Question
QS: Using the System.IO in C#, Create a graphical application of your choice to demonstrate the use of READ and WRITE operations like we did
QS: Using the System.IO in C#, Create a graphical application of your choice to demonstrate the use of READ and WRITE operations like we did in LAB#5. Be Creative.
Submit a Word Document with the Design and the Code.
For Reference:
Lab # 5: C# File Management Solution
Problem Description:
READ: Create a program to display all the contents of a text file on screen (note: you must use a StreamReader). The name of the file will be entered in the command line.
WRITE: Create a program to ask the user for several sentences until they just press Enter (empty sentence) and store them in a text file named "test.txt"
Solution:
GUI
Coding
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace FileRead
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string fileName = Microsoft.VisualBasic.Interaction.InputBox("Enter the Name of the File", "File Name", "", 50, 100);
label1.Text = label1.Text + fileName;
StreamReader myFile;
try
{
myFile = File.OpenText(fileName);
string line = "";
do
{
line = myFile.ReadLine();
if (line != null)
{
richTextBox1.AppendText(Environment.NewLine + line);
}
} while (line != null);
}catch(IOException)
{
MessageBox.Show("File Does not Exit", "Error", MessageBoxButtons.OK, MessageBoxIcon.Stop);
}
}
}
}
II.
Coding:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.IO;
namespace FileWrite
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
StreamWriter myFile;
string fileName = Microsoft.VisualBasic.Interaction.InputBox("What do you want to name your File", "File Name", "", 50, 100);
myFile = File.CreateText(fileName);
try
{
for (int i = 0; i
{
myFile.WriteLine(richTextBox1.Lines[i]);
}
}
catch (IOException)
{
MessageBox.Show("File Writing Error", "Error", MessageBoxButtons.OK, MessageBoxIcon.Stop);
}
myFile.Close();
MessageBox.Show("Creating the File "+fileName+" Was Successful", "Success", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
}
}
}
File Read Application File Details Enter File File Name File Content File Read Application File Details Enter File File Name File Content
Step by Step Solution
There are 3 Steps involved in it
Step: 1
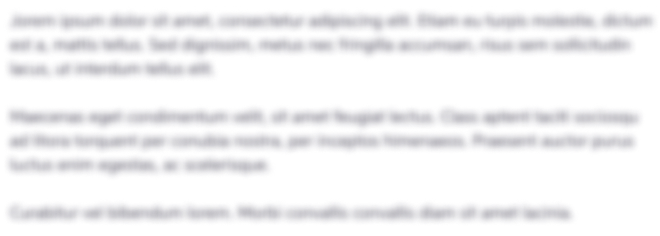
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started