Question
Q) We will create a factory pattern. The factory pattern is useful when you have to create objects based on user input or when you
Q) We will create a factory pattern. The factory pattern is useful when you have to create objects based on user input or when you need to defer the instantiation of objects to subclasses. An object is created by calling a factory method. The factory method uses an abstract class for the superclass. Any subclasses extend the super class. Below you will have an example of a Factory Design Pattern. This is one of the most popular design patterns used in industry today. Use this example to create your own! It can be a factory of colors, shapes, animals, vehicles, toys, food, products, employees, etc. Anything you want!
Include ALL of your classes (you should have 5 at the minimum), and a screenshot of the output.
Below is an example using an abstract phone class as the superclass. The two subclasses are iphone and android. The phonefactory class provides the instantiation of the class. The factory is the main class. Notice that the Samsung and iphonex variables are of type Phone).
Phone.java this is the super class and it is abstract
public abstract class Phone {
//The abstract methods in Phone must be implemented in the subclasses
public abstract String getRAM();
public abstract String getStorage();
public abstract String getSize();
public abstract void slogan();
@Override
public String toString(){
return "RAM= "+this.getRAM()+", Storage="+this.getStorage()+", Size="+this.getSize();
}
}
Android.java subclass 1
public class Android extends Phone{
private String ram;
private String storage;
private String size;
public Android(String r, String s, String sz){
ram=r;
storage=s;
size=sz;
}
@Override
public String getRAM() {
return this.ram;
}
@Override
public String getStorage() {
return this.storage;
}
@Override
public String getSize() {
return this.size;
}
public void slogan()
{
System.out.println("Be together, not the same");
}
}
iPhone.java subclass 2
public class iPhone extends Phone {
private String ram;
private String storage;
private String size;
public iPhone(String r, String s, String sz){
ram=r;
storage=s;
size=sz;
}
@Override
public String getRAM() {
return this.ram;
}
@Override
public String getStorage() {
return this.storage;
}
@Override
public String getSize() {
return this.size;
}
public void slogan()
{
System.out.println("Think different");
}
}
PhoneFactory factory class that instantiates the object requested
public class PhoneFactory {
public Phone getPhone(String PhoneType, String r, String s, String sz)
{
//Create object here
if(PhoneType.equalsIgnoreCase("ANDROID"))
return new Android(r, s, sz);
else if(PhoneType.equalsIgnoreCase("IPHONE"))
return new iPhone(r, s, sz);
else
return null;
}
}
Main Factory.java
public class Factory {
public static void main(String[] args) {
PhoneFactory pf = new PhoneFactory();
Phone samsung= pf.getPhone("android","6 GB","64 GB","6.0 inches");
Phone iphonex= pf.getPhone("iphone","3 GB","64 GB","5.8 inches");
System.out.println("Android Configuration:"+samsung);
samsung.slogan();
System.out.println("iPhone Configuration:"+iphonex);
iphonex.slogan();
}
}
Results of the above code:
Android Configuration:RAM= 6 GB, Storage=64 GB, Size=6.0 inches
Be together, not the same
iPhone Configuration:RAM= 3 GB, Storage=64 GB, Size=5.8 inches
Think different
BUILD SUCCESSFUL (total time: 0 seconds)
Your code:
Screenshot showing it working:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
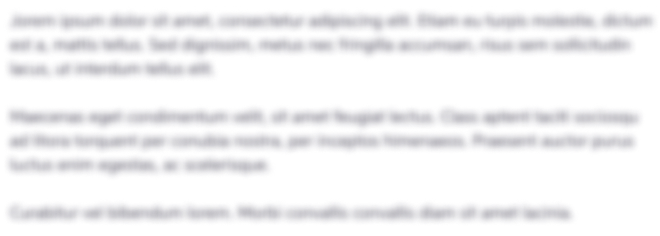
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started