Question
Python Project 3 In this project, you will practice how to use tuples as well as lists in Python by developing a shopping cart application.
Python Project 3
In this project, you will practice how to use tuples as well as lists in Python by developing a shopping cart application. You will read a file that contains a list of groceries along with their prices. You will then display the list to the user. You will then ask the user to pick some items from the list and enter the quantity for each item. Finally, you will print a receipt for this shopping session.
Getting Started
This can be divided into four major chunks:
Reading the Grocery List
In your program, you will open a file named grocerydb.txt. This file is in the following format:
Item1Name,pricePerUnit Item2Name,pricePerUnit . . .
For example:
Milk,5.97 Eggs,8.94 Ice Cream,4.45 Corn Flakes,3.89 Orange Juice,3.56 . . .
This format is known as the CSV (comma-separated values) format. For this project open the file with the open() function and using a for loop, read the file line by line and split each line into two components using the str.split(sep=",") function. Here is an example: line.split(sep=",")
Storing Items in a List
First, create an empty list named inventory. For each line you read from the file, create a new tuple. The first element of the tuple is the name of the grocery item and the second element is the price. For example, for the first line shown in the example input file the tuple you create would be ('Milk', 5.97). Then you insert this tuple into the inventory list.
Displaying the List to the User and Ask for Selections
Now that you have the grocery list stored in a list in your program, you should display this list to the user. You also have to display a serial number with each item. Remember: the serial number printed for an item must be the same as the index of that item in the inventory list. For example, if the user enters 2 as their choice, then inventory[2] == ('Ice Cream', 4.45).
After printing the list, you have to ask the user to select a few items. Create an empty list named selectedItems. Prompt the user for the serial number of a single item, and then prompt them for the quantity of the item. Create a tuple with the serial number as the first element and quantity as the second element. Add this tuple to the selectedItems list.
Finally, ask the user if they want to add another item. If the user replies y or yes, prompt for another items serial number and quantity and store it in the selectedItems list as described above. This must be repeated using a loop until the user replies n or no.
Printing the Final Receipt
For each tuple in the selectedItems list, use the first element as the index to get the corresponding item tuple from the inventory list. (Now you realize the benefit of the printed serial number and the list index being equal!) Calculate the charge for a single item from the price and the quantity, and print the item in the following format:
Milk $5.94 2 $11.94 Orange Juice $8.94 1 $8.94
The item name is left aligned in a 15-character field. The unit price is right-aligned in 10-character field. The quantity is right-aligned in 4-character field. The charge for each item is also right-aligned in 10-character field.
You also have to sum the charge for each item to calculate a total for the receipt. Then print the total for the receipt in the following format:
-------------------------------------- Total: $20.88 --------------------------------------
Example Input/Output Session:
Welcome to Shopping Cart! Today you can buy: 0 Milk 5.97 1 Eggs 8.94 2 Ice Cream 4.45 3 Corn Flakes 3.89 4 Orange Juice 3.56 Enter the serial number of the item to place in your cart: 0 Enter the quantity for the this item: 2
Do you want to select another item (y/n)? y
Enter the serial number of the item to place in your cart: 4 Enter the quantity for the this item: 1
Do you want to select another item (y/n)? n
Here is the receipt for your shopping cart: -------------------------------------- Milk $5.94 2 $11.94 Orange Juice $8.94 1 $8.94
-------------------------------------- Total: $20.88 -------------------------------------- Goodbye, have a nice day!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
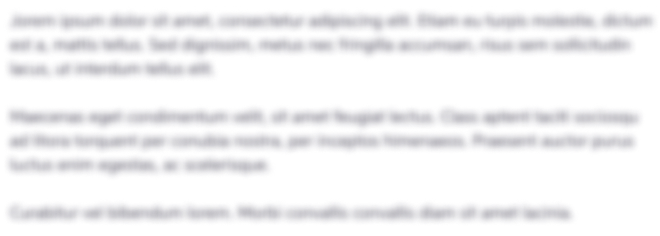
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started