Question
Python Program: There are many variants of the gambling card-game Poker. The classic 5-card draw variant involves ranking a hand consisting of 5 cards from
Python Program:
There are many variants of the gambling card-game "Poker". The classic 5-card draw variant involves ranking a hand consisting of 5 cards from a 52-card deck of cards and then awarding the accumulated "pot" of money to the person with the highest ranking hand. Write a Python program that will simulate "dealing" 100,000 5-card hands and then report the frequency of occurrence of "natural" Poker hands. A "natural hand" is one that is dealt "as-is" without replacing any cards. You should first consult the following wikipedia page for a full description of the ranking of 5-card poker hands: http://en.wikipedia.org/wiki/List_of_poker_hands
Requirements:
You must do the following:
1. Construct an object class named Card that will represent individual playing cards from a standard 52- card deck (no Jokers or special cards). Each Card object will have two instance variables to represent the value and suit of the playing card. The values you choose are up to you. Include the following methods in the Card class:
a. getValue() : Return the value of the card
b. getSuit() : Return the suit of the card
c. __str__() : Return a string representing the card value and suit
2. Construct an object class named Carddeck that will simulate a deck of playing cards. The Carddeck class must include two instance variables: a list of 52 Card objects and an integer "index" value to keep track of the position in the deck from which to deal the next card.
3. The Carddeck class must include the following methods:
a. __repr__() : Return a string with the deck displayed as a list
b. shuffle() : Shuffle the deck (use random.shuffle()) and set the next card index to zero
c. dealcard() : Return the "next" Card object from the deck. Note that after the last card in the deck has been dealt, the deck should be reshuffled automatically!
4. Construct a third object class named Pokerhand that will represent a 5-card poker hand of "cards" dealt from a Carddeck object. The Pokerhand class must include a single instance variable: a list containing 5 Cards.
5. The Pokerhand class must include the following methods: a. newHand(): get 5 new cards from the Carddeck b. __repr__ : Return the hand-list as a string c. rank() : Return the rank of the poker hand as an integer value as follows:
0 : High card
1 : One pair
2 : Two pair
3 : Three of a kind
4 : Straight
5 : Flush
6 : Full house
7 : Four of a kind
8 : Straight flush
Note that this may be somewhat challenging to construct. It will be much simpler if you use the Python container classes set and dict to evaluate the number of different suits and values in the hand. For example, if there is exactly 1 suit, then the hand must be some sort of 'flush'...
6. Write a non-pure function named main that will take no arguments and do the following:
a. Instantiate a single Carddeck object
b. Use a loop to "deal" 100,000 5-card hands from the Carddeck object and use a dictionary to count the frequency of occurrence of each of the 9 possible poker hand rankings
c. Display the resulting counts using the hand "names" (strings) in order of least frequent to most frequent (see example)
Example:
Straight Flush : 8
Four of a kind : 33
Full house : 163
Flush : 206
Straight : 407
Three of a kind : 2244
Two pair : 4850
One pair : 42270
High card : 49819
Step by Step Solution
There are 3 Steps involved in it
Step: 1
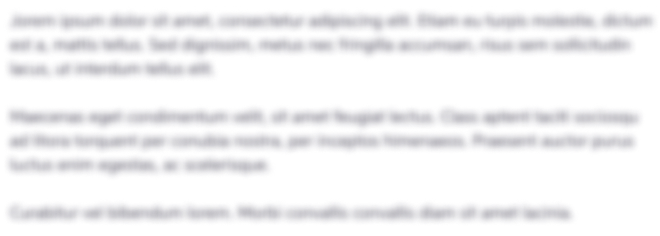
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started