Question
PYTHON PROGRAMMING. (**Only need Part B**) This assignment focuses on the design, implementation and testing of Python programs to process data files, as described below.
PYTHON PROGRAMMING. (**Only need Part B**)
This assignment focuses on the design, implementation and testing of Python programs to process data files, as described below.
Assignment Specifications
1. The World Health Organization (WHO) compiles data about immunization levels around the world. The file namedmeasles.txt contains data about the level of measles vaccinations in various countries over time.
measles.txt
Each line of the file contains the following fields, where there is one space between fields:
Country (50 characters)
Income Level (6 characters)
Percent Vaccinated (3 characters)
Region (25 characters)
Year (4 characters)
The Country field contains the name of the country.
The Income Level field identifies the category assigned to that country by the World Bank:
WB_LI low income
WB_LMI lower middle income
WB_UMI upper middle income
WB_HI high income
The Percent Vaccinated field contains an integer number representing the percentage of children in that country who have received measles vaccine by the age of one.
The Region field identifies the region assigned to that country by WHO.
The Year field contains the year for which the data was compiled.
Part A (Part A is completed. I have the code below)
1. The program in lastname_firstname_7a.pywill copy selected lines from measles.txt into a file selected by the user.
a) The program will always read from measles.txt (it will not prompt the user for the name of the input file). If it is unable to open that file, the program will halt.
b) The program will prompt the user for the name of the output file. If that file does not exist, the program will create it and continue. If that file does exist, the program will discard the current contents of the file and continue.
c) The program will prompt the user to enter a year, and will copy all lines of measles.txt selected by the users response. A line is selected if the users response matches the Year field or any of its prefixes. All lines are selected if the users response is any of the values in the set {, all, ALL}. Note that is the empty string.
For example, a line whose Year field contains 1987 would be selected by any of the following user responses: {1, 19, 198, 1987, , all, ALL}.
2. The output file created by the program will have the same format as the input file (same field widths and spacing). Note that when the user selects all lines, the output file will be identical to the input file.
3. The program will display appropriate messages to inform the user about any unusual circumstances.
Part B
1. The program in lastname_firstname_7b.py will display one summary report to the user.
a) The program will prompt the user to enter the name of the input file. If it is unable to open that file, the program will prompt the user again until the user enters a valid file name.
b) The program will prompt the user to enter a year, and will then prompt the user to enter an income level. The income level must be one of the characters in the set {1, 2, 3, 4}, where 1 corresponds to low income, 2 corresponds to lower middle income, 3 corresponds to upper middle income and 4 corresponds to high income.
c) The program will identify all records (lines) in the input file which match the users criteria for year and income level, and the program will display a report with the following information:
The count of records in the input file which match the users criteria
The average percentage for those records (displayed with one fractional digit)
The country with the lowest percentage for those records
The country with the highest percentage for those records
The name of the country and the percent of children vaccinated will be displayed for the last two items (lowest percentage and highest percentage).
2. The program will display appropriate messages to inform the user about any unusual circumstances.
3. The program will contain the following functions (you may develop additional functions):
open_file() -> file object
process_file( file object ) -> None
main() -> None
The notation above gives the name of each function, the number and type of its argument(s), and the type of its return value.
a) The function names will be spelled exactly as shown (for example, open_file).
b) Function open_file has no parameters. It returns a file object after prompting the user to enter the name of the input file (see above).
c) Function process_file has one parameter (a file object). It performs the processing to read the input file and display the report.
d) Function main has no parameters. It invokes the other two functions and then closes the file object.
Assignment Notes
1. The data in measles.txt is from the World Health Organizations website and is used with permission:
http://apps.who.int/gho/data/node.main.A824?lang=en (Links to an external site.)Links to an external site.
2. Documentation about the built-in function open is on the Python website:
http://docs.python.org/3/library/functions.html#open (Links to an external site.)Links to an external site.
In particular, the information about modes r, w and x may be useful.
3. A simple approach to determining the maximal value in a set of values is described below.
Initialize the current maximal value to something smaller than all valid values
For each value in the set:
If the value is larger than the current maximal value, save it as the current maximal value
A similar procedure can be used to determine the minimal value in a set of values.
4. When performing file I/O its very inefficient to constantly open and close the files youre working with. Try to avoid putting the open and close in loops unless you cant figure any other way to do it.
Here is an example of the .txt:
Angola WB_LMI 0 Africa 1981
Angola WB_LMI 0 Africa 1982
Angola WB_LMI 26 Africa 1983
Angola WB_LMI 35 Africa 1984
Angola WB_LMI 44 Africa 1985
Angola WB_LMI 44 Africa 1986
Angola WB_LMI 55 Africa 1987
Angola WB_LMI 56 Africa 1988
Angola WB_LMI 48 Africa 1989
Angola WB_LMI 38 Africa 1990
Angola WB_LMI 39 Africa 1991
Angola WB_LMI 39 Africa 1992
Angola WB_LMI 47 Africa 1993
Angola WB_LMI 44 Africa 1994
Angola WB_LMI 46 Africa 1995
Angola WB_LMI 62 Africa 1996
Angola WB_LMI 78 Africa 1997
Angola WB_LMI 62 Africa 1998
Angola WB_LMI 46 Africa 1999
-----------------------------------------------------------------------------------------------------------------
Part A:
year=input('Enter year:') l=[] try: with open('measles.txt', 'r') as f: for lines in f: line = lines.split() if line: if year in ["ALL", 'all', '']: l.append(lines) elif line[4].startswith(year): l.append(lines) except IOError: print('Could not read file') if l: file=input("Enter name of file in which data will be written: ") with open(file,'w') as f: for lines in l: f.write(lines) else: print('No data to write')
Step by Step Solution
There are 3 Steps involved in it
Step: 1
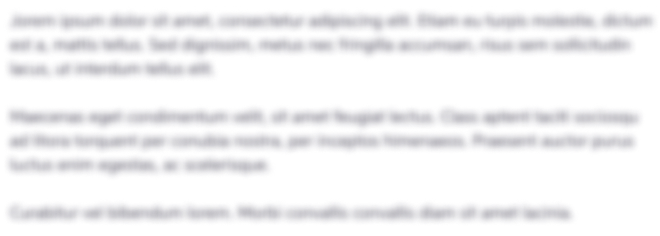
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started