Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Python Write a function square(a) that takes a list a of numbers and squares each of the numbers in the list. The function should not
Python
- Write a function square(a) that takes a list a of numbers and squares each of the numbers in the list. The function should not return a value (more specifically, it should return None).
- Write a function squared(a) that takes a list a of numbers and returns a new list that contains each value in a squared. The original list a should not be modified by your function.
- Write a function lowercase(a) that takes a list a of strings and replaces each string in a with a lowercase version of the string
- Write a function lowercased(a) that takes a list a of strings and returns a new list in that contains a lowercase version of each string in a
- Create a new class called Point that represents a point in the Cartesian plane with two coordinates.
- Each instance of Point should have to coordinates called x and y.
- The constructor for Point should take two arguments x and y that represent the two coordinates of the newly created point.
- Your class Point should override the __str__(self) method so that it returns a string showing the x- and y-coordinates of the Point enclosed in parentheses and separated by a comma.
- Your class Point should override the __repr__(self) method so that it returns a string that contains Python code that would produce an instance of the same point.
- Your class Point should override the __eq__(self, other) method so that it returns True if and only if other is also a Point that has the same x- and y- coordinate as self.
- Write a function distance(p, q) that takes two Points p and q and returns the Euclidean distance between p and q.
- Write a function double(p) that modifies p so that the values of its x- and y-coordinates are doubled.
- Write a function doubled(p) that returns a new Point whose x- and y-coordinates are twice those of p
Sample Output
>>> a = list(range(1, 6)) >>> a [1, 2, 3, 4, 5] >>> square(a) >>> a [1, 4, 9, 16, 25] >>> >>> >>> b = list(range(1,6)) >>> squared(b) [1, 4, 9, 16, 25] >>> b [1, 2, 3, 4, 5] >>> c = squared(b) >>> c [1, 4, 9, 16, 25] >>> b [1, 2, 3, 4, 5] >>> >>> >>> a = ["Kate", "and", "Allie", "spoke", "LOUDLY"] >>> a ['Kate', 'and', 'Allie', 'spoke', 'LOUDLY'] >>> lowercase(a) >>> a ['kate', 'and', 'allie', 'spoke', 'loudly'] >>> >>> >>> b = ["Kate", "and", "Allie", "spoke", "LOUDLY"] >>> b ['Kate', 'and', 'Allie', 'spoke', 'LOUDLY'] >>> lowercased(b) ['kate', 'and', 'allie', 'spoke', 'loudly'] >>> b ['Kate', 'and', 'Allie', 'spoke', 'LOUDLY'] >>> >>> c = lowercased(b) >>> c ['kate', 'and', 'allie', 'spoke', 'loudly'] >>> b ['Kate', 'and', 'Allie', 'spoke', 'LOUDLY'] >>> >>> p = Point(3,4) >>> p Point(3,4) >>> >>> str(p) '(3,4)' >>> >>> print("p = {}".format(p)) p = (3,4) >>> >>> >>> q = Point(3,4) >>> >>> >>> p Point(3,4) >>> q Point(3,4) >>> >>> >>> p == q True >>> >>> >>> p is q False >>> >>> >>> >>> >>> r = Point(3,7) >>> p Point(3,4) >>> r Point(3,7) >>> >>> p == r False >>> >>> >>> p Point(3,4) >>> q Point(3,4) >>> r Point(3,7) >>> >>> distance (p,q) 0.0 >>> >>> distance(p,r) 3.0 >>> >>> >>> p Point(3,4) >>> double(p) >>> p Point(6,8) >>> >>> >>> q Point(3,4) >>> doubled(q) Point(6,8) >>> q Point(3,4) >>> s = doubled(q) >>> >>> s Point(6,8) >>> q Point(3,4) >>> >>> quit()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
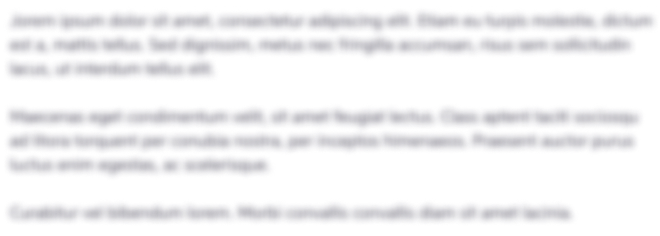
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started