Question
PYTHON PROGRAMMING . def make_class(attrs, base=None): def make_instance(cls): def bind(val, instance): if callable(val): def method(*args): return val(instance, *args) return method return val def get_v(attr_name): if
PYTHON PROGRAMMING
.
def make_class(attrs, base=None): def make_instance(cls): def bind(val, instance): if callable(val): def method(*args): return val(instance, *args)
return method return val
def get_v(attr_name): if attr_name in attrs: return attrs[attr_name]
val = cls['get'](attr_name) return bind(val, instance)
def set_v(attr_name, val): attrs[attr_name] = val # FIXME: Edit set_protected to set attr_name as protected. # This is the only "helping" stub you are given. # You are on your own now. def set_protected(attr_name): pass
# TIP: One of the possible solutions is to create or use another # instance dictionary that has the protected attributes # and somehow inject it via the bind() call in the get() # method. This means that you may need to add or amend more # stuff than the ones given in this function. # # Feel free to change this make_instance() function in any way # as long as the dictionary returned by this function has # the three methods get(), set(), and set_protected(). attrs = {} instance = { 'get': get_v, 'set': set_v, 'set_protected': set_protected, }
return instance
# PS. Please do not edit anything below this comment to make your life # easier. :) def init(cls, *args): obj = make_instance(cls) init_m = cls['get']('__init__')
if init_m: init_m(obj, *args)
return obj
def get_a(attr_name): if attr_name == 'super': return base elif attr_name in attrs: return attrs[attr_name] elif base is not None: return base['get'](attr_name) else: return None
def set_a(attr_name, val): attrs[attr_name] = val
def new_o(*args): return init(cls, *args)
cls = { 'get': get_a, 'set': set_a, 'new': new_o, }
return cls
def main(): print('hooray!')
if __name__ == '__main__': main()
Composing Programs has introduced us to a way to implement an OOP system in programming languages that don't have it. In "Challenge" 11A, we have augmented this system to incorporate object equality. This time, we will be taking it a notch further and implement basic information hiding. Python inherently doesn't support access modifiers, but you can make a class property "private" by appending two underscores in the name, like --print. This name mangling enables these properties to be used unambiguously in case two or more classes have the exact same property name. Now, we are interested in making object properties protected, like the ones delegated to self. A property is protected if it can only be accessed within the class definition of both the class that defines it and its subclasses, and not outside of it. This is in contrast with a public property, which can be accessed from anywhere, and a private property, which can be accessed only by the class itself. To emulate protected properties, we will edit the dictionary returned by the make_instance() function to have a set_protected (attr_name) method. This method will set attr_name to protected if it exists, and creates a new attribute with that name and value None otherwise. If a protected property is accessed from the outside, None will be returned. Setting a protected property from the outside will also be ignored. Note that there shall be no case where the same attribute name has a public and protected attribute as attribute names should be unique in each class or object. Also, when a subclass inherits from a class with protected properties, those properties will be "copied" (i.e. visible) to the subclass. Here is a code snippet for a sample usage of the amended OOP system. def SampleClassMaker: def __init__(self): self['set']('a', 'hello') self['set']('b', 'world') # b has been converted from public to # protected self['set_protected'] (6) def get_b (self): # within class def! # bis accessible here return self['get'l('6') def set_b(self, val): # within class def! # bis accessible here return self ['set']('b', val) return make_class (locals() = Sample = SampleClassMaker() sample_obj = Sample['new'] # outside class def! #bis NOT accessible here sample_obj['get'l('a') # 'hello' sample_obj['get'] ('b') # None sample_obj['get']('set_b') # 'world! sample_obj['set'l('b', 'earth') sample_obj['get' ('get_b'> 0 # 'world! sample_obj['get'l('set_b) ('earth') sample_objl'get']('set_b') # 'earth! Input Format This "challenge" checks your code by parsing it, and that the testcases are just placeholders. For sanity check, the input will always be the string hip hip!. Constraints Input Constraints None Functional Constraints You are required to create a function named set_protected (attr_name) inside the make_instance() function, and for all objects to have a set_protected() method. You are also not allowed to have any class keywords in your code. Failure to follow these functional constraints will mark your code with a score of zero. Output Format The output of the program should always be hooray!. Sample Input o hip hip: Sample Output 0 hooray! Explanation 0 This test runs the Sample class snippet in the problem statement 47 3 def make_class(attrs, base=None): def make_instance (cls): 5 def bind (val, instance): 6 if callable(val): 7 def method(*args) : 8 return val(instance, *args) 9 10 return method 11 12 return val 13 14 def get_v(attr_name): 15 if attr_name in attrs: 16 return attrs[attr_name] 17 18 val = cls['get'] (attr_name) 19 return bind (val, instance) 20 21 def set_v(attr_name, val): 22 attrs[attr_name] = val 23 24 # FIXME: Edit set_protected to set attr_name as protected. 25 This is the only "helping" stub you are given. 26 You are on your own now. 27 def set_protected (attr_name): 28 pass 29 30 # TIP: One of the possible solutions is to create or use another 31 instance dictionary that has the protected attributes 32 and somehow inject it via the bind call in the get 33 method. This means that you may need to add or amend more 34 stuff than the ones given in this function. 35 36 Feel free to change this make_instance() function in any way 37 as long as the dictionary returned by this function has 38 the three methods get(), set(), and set_protected(). # # ######## 03 = attrs = instance { 'get': get_V, 'set': set_V, set_protected': set_protected, } return instance 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 # PS. Please do not edit anything below this comment to make your life easier. :) def init(cls, *args) : obj = make_instance (cls) init_m = cls['get'](__init__') if init_m: init_m(obj, *args) return obj 62 def get_a(attr_name): if attr_name == 'super': return base elif attr_name in attrs: return attrs[attr_name] elif base is not None: return base['get'] (attr_name) else: return None 64 66 WY88988398 def set_a (attr_name, val): attrs[attr_name] = val 69 70 71 72 73 def new_o(*args) : return init(cls, *args) 74 75 cls = { 76 'get': get_a, 77 'set': set_a, 78 new': new_o, 79 } 80 81 return cls 82 83 def main(): 84 print("hooray!' 85 86 if -_name__ == '__main__': 87 main()Step by Step Solution
There are 3 Steps involved in it
Step: 1
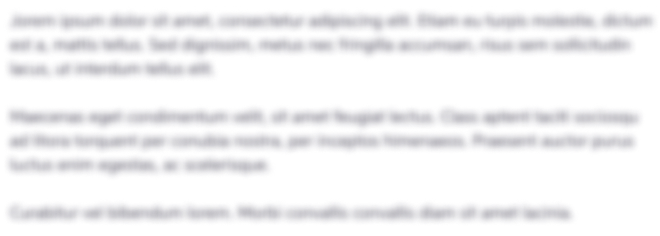
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started