Question
Python pls! Starter code provided at the end. starter code in text: ### ### Author: ? ### Course: ? ### Description: ? ### from graphics
Python pls! Starter code provided at the end.
starter code in text:
### ### Author: ? ### Course: ? ### Description: ? ###
from graphics import graphics
# Some constants to be used throughout the code # The literals 'X' and 'O' and ' ' should not be used elsewhere WHITE = 'O' BLACK = 'X' EMPTY = ' '
def is_move_acceptable(board, turn, pos): ''' Implement ''' pass
def move(board, turn, pos): ''' Implement ''' pass
def get_move(turn): ''' Implement ''' pass
def is_over(board): ''' Implement ''' pass
def get_opposite_turn(turn): ''' Implement ''' pass
def print_board(board): ''' Implement ''' pass
def draw_board(board, gui): ''' Implement ''' pass
def who_is_winner(board): ''' Implement ''' pass
def main(): print('WELCOME TO REVERSI')
gui = graphics(700, 200, 'reversi')
# Initialize an empty list with 12 slots board = [EMPTY] * 12 # State of whether or not the game is over over = False # Starting turn (should alternate as gome goes on) turn = BLACK
# Print out the initial board print_board(board) draw_board(board, gui)
# Repeatedly process turns until the game should end (every slot filled) while not over: place_to_move = get_move(turn) while not is_move_acceptable(board, turn, place_to_move): place_to_move = get_move(turn) move(board, turn, place_to_move)
print_board(board) draw_board(board, gui)
over = is_over(board) turn = get_opposite_turn(turn) print('GAME OVER') print(who_is_winner(board))
main()
CSC 110 - 1 Dimensional Reversi Reversi (also known as Othello) is a simplistic, two-player board game played on a 2-dimensional grid. At the beginning of the game, each player gets half of the total pieces to fit in every slot on the board. Each piece (or "stone") has two sides - white and black. Each player takes turns placing pieces of their color on the board, until the entire board is full. However, when each piece is played, there is a possibility for that player to "flip" some of the existing opponent pieces to the other side, depending on the positions on the board. Whoever has more pieces of their color when the board fill sup wins! For more info on the game, and how the stone-flipping works to the game then this, see here. Implementing a fully-featured game of Reversi might be a daunting task for some of you, especially given the amount of programming experience that you have. Thankfully, in this assignment, you won't have to! Instead, you will be implementing a simpler variant of reversi: 1-Dimensional (10) Reversi! 1D Reversi is a variant of the game that is played on a board that has only one row of spaces, rather than a grid of spaces. For the purposes of this PA, O will represent the white player and the black player. ID Reversi Rules As you might expect, the rules for 10 reversi will be somewhat different that for regular Reversi. In 110 1D reversi, the board will have only 1 row, with 12 total slots. Thus, each player will have the opportunity to place 6 total pieces. Black will get the first move, and it will alternate back and forth until the board is full. Players may choose to put a piece wherever desired on the board, so long as the slot is not already taken by another white or black chip. When a payer places a piece, it is possible that the move will cause some of the other player's pieces to "flip". If there is a sequence of contiguous opponent pieces between where the new piece was placed and another piece on the current players side, then each one in-between flips. For example, say that the board has the current state of: | 10|XX||||||||| and it is white's turn. If white places a piece at position 5, then the two X's at positions 3 and 4 will flip to Os. | 10|0|0|0||||X|10 However, if white placed a piece at position 6 instead, none would flip, because there isn't a contiguous line of Xs between the new piece and an existing piece. | 10|XX|10|||X|||0| An Example Game Below is an example play out of a game of 1D Reversi. Make sure that you understand why each turn worked out the way it did. If you don't understand something, just ask on piazza or in office hours! The starting board is empty: Black (X)goes first, and white (O) follows: x X O Then, X places another piece between it and the O, and places another too. XXO XX 00 Next, X places a piece to flip the two Os hi Xs: X X X X X White places a piece at the beginning of the board: O X X X X X Xplaces one next to o, but o quickly flips it to an X: OX XXXXX xxxxx Next, X places a piece on the other end, and O adds one as position 4: 000 X X X X X x OOOOXXXXX X Xplaces one more piece: OOOOXX X X X X X And then O swoops in for a last-minute win by flipping several Xs to Os. x In this case, O (white) wins! Program Structure For this PA, we will actually be providing some starter code. The starter code will include some constants, a main function (already coded), and a bunch of function definitions, which you will need to implement! Thus, in this PA, you should not attempt build the program completely from scratch. Rather, you should implement a handful of functions to get the game to work properly. Click the link below to download the starter code: reversi.py Before you start working on the functions, make sure you read through the starter code carefully. Restrictions and Requirements . This program must follow the style guidelines. . You should structure all of your code into the provided function definitions. . You are not allowed to change main, other than commenting out the lines for graphics. You are not allowed to have any code outside of a function (other than constants or imports). You should use the provided constants when referring to and spaces. . Make sure you write detailed function comments for every function other than main. Functions to Implement There are a number of functions which you should implement to get the game working properly. There will be both a text representation of the game, and a graphical representation of the game. Initially, don't worry about the graphical representation. Below, I provide a description of what each function should do. It is up to you to implement them, and then test that they are working properly! print_board This functions should have one parameter variable, the board list. The job of this function is to simply print out the board to standard output. If the board is passed in and in the initial game state, the output should look like so: is_move_acceptable This function has three parameter variables. The board (a list, representing the 1 by 12 board), the current players turn (WHITE or BLACK) and the 1-based position of the requested move. This function should return a boolean (True or False). True if the desired move is valid, False otherwise. A Move is valid if The position is a valid location on the board. The position is not already occupied by BLACK or WHITE piece. You do not need a while loop or for loop within this function. It can be accomplished with if statements. If the above two conditions are True, the function should return True. In any other case, return false. move This function has three parameter variables. The board (a list, representing the 1 by 12 board), the current players turn (WHITE or BLACK) and the 1-based position of the requested move. This function should place the piece at the given location. It should also handle the flipping of pieces, if necessary. This function may assume that the move location has already been determined to be valid. You should use one or more for-loops or while-loops for this function. get_move This function should have 1 parameter. The parameter will be either BLACK or WHITE. This function should simple ask the user for an input value, convert it to an integer, and return the number. is_over This function should take the board list as it's only parameter, and should determine if the game is over or not. Basically, the game is over if every slot of the board is filled. It should return True if it is over, and False otherwise. draw_board This game can be played purely on the command line, as long as you have implemented the print_board function. However, you should also implement draw_board. This function should display the board on a graphical canvas using the graphics module. This function has two parameters, the board list and a graphics object. This function should display a graphical representation of the board. This function is already called from main, and the gui variable is defined in main. You should use the gui variable and the board to display it graphically. The board should appear roughly as shown below, but the pieces should show up in the locations that they are in in the board list. revers Evers REVERSI The white pieces should display as )s and the black as Xs. You should also include the string "REVERSI" in the bar and above the board on the canvas. If you have the time and ability, you can make the canvas more interesting. You can even draw out an icon for the king and knight, instead of using a word to represent them. Don't forget to call update_frame at the end of the draw_board function! Also, you'll want to use the text function to display text on the canvas. The text function takes 5 arguments: the x position, y position, the label (text) to display, color, and size. For instance, to display the words "hi there" on a canvas in green, you could run this: gui = canvas(2ee, 2ee, 'canvas') gui.text(50, 50, 'hi there', 'green', 25) gui.update_frame() get_opposite_turn This function should have 1 parameter. The parameter will be either the string BLACK or WHITE. If the parameter is WHITE, return BLACK. If the parameter is BLACK, return WHITE. Submission This PA is due on March 16th, at 7pm. You should turn it in on Gradescope. Remember. There will be an autograder for this, but there will not be an Gradescope autograder test case for the graphics portion. CSC 110 - 1 Dimensional Reversi Reversi (also known as Othello) is a simplistic, two-player board game played on a 2-dimensional grid. At the beginning of the game, each player gets half of the total pieces to fit in every slot on the board. Each piece (or "stone") has two sides - white and black. Each player takes turns placing pieces of their color on the board, until the entire board is full. However, when each piece is played, there is a possibility for that player to "flip" some of the existing opponent pieces to the other side, depending on the positions on the board. Whoever has more pieces of their color when the board fill sup wins! For more info on the game, and how the stone-flipping works to the game then this, see here. Implementing a fully-featured game of Reversi might be a daunting task for some of you, especially given the amount of programming experience that you have. Thankfully, in this assignment, you won't have to! Instead, you will be implementing a simpler variant of reversi: 1-Dimensional (10) Reversi! 1D Reversi is a variant of the game that is played on a board that has only one row of spaces, rather than a grid of spaces. For the purposes of this PA, O will represent the white player and the black player. ID Reversi Rules As you might expect, the rules for 10 reversi will be somewhat different that for regular Reversi. In 110 1D reversi, the board will have only 1 row, with 12 total slots. Thus, each player will have the opportunity to place 6 total pieces. Black will get the first move, and it will alternate back and forth until the board is full. Players may choose to put a piece wherever desired on the board, so long as the slot is not already taken by another white or black chip. When a payer places a piece, it is possible that the move will cause some of the other player's pieces to "flip". If there is a sequence of contiguous opponent pieces between where the new piece was placed and another piece on the current players side, then each one in-between flips. For example, say that the board has the current state of: | 10|XX||||||||| and it is white's turn. If white places a piece at position 5, then the two X's at positions 3 and 4 will flip to Os. | 10|0|0|0||||X|10 However, if white placed a piece at position 6 instead, none would flip, because there isn't a contiguous line of Xs between the new piece and an existing piece. | 10|XX|10|||X|||0| An Example Game Below is an example play out of a game of 1D Reversi. Make sure that you understand why each turn worked out the way it did. If you don't understand something, just ask on piazza or in office hours! The starting board is empty: Black (X)goes first, and white (O) follows: x X O Then, X places another piece between it and the O, and places another too. XXO XX 00 Next, X places a piece to flip the two Os hi Xs: X X X X X White places a piece at the beginning of the board: O X X X X X Xplaces one next to o, but o quickly flips it to an X: OX XXXXX xxxxx Next, X places a piece on the other end, and O adds one as position 4: 000 X X X X X x OOOOXXXXX X Xplaces one more piece: OOOOXX X X X X X And then O swoops in for a last-minute win by flipping several Xs to Os. x In this case, O (white) wins! Program Structure For this PA, we will actually be providing some starter code. The starter code will include some constants, a main function (already coded), and a bunch of function definitions, which you will need to implement! Thus, in this PA, you should not attempt build the program completely from scratch. Rather, you should implement a handful of functions to get the game to work properly. Click the link below to download the starter code: reversi.py Before you start working on the functions, make sure you read through the starter code carefully. Restrictions and Requirements . This program must follow the style guidelines. . You should structure all of your code into the provided function definitions. . You are not allowed to change main, other than commenting out the lines for graphics. You are not allowed to have any code outside of a function (other than constants or imports). You should use the provided constants when referring to and spaces. . Make sure you write detailed function comments for every function other than main. Functions to Implement There are a number of functions which you should implement to get the game working properly. There will be both a text representation of the game, and a graphical representation of the game. Initially, don't worry about the graphical representation. Below, I provide a description of what each function should do. It is up to you to implement them, and then test that they are working properly! print_board This functions should have one parameter variable, the board list. The job of this function is to simply print out the board to standard output. If the board is passed in and in the initial game state, the output should look like so: is_move_acceptable This function has three parameter variables. The board (a list, representing the 1 by 12 board), the current players turn (WHITE or BLACK) and the 1-based position of the requested move. This function should return a boolean (True or False). True if the desired move is valid, False otherwise. A Move is valid if The position is a valid location on the board. The position is not already occupied by BLACK or WHITE piece. You do not need a while loop or for loop within this function. It can be accomplished with if statements. If the above two conditions are True, the function should return True. In any other case, return false. move This function has three parameter variables. The board (a list, representing the 1 by 12 board), the current players turn (WHITE or BLACK) and the 1-based position of the requested move. This function should place the piece at the given location. It should also handle the flipping of pieces, if necessary. This function may assume that the move location has already been determined to be valid. You should use one or more for-loops or while-loops for this function. get_move This function should have 1 parameter. The parameter will be either BLACK or WHITE. This function should simple ask the user for an input value, convert it to an integer, and return the number. is_over This function should take the board list as it's only parameter, and should determine if the game is over or not. Basically, the game is over if every slot of the board is filled. It should return True if it is over, and False otherwise. draw_board This game can be played purely on the command line, as long as you have implemented the print_board function. However, you should also implement draw_board. This function should display the board on a graphical canvas using the graphics module. This function has two parameters, the board list and a graphics object. This function should display a graphical representation of the board. This function is already called from main, and the gui variable is defined in main. You should use the gui variable and the board to display it graphically. The board should appear roughly as shown below, but the pieces should show up in the locations that they are in in the board list. revers Evers REVERSI The white pieces should display as )s and the black as Xs. You should also include the string "REVERSI" in the bar and above the board on the canvas. If you have the time and ability, you can make the canvas more interesting. You can even draw out an icon for the king and knight, instead of using a word to represent them. Don't forget to call update_frame at the end of the draw_board function! Also, you'll want to use the text function to display text on the canvas. The text function takes 5 arguments: the x position, y position, the label (text) to display, color, and size. For instance, to display the words "hi there" on a canvas in green, you could run this: gui = canvas(2ee, 2ee, 'canvas') gui.text(50, 50, 'hi there', 'green', 25) gui.update_frame() get_opposite_turn This function should have 1 parameter. The parameter will be either the string BLACK or WHITE. If the parameter is WHITE, return BLACK. If the parameter is BLACK, return WHITE. Submission This PA is due on March 16th, at 7pm. You should turn it in on Gradescope. Remember. There will be an autograder for this, but there will not be an Gradescope autograder test case for the graphics portionStep by Step Solution
There are 3 Steps involved in it
Step: 1
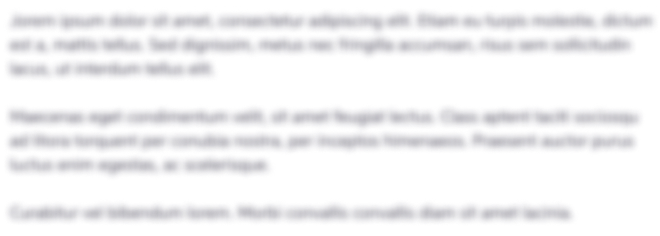
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started