapping node labels to indices, which is updated whenever you swap nodes while up/downheaping. You may make other modifications to your min heap as needed, as long as your implementation is still fundamentally a min heap. We won't test your min heap class or its methods directly. Here's an example of how your dijkstra function should work: graph = { 'a': ['b', 'c'], 'b': ['a', 'c', 'e'], 'c': ['b', 'd', 'e'], 'd': ['a'], 'e': [], 'f': [], 'g': ['e'], } edges = { ('a', 'b'): 2, ('a', 'c'): 7, ('b', 'a'): 4, ('b', 'c'): 3, ('b', 'e'): 10, ('c', 'b'): 6, ('c', 'd'): 4, ('c', 'e'): 1, ('d', 'a'): 3, ('g', 'e'): 2 } distances = dijkstra(graph, edges, 'a') print(distances) # {'a': 0, 'b': 2, 'c': 5, 'd': 9, 'e': 6, 'f': inf, 'g': inf} distances = dijkstra(graph, edges, 'c') print(distances) # {'a': 7, 'b': 6, 'c': 0, 'd': 4, 'e': 1, 'f': inf, 'g': inf} distances = dijkstra(graph, edges, 'g') print(distances) #{'a': inf, 'b': inf, 'c': inf, 'd': inf, 'e': 2, 'f': inf, 'g': 0}Step by Step Solution
There are 3 Steps involved in it
Step: 1
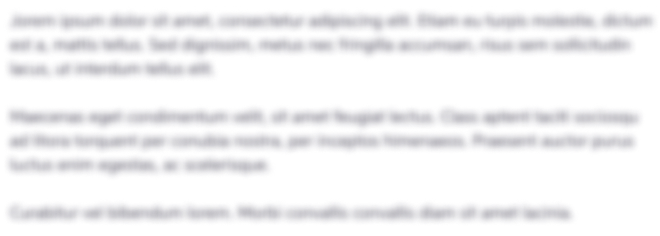
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started