Question
q1.c 1 #include 2 3 // Struct declaration 4 struct Node { 5 double data; 6 struct Node* left; 7 struct Node* right; 8 };
q1.c
1 #include
data1.txt 20 8 22 4 12 10 14
data2.txt
127, 234, 127, 654, 355, 789, 355, 355, 999, 654
data3.txt
44 31 83 95 18 100 22 58 36 54 24 58 98 95 94 27 53 86 56 71 34 71 88 19 26 46 53 89 72 57 37 34 61 75 25 34 19 55 100 30 70 32 41 26 79 52 75 9 4 39 67 91 71 5 19 39 4 98 61 39 42 96 3 8 63 74 84 95 28 62 54 62 61 1 5 6 33 58 83 67 98 63 76 91 83 68 64 14 80 7 33 2 67 61 20 8 31 95 7 82
data4.txt
0.815 0.906 0.127 0.913 0.632 0.098 0.278 0.547 0.958 0.965 0.158 0.971 0.957 0.485 0.800 0.142 0.422 0.916 0.792 0.959 0.656 0.036 0.849 0.934 0.679 0.758 0.743 0.392 0.655 0.171 0.706 0.032 0.277 0.046 0.097 0.823 0.695 0.317 0.950 0.034 0.439 0.382 0.766 0.795 0.187 0.490 0.446 0.646 0.709 0.755
Problem 1. (40 points] A binary search tree (BST) is a binary tree with ordering among its children. A BST satisfies the following: - The left subtree nodes less than the node's key, i.e., (the root). - The right subtree nodes greater than the node's key, i.e., (the root). - The left and right subtree each must also be a BST. Unlike linear data structures (Array, Linked List, Queues, Stacks, etc) which have only one logical way to traverse them, trees can be traversed in different ways. Here is a list of depth first traversal methods: (a) Inorder (Left, Root, Right), (b) Preorder (Root, Left, Right), Postorder (Left, Right, Root). Write a C program that you implement BST representation using data provided in an input text file using the command line arguments. Check the provided BST node structure. Your program should read input file to form a BST, display BST nodes in Inorder and return kthe largest element(s) in the BST. You should run your code as follows: ./q1 data1.txt 5 ./q1 data.txt 5 8 11 (a) (10 Points) Write a C function(s) to read input data (unsorted) from an input data file to form a BST. Note that data can be assumed as 'double' type but the number of entries in each file is unknown, and hence to be read dynamically until EOF (End of File) is reached (b) (10 Points) Write a C function display the elements using inorder traversal method, i.e., in sorted order from minimum to maximum value (no duplicates). (c) (15 Points) Write a C function to find the kthe largest element in the BST, where k is also a variable to be read using command line arguments. Here is an algorithm. [- Find the reverse of inorder traversal of the binary search tree. (- Keep track of the count of nodes visited while traversing. - When the count of the nodes becomes equal to k value, return the node. (c) [5 Points] Complete the main() to process command line arguments, and print the outcomes as in sample runs, (make sure to match the format of your outputs with respect to precision). Zafers-MacBook-Pro:93 zafers ./bst data3.txt 2 3 5 8 14 1.00 2.00 3.00 4.00 5.00 6.00 7.00 8.00 9.00 14.00 18.00 19.00 Zafers-MacBook-Pro:q3 zafers /bst datal.txt 2 3 5 8 20.00 22.00 24.00 25.00 26.00 27.00 28.00 30.00 31.00 32.00 33. 4.00 8.00 10.00 12.00 14.00 20.00 22.00 @0 34.00 36.00 37.00 39.00 41.00 42.00 44.00 46.00 52.00 53.00 2-nd largest element is 20.00 54.00 55.00 56.00 57.00 58.00 61.00 62.00 63.00 64.00 67.00 68. 3-rd largest element is 14.00 00 70.00 71.00 72.00 74.00 75.00 76.00 79.00 80.00 82.00 83.00 5-th largest element is 10.00 84.00 86.00 88.00 89.00 91.00 94.00 95.00 96.00 98.00 100.00 Zafers-MacBook-Pro:q3 zafer$ ./bst data2.txt 2 3 5 8 2-nd largest element is 98.80 127.00 234.00 355.00 654.00 789.00 999.00 3-rd largest element is 96.00 2-nd largest element is 789.00 5-th largest element is 94.00 3-rd largest element is 654.00 8-th largest element is 88.00 5-th largest element is 234.00 14-th largest element is 79.00 Zafers-MacBook-Pro:q3 zafer$ ./bst data3.txt 2 3 5 8 Zafers-MacBook-Pro:q3 zafers ./bst data4.txt 2 3 5 8 14 22 1.80 2.80 3.80 4.00 5.00 6.00 7.00 8.00 9.00 14.00 18.00 19.00 0.03 0.03 0.04 0.05 0.10 0.10 0.13 0.14 0.16 0.17 0.19 0.28 0.2 20.00 22.00 24.00 25.00 26.00 27.00 28.00 30.00 31.00 32.00 33.8 0.32 0.38 0.39 0.42 0.44 0.45 0.48 0.49 0.55 0.63 0.65 0.66 O 00 34.00 36.00 37.00 39.00 41.00 42.00 44.00 46.00 52.00 53.00 .66 0.68 0.69 0.71 0.71 0.74 0.76 0.76 0.77 0.79 0.80 0.80 0.81 54.00 55.00 56.00 57.00 58.00 61.00 62.00 63.00 64.00 67.00 68. 0.82 0.85 0.91 0.91 0.92 0.93 0.95 0.96 0.96 0.96 0.96 0.97 80 70.00 71.00 72.00 74.00 75.00 76.00 79.00 80.00 82.00 83.00 2-nd largest element is 0.96 84.00 86.00 88.00 09.00 91.00 94.00 95.00 96.00 98.00 100.00 3-rd largest element is 0.96 2-nd largest element is 98.00 5-th largest element is 0.96 3-rd largest element is 96.00 8-th largest element is 0.92 5-th largest element is 94.00 14-th largest element is 0.80 8-th largest element is 88.00 22-th largest element is 0.71Step by Step Solution
There are 3 Steps involved in it
Step: 1
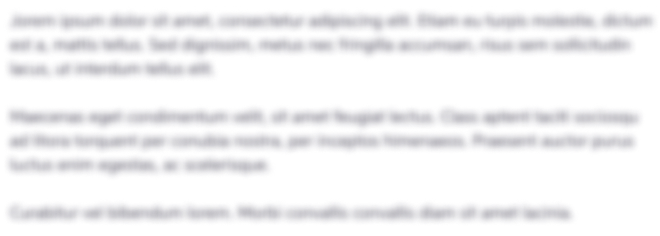
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started