Question
Python programming. You will create a Python library module that will contain three constants and eight function definitions. The functions focus on string manipulation. a)
Python programming.
You will create a Python library module that will contain three constants and eight function definitions. The functions focus on string manipulation.
a) It will include the following constants:
ASCII_LOWERCASE = "abcdefghijklmnopqrstuvwxyz"
ASCII_UPPERCASE = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
DECIMAL_DIGITS = "0123456789"
b) It will include the following functions:
is_alpha( str ) -> bool
Takes one parameter (a string). It returns True if all of the characters in the string are upper case or lower case ASCII letters (it returns False otherwise).
is_digit( str) -> bool
Takes one parameter (a string). It returns True if all of the characters in the string are ASCII decimal digits (it returns False otherwise).
to_lower( str ) -> str
Takes one parameter (a string). It returns the string which is a copy of the parameter, but where all of the upper case ASCII letters have been converted to lower case ASCII letters.
to_upper( str ) -> str
Takes one parameter (a string). It returns the string which is a copy of the parameter, but where all of the lower case ASCII letters have been converted to upper case ASCII letters.
find_chr( str, str ) -> int
Takes two parameters (both strings), where the second parameter must be of length 1. It returns the lowest index where the second parameter is found within the first parameter (it returns -1 if the second parameter is not of length 1 or is not found within the first parameter).
find_str( str, str ) ->int
Takes two parameters (both strings). It returns the lowest index where the second parameter is found within the first parameter (it returns -1 if the second parameter is not found within the first parameter).
replace_chr( str, str, str ) -> str
Takes three parameters (all strings), where the second and third parameters must be of length 1. It returns the string which is a copy of the first parameter, but where all occurrences of the second parameter have been replaced by the third parameter (it returns the empty string if the second or third parameter are not of length 1).
replace_str( str, str, str ) -> str
Takes three parameters (all strings). It returns the string which is a copy of the first parameter, but where all occurrences of the second parameter have been replaced by the third parameter. If there are no occurrences of the second parameter in the first, it returns a copy of the first parameter. If the second parameter is the empty string, it returns the string which is a copy of the first parameter, but with the third parameter inserted before the first character, between each character, and after the last character.
Notes:
a) The function names must be spelled exactly as shown (for example, is_digit).
b) The library module will not use any of the string methods listed in Section 4.7.1 of the Python Standard Library:
http://docs.python.org/3.3/library/stdtypes.html#string-methods
c) You are free to use the any of the functions or operators from the built-in library. The following may be useful when you implement your library module:
ord( str ) int # return code of ASCII character
chr( int ) str # return ASCII character of code
Slice operator [n:m] # returns the part of the string from the nth character to the mth character
In operator x in s # Returns true if x is a member of s
d) The library module will not contain any import statements.
e) The library module will not perform any input or output operations. For example, it will not call function input or function print.
f) Be sure to check the validity of the data being passed in. If the parameter has a restriction on it, be sure you let the user know they provided bad data.
Example usage and output:
>>> is_alpha(a)
True
>>> is_digit(a)
False
>>> to_lower(Jason %*&(* IS AWESOME)
jason %*&(* is awesome
>>> to_upper(Jason %*&(* IS AWESOME)
JASON %*&(* IS AWESOME
>>> find_chr(Hello, l)
2
>>> find_str(My name is Jason, x)
-1
>>> replace_chr(My name is Jason, s, x)
'My name ix Jaxon'
>>> replace_str(My name was Jason, as ill)
'My name will Jillon'
Step by Step Solution
There are 3 Steps involved in it
Step: 1
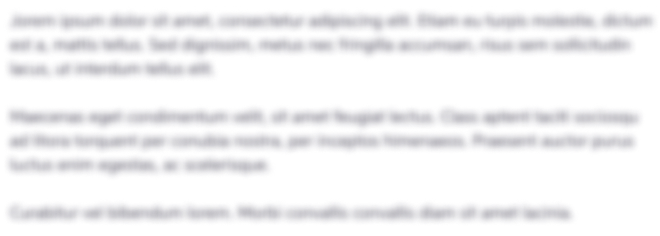
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started