Question
Q: You are given a string S, and you have to find all the amazing substrings of S. Amazing Substring is one that starts with
Q:
You are given a string S, and you have to find all the amazing substrings of S.
Amazing Substring is one that starts with a vowel (a, e, i, o, u, A, E, I, O, U).
Input
Only argument given is string S.
Output
Return a single integer X mod 10003, here X is number of Amazing Substrings in given string.
Constraints
1 <= length(S) <= 1e6
S can have special characters
Example
Input
ABEC
Output
6
Explanation
Amazing substrings of given string are :
1. A
2. AB
3. ABE
4. ABEC
5. E
6. EC
here number of substrings are 6 and 6 % 10003 = 6.
Python code for above cp question and mcq questions explanation is must. code will be tested on large test cases
1. What will be the output of print(math.factorial(4.5))?
a) 24
b) 120
c) error
d) 24.0
2. What will be the output of the following Python code snippet?
numbers = {}
letters = {}
comb = {}
numbers[1] = 56
numbers[3] = 7
letters[4] = 'B'
comb['Numbers'] = numbers
comb['Letters'] = letters
print(comb)
a) Error, dictionary in a dictionary can't exist
b) 'Numbers': {1: 56, 3: 7}
c) {'Numbers': {1: 56}, 'Letters': {4: 'B'}}
d) {'Numbers': {1: 56, 3: 7}, 'Letters': {4: 'B'}}
3. What will be the output of the following Python code?
class A:
def init(self):
self.x = 1
class B(A):
def display(self):
print(self.__x)
def main():
obj = B()
obj.display()
main()
a) 1
b) 0
c) Error, invalid syntax for object declaration
d) Error, private class member can't be accessed in a subclass
4. The function pow(x,y,z) is evaluated as:
a) (x**y)**z
b) (x**y) / z
c) (x**y) % z
d) (x**y)*z
5. What will be the output of the following Python code?
class Test:
def __init(self):
self.x = 0
class Derived_Test(Test):
def init(self):
self.y = 1
def main():
b = Derived_Test()
print(b.x,b.y)
main()
a) 0 1
b) 0 0
c) Error because class B inherits A but variable x isn't inherited
d) Error because when object is created, argument must be passed like Derived_Test(1)
6. The following Python code will result in an error.
import turtle
t=turtle.Pen()
t.speed(-45)
t.circle(30)
a) True
b) False
7. What is the use of seek() method in files?
a) sets the file's current position at the offset
b) sets the file's previous position at the offset
c) sets the file's current position within the file
d) none of the mentioned
8. Which operator is overloaded by the or() function?
a) ||
b) |
c) //
d) /
9. What will be the output of the following Python code?
class Test:
def init(self):
self.x = 0
class Derived_Test(Test):
def init(self):
Test.init(self)
self.y = 1
def main():
b = Derived_Test()
print(b.x,b.y)
main()
a) Error because class B inherits A but variable x isn't inherited
b) 0 0
c) 0 1
d) Error, the syntax of the invoking method is wrong
10. What will be the output of the following Python code?
re.split('mum', 'mumbai*', 1)
a) Error
b) [", 'bai*']
c) [", 'bai']
d) ['bai*']
11. What will be the output of the following Python code, if the code is run on Windows operating system?
import sys
if sys.platform[:2]== 'wi':
print("Hello")
a) Error
b) Hello
c) No output
d) Junk value
12. What is the use of tell() method in python?
a) tells you the current position within the file
b) tells you the end position within the file
c) tells you the file is opened or not
d) none of the mentioned
13. What will be the output of the following Python code?
a={}
a[2]=1
a[1]=[2,3,4]
print(a[1][1])
a) [2,3,4]
b) 3
c) 2
d) An exception is thrown
14. What will be the output of the following Python code?
x = 'abcd'
print(list(map(list, x)))
a) ['a', 'b', 'c', 'd']
b) ['abcd']
c) [['a'], ['b'], ['c'], ['d']]
d) none of the mentioned
15. Which of the following statements are true?
a) When you open a file for reading, if the file does not exist, an error occurs
b) When you open a file for writing, if the file does not exist, a new file is created
c) When you open a file for writing, if the file exists, the existing file is overwritten with the new file
d) All of the mentioned
16. What will be the output of the following Python code?
class test:
def init(self,a="Hello World"):
self.a=a
def display(self):
print(self.a)
obj=test()
obj.display()
a) The program has an error because constructor can't have default arguments
b) Nothing is displayed
c) "Hello World" is displayed
d) The program has an error display function doesn't have parameters
17. What will be the output of the following Python code?
import sys
sys.argv
a) ' '
b) [ ]
c) [' ']
d) Error
18. What is the type of sys.argv?
a) set
b) list
c) tuple
d) string
19. Is the following Python code valid?
try:
# Do something
except:
# Do something
else:
# Do something
a) no, there is no such thing as else
b) no, else cannot be used with except
c) no, else must come before except
d) yes
20. What will be the output of the following Python code?
def change(one, *two):
print(type(two))
change(1,2,3,4)
a) Integer
b) Tuple
c) Dictionary
d) An exception is thrown
21. The output of the following Python code is either 1 or 2.
import random
random.randint(1,2)
a) True
b) False
22. Which of the following functions does not accept any argument?
a) re.purge
b) re.compile
c) re.findall
d) re.match
23. Which of the following mode will refer to binary data?
a) r
b) w
c) +
d) b
24. What will be the output of the following Python code?
x = ['ab', 'cd']
print(len(map(list, x)))
a) [2, 2]
b) 2
c) 4
d) none of the mentioned
25. What will be the output of the following Python code?
def san(x):
print(x+1)
x=-2
x=4
san(12)
a) 13
b) 10
c) 2
d) 5
26. What will be the output of the following Python code?
a={1:"A",2:"B",3:"C"}
for i in a:
print(i,end=" ")
a) 1 2 3
b) 'A' 'B' 'C'
c) 1 'A' 2 'B' 3 'C'
d) Error, it should be: for i in a.items():
27. If a is a dictionary with some key-value pairs, what does a.popitem() do?
a) Removes an arbitrary element
b) Removes all the key-value pairs
c) Removes the key-value pair for the key given as an argument
d) Invalid method for dictionary
Step by Step Solution
There are 3 Steps involved in it
Step: 1
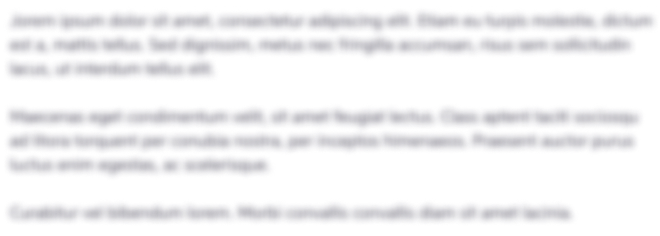
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started