Question
Python: Regular Expression:( I need help writing regular expression for exercise 2,3,5,6,7,8 similar to exercise 1 ) Assume using Greedy Quantifiers You may not use
Python: Regular Expression:( I need help writing regular expression for exercise 2,3,5,6,7,8 similar to exercise 1 ) Assume using Greedy Quantifiers
You may not use any additional if-statements, you must accomplish all of the tasks through the use of regular expressions.
Exercise 1: Validate Simple Zip Codes
Determine if the user's input could be a zip code or not. For this first exercise, we'll keep it simple, just make sure that the input has 5 digits.
Exercise 2: Extended Zip Codes
Determine if the zip code is valid. Zip code rules:
- Zip codes are formatted as either XXXXX or XXXXX-XXXX
Exercise 3: Numerically Correct Zip Codes
Determine if the zip code is valid. Zip code rules
- Zip codes are formatted as either XXXXX or XXXXX-XXXX
- Zip codes may start with a 0, but then the second digit must be a non-zero digit
- Zip codes may start with any non-zero digit and the second digit may be any digit
Exercise 4: Variable Name Validation
Determine valid python variable names. You already know the rules for these....
Exercise 5: Username Validation
Determine if the username is valid. Username rules:
- May be any number of characters
- Must start with an alphabetical character
- May have any number of "word" characters after the first character (alphabetical, numerical, underscore)
- May have periods, but cannot have more than one period in a row
- Usernames may not end in a period
Exercise 6: Min/Max Length Username Validation
Determine if the username is valid. Username rules:
- Must be at least 6 characters and no more than 16 characters
- Must start with an alphabetical character
- May have only "word" characters after the first character
Exercise 7: Email Validator
Determine if the email is valid. Email rules:
- Must start with an alphabetical character
- May have "word" or period characters after the first character
- Must have a "word" character after any period character
- May not have two periods in a row
- Must have an "@" somewhere after the first character
- Must have a "word" character preceding the @
- Must have a "word" character immediately following the @
- Must have at least one period after the @
- Must end with 2-4 "word" characters
Exercise 8: Separate City, State and Zip
Separate the City, State, and Zip from the line. DO NOT print whether the city/state/zip were valid, just pull each one out of the input using a regex.
City rules:
- Words in cities start with an uppercase letter followed by lowercase letters
- Parts of cities may be abbreviated with at least one character (W., St., Ste.)
- Cities may have one or more words
- Cities are separated from their state by a comma and at least one space
State rules:
- States are abbreviated with exactly 2 characters
- States are separated from their zip by at least one space
Zip rules:
- Zip codes may be formatted as XXXXX or XXXXX-XXXX
- Zip codes may start with a 0 but then the second digit cannot be a zero
Print the City, State and Zip:
To accomplish this, you might need to use named groups! Check this out: http://www.learningaboutelectronics.com/Articles/Named-groups-with-regular-expressions-in-Python.php
print("City: " + ### city ###) print("State: " + ### state ###) print("Zip: " + ### zip ###)
My Code:
################################################################### # Main ###################################################################
import re
exercise = -1 while (exercise != 0): exercise = int(input("Which exercise would you like to test (1-8, 0 to exit)? "))
##################################################### # Exercise 1: Validate Simple Zip Codes ##################################################### if exercise == 1: zipcode = input("Enter a zip code: ") # TODO: put your regex here zipcodeRegex=re.compile(r'^\d{5}$') mo = zipcodeRegex.search(zipcode) if mo:# TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 2: Extended Zip Codes ##################################################### elif exercise == 2: zipcode = input("Enter a zip code: ") pattern = "([0-9]{5}\-[0-9]{4})|[0-9]{5}" # TODO: put your regex here if re.search(pattern,zipcode): # TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 3: Numerically Correct Zip Codes ##################################################### elif exercise == 3: zipcode = input("Enter a zip code: ") pattern = "^[0][1-9][0-9]{3}\-[0-9]{4}|^[1-9][0-9]{4}\-[0-9]{4}|^[0][1-9][0-9]{3}|^[1-9][0-9]{4}" # TODO: put your regex here if re.search(pattern,zipcode): # TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 4: Variable Name Validation ##################################################### elif exercise == 4: name = input("Enter a variable name: ") pattern = "^[^\d\W]\w*\Z" # TODO: put your regex here if re.search(pattern,name): # TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 5: Username Validation ##################################################### elif exercise == 5: username = input("Enter a username: ") pattern = "^[^\d\W](\w|[(? # TODO: put your regex here if re.search(pattern,username): # TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 6: Min/Max Length Username Validation ##################################################### elif exercise == 6: username = input("Enter a username: ") pattern = "^[a-zA-z]\w{5,15}" # TODO: put your regex here if re.search(pattern,username): # TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 7: Email Validator ##################################################### elif exercise == 7: email = input("Enter a email address: ") pattern = "(^[a-zA-Z][\w\.]*@\w+\.\w{2,4}$)" # TODO: put your regex here if re.search(pattern,email): # TODO: test your search here print("Valid") else: print("Invalid")
##################################################### # Exercise 8: Separate City, State and Zip ##################################################### elif exercise == 8: address = input("Enter a city, state and zip: ") # TODO: put your regex here # TODO: use the regex to pull of the city, state, and zip here pattern = re.compile("(^[A-Z][a-z.]*)[\s,]{1}([a-zA-Z]{2})[\s]{1}(^[0][1-9]{1}\d{3}|\d{5}\-\d{4}|\d{5})$") groups=pattern.match(address) if groups: print("City: %s " %groups.group(1)) print("State: %s" %groups.group(2)) print("Zip: %s" %groups.group(3))
##################################################### # Invalid choice ##################################################### elif exercise == 0: exit
else: print("Your response must be from 0 to 8, try again: ")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
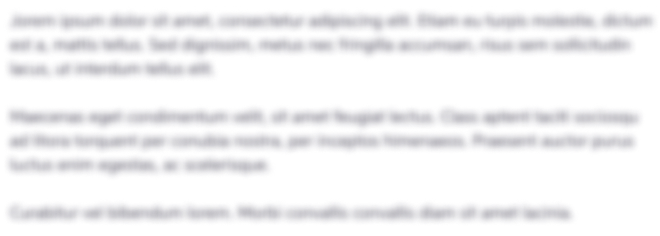
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started