Question
Q:Buffering and Structures Let me remind you of the expectations. Code should be neat, well documented. Variables should have meaningful names and be in a
Q:Buffering and Structures
Let me remind you of the expectations. Code should be neat, well documented. Variables should have meaningful names and be in a consistent format (I do not care if you use camelCase or under_scores in variables but be consistent. Maximum line length in your code is 100 characters. In addition, each file should have a standard header as defined below.
This assignment will give you experience using structures, pointers, character strings, enumerated types, bitmap fields, and buffering data into blocks. The files assignment2.h and assignment2.o are required for this assignment. The functions listed in assignment2.h (writePersonalInfo, getNext, commitBlock, and checkIt) have been written for you and are the functions in assignment2.o (you do not have the source code that builds that object file). Do not re-implement those functions.
There will be at least three arguments, but there may be more. Do not limit the number.
Step one - read the assignment carefully and fully. Do each step and don't read more into it then is there.
Step two of this assignment is to review the provided header file assignmet2.h. You will see a large number of #define statments the represent different computer lanaguages. Then there is a stucture called personalInfo. This is the structure you will allocate and populate. Next there is a #define of BLOCK_SIZE set to 256 this is going to be your buffer size to transform byte data into block data. Finally there are the prototypes for 4 functions that you will need and are described in the steps below. Note that the functions are implemented in provided assignment2.o file, you do not implement them.
Step three is to remember some of the rules. Name your c file according to our standard of lastname_firstname_HW1_main.c, edit the Makefile to enter your FIRST and LAST name (this Makefile is a little different then the first one - do not change anything other than the variables FIRSTNAME and LASTNAME). Make sure to comment your code, have the standard header. Remember that for every malloc, there must also be a corresponding free. Use printf's to debug your program. In keeping code neat and readable, lines in your program (including commments) should be kept to about 80 characters long but never more than 100 characters.
Now what is the coding portion of this assignment...
Step four is to allocate (using malloc) an instantiation of the personInfo structure and to populate it. The firstName and the lastName are populated from the 1st and second command line argument. You will then assign your student ID to the studentID field, you will populate the level (gradelevel) appropriately. You will then populate the languages field. To do so, specify every language you have Knowledge of and there must be at least three (by definition, with the prerequisites for the course you should at least know some Java, C++, some assembler and of course now C. But, include all you have knowledge of. The last part of populating the structure is to copy the third command line parameter to the message field. Do note the length of the message field.
Step five is to "write" your personal information structure by calling writePersonalInfo which is one of the function prototypes in the assignment2.h file. The return value from the function is 0 if it succeeds.
Step six involves getting a series of C stings (you do not know how many or how long each one it or what it contains). You get these strings by calling the function getNext. The return value is a char * (C string). If the return value is NULL then you have finished. You will copy the contents of each of those strings into a buffer (block) that is BLOCK_SIZE (use malloc to allocate the buffer), as the buffer is filled you will commit the buffer by calling commitBlock passing in the pointer to your BLOCK_SIZE buffer. Note: You must copy the data into the buffer in chunks using memcpy (not character by character).
Step seven if the final coding step. Call the function checkIt, then exit main returning the same value as returned from checkIt.
Step eight is dependent on checkIt running correctly and displays some binary data as a hexdump which is the personalInfo structure. You are to describe what each element is, use the structure as reference and show the values and how to read those values. i.e. which bytes are the student ID, what is it's hexadecimal value and if that is converted to decimal is it correct? You are to prove that each value in the structure is correct and why it is correct. The purpose is so that you can be sure that what you thought should be written is actually written. Use color highlights and start and end addresses to describe each element from the hexdump output.
Makefile code:
# File: Standard Makefile for CSC415 # # Description - This make file should be used for all your projects # It should be modified as needed for each homework # # ROOTNAME should be set you your lastname_firstname_HW. Except for # and group projects, this will not change throughout the semester # # HW should be set to the assignment number (i.e. 1, 2, 3, etc.) # # FOPTION can be set to blank (nothing) or to any thing starting with an # underscore (_). This is the suffix of your file name. # # With these three options above set your filename for your homework # assignment will look like: bierman_robert_HW1_main.c # # RUNOPTIONS can be set to default values you want passed into the program # this can also be overridden on the command line # # OBJ - You can append to this line for additional files necessary for # your program, but only when you have multiple files. Follow the convention # but hard code the suffix as needed. # # To Use the Makefile - Edit as above # then from the command line run: make # That command will build your program, and the program will be named the same # as your main c file without an extension. # # You can then execute from the command line: make run # This will actually run your program # # Using the command: make clean # will delete the executable and any object files in your directory. #
# Set your First name and your Last name # Ensure there no spaces before or after your name
FIRSTNAME= LASTNAME=
########################################################################## # Do NOT modify anything below this line #########################################################################
ROOTNAME=$(LASTNAME)_$(FIRSTNAME)_HW HW=2 FOPTION=_main # DO NOT CHANGE the RUNOPTIONS RUNOPTIONS=$(FIRSTNAME) $(LASTNAME) "Four score and seven years ago our fathers brought forth on this continent, a new nation, conceived in Liberty, and dedicated to the proposition that all men are created equal." $(TESTOPTIONS)
CC=gcc CFLAGS= -g -I. # To add libraries, add "-l
ifeq ($(ARCH), aarch64) ARCHOBJ=assignment2M1.o else ARCHOBJ=assignment2.o endif
OBJ = $(ROOTNAME)$(HW)$(FOPTION).o $(ARCHOBJ)
%.o: %.c $(DEPS) $(CC) -c -o $@ $< $(CFLAGS)
$(ROOTNAME)$(HW)$(FOPTION): $(OBJ) $(CC) -o $@ $^ $(CFLAGS) $(LIBS)
clean: rm $(ROOTNAME)$(HW)$(FOPTION).o $(ROOTNAME)$(HW)$(FOPTION)
run: $(ROOTNAME)$(HW)$(FOPTION) ./$(ROOTNAME)$(HW)$(FOPTION) $(RUNOPTIONS) $(TESTOPTIONS)
vrun: $(ROOTNAME)$(HW)$(FOPTION) valgrind ./$(ROOTNAME)$(HW)$(FOPTION) $(RUNOPTIONS) $(TESTOPTIONS)
assignment2.h code:
/************************************************************** * Class: CSC-415-0# Summer 2021 * Name: Robert Bierman * Student ID: * GitHub Name: bierman * Project: Assignment 2 Command Line Arguments * * File: assignment2.h * * Description: To show how to use ... * **************************************************************/ #ifndef ASSIGNMENT2_H #define ASSIGNMENT2_H 1
#ifdef USEDECIMAL #define KNOWLEDGE_OF_C 1 #define KNOWLEDGE_OF_JAVA 2 #define KNOWLEDGE_OF_JAVASCRIPT 4 #define KNOWLEDGE_OF_PYTHON 8 #define KNOWLEDGE_OF_CPLUSPLUS 16 #define KNOWLEDGE_OF_PASCAL 32 #define KNOWLEDGE_OF_FORTRAN 64 #define KNOWLEDGE_OF_RUBY 128 #define KNOWLEDGE_OF_ADA 256 #define KNOWLEDGE_OF_LISP 512 #define KNOWLEDGE_OF_SQL 1024 #define KNOWLEDGE_OF_HTML 2048 #define KNOWLEDGE_OF_SWIFT 4096 #define KNOWLEDGE_OF_PROLOG 8192 #define KNOWLEDGE_OF_C_SHARP 16384 #define KNOWLEDGE_OF_PL1 32768 #define KNOWLEDGE_OF_INTEL_ASSEMBLER 65536 #define KNOWLEDGE_OF_IBM_ASSEMBLER 131072 #define KNOWLEDGE_OF_MIPS_ASSEMBLER 262144 #define KNOWLEDGE_OF_ARM_ASSEMBLER 524288 #define KNOWLEDGE_OF_COBOL 1048576 #define KNOWLEDGE_OF_APL 2097152 #define KNOWLEDGE_OF_R 4194304 #define KNOWLEDGE_OF_OBJECTIVE_C 8388608 #define KNOWLEDGE_OF_BASIC 16777216 #define KNOWLEDGE_OF_PHP 33554432 #define KNOWLEDGE_OF_GO 67108864
#else
#define KNOWLEDGE_OF_C 0x00000001 #define KNOWLEDGE_OF_JAVA 0x00000002 #define KNOWLEDGE_OF_JAVASCRIPT 0x00000004 #define KNOWLEDGE_OF_PYTHON 0x00000008 #define KNOWLEDGE_OF_CPLUSPLUS 0x00000010 #define KNOWLEDGE_OF_PASCAL 0x00000020 #define KNOWLEDGE_OF_FORTRAN 0x00000040 #define KNOWLEDGE_OF_RUBY 0x00000080 #define KNOWLEDGE_OF_ADA 0x00000100 #define KNOWLEDGE_OF_LISP 0x00000200 #define KNOWLEDGE_OF_SQL 0x00000400 #define KNOWLEDGE_OF_HTML 0x00000800 #define KNOWLEDGE_OF_SWIFT 0x00001000 #define KNOWLEDGE_OF_PROLOG 0x00002000 #define KNOWLEDGE_OF_C_SHARP 0x00004000 #define KNOWLEDGE_OF_PL1 0x00008000 #define KNOWLEDGE_OF_INTEL_ASSEMBLER 0x00010000 #define KNOWLEDGE_OF_IBM_ASSEMBLER 0x00020000 #define KNOWLEDGE_OF_MIPS_ASSEMBLER 0x00040000 #define KNOWLEDGE_OF_ARM_ASSEMBLER 0x00080000 #define KNOWLEDGE_OF_COBOL 0x00100000 #define KNOWLEDGE_OF_APL 0x00200000 #define KNOWLEDGE_OF_R 0x00400000 #define KNOWLEDGE_OF_OBJECTIVE_C 0x00800000 #define KNOWLEDGE_OF_BASIC 0x01000000 #define KNOWLEDGE_OF_PHP 0x02000000 #define KNOWLEDGE_OF_GO 0x04000000
#endif typedef struct personalInfo { char * firstName; char * lastName; int studentID; enum gradelevel {FRESHMAN, SOPHMORE, JUNIOR, SENIOR, GRAD, INSTRUCTOR} level; int languages; // See #defines for the bitmap values char message[100]; } personalInfo; #define BLOCK_SIZE 256
int writePersonalInfo (personalInfo * pi); //Write your personal info structure const char * getNext(void); //Get the next line to buffer write void commitBlock (char * buffer); //Flush out your 256 byte Buffer int checkIt (void); //Called at the end of your program to check the results
#else #error Do Not include header file assignment2.h more than once #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
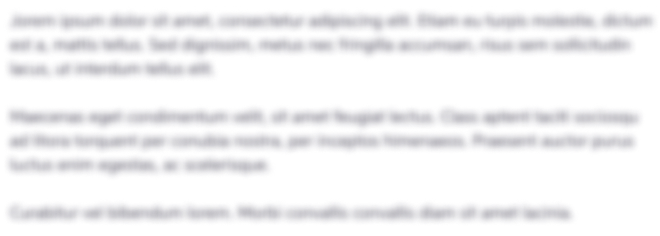
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started