Question
Question 1: Consider the following java class public class Test { public static void main(String[] args) { try { System.out.println(1/0) ; } catch (NullPointerException ex)
Question 1: Consider the following java class
public class Test {
public static void main(String[] args) {
try {
System.out.println(1/0) ;
}
catch (NullPointerException ex) {
System.out.println(NullPointerException);
}
catch (IndexOutOfBoundsException
ex) {
System.out.println("Exception IndexOutOfBoundsException ");
}
finally {
System.out.println("Finally in main");
}
System.out.println("End of main");
}
The output of the class is : .
Change the instruction System.out.println(1/0) thus the exception NullPointerException is caught.
|
Change the instruction System.out.println(1/0) thus the exception IndexOutOfBoundsException is caught.
|
Question 2: user defined exception. Consider the following java classes:
class NegativeException extends Exception
{
private int e;
NegativeException (int num1)
{
e = num1;
}
public String toString()
{
return "(" + e +") is less than 0";
}
}
class Demo1
{
static void sum(int num,int num1) throws NegativeException
{
if(num1<0)
{
throw new NegativeException (num1);
}
else
{
System.out.println(num+num1);
}
}
public static void main(String[] args)
{
try
{
sum(-5, 4);
}
catch(NegativeException e1)
{
System.out.println(e1);
}
}
}
2.1 : The class output is :
2.2 Similarly to the classes above write the following classes :
- ZeroException class that prints the message the Number is Zero
|
- DemoDivision that contains a method :
- static int division(int num1,int num2) throws ZeroException {} that calculates num1/num2 and throws the ZeroException when the num2 is equal to 0.
- Write the main function.
|
Question 3: Working with Files
3.1 : Ordinary file creation
Create a new folder named FileDemo
Open NotePad and create in FileDemo a new file named fname.txt. Write your Student Id and Your name and save the file.
Check if the file fname.txt exists in the folder FileDemo. The size of the file is .
The absolute path to the file is .
3.2 : A java code to read the file content :
import java.io.File;
import java.util.Scanner;
public class ReadFromFileUsingScanner
{
public static void main(String[] args) throws Exception
{
// pass the path to the file as a parameter
File file = new File(".);
Scanner sc = new Scanner(file);
while (sc.hasNextLine())
System.out.println(sc.nextLine());
}
}
The output is
Add 3 new names in the file and run the class. The new output is .
3.3 Write a text to a file
public class WriteFile {
// Main driver method
public static void main(String[] args)
{
// Content to be assigned to a file
// Custom input just for illustration purposes
String text = " Salam Alaykom";
// Try block to check if exception occurs
try {
// Create a FileWriter object
// to write in the file Path / file name
FileWriter fWriter = new FileWriter( .");
fWriter.write(text);
// Closing the file writing connection
fWriter.close();
// Display message for successful execution of
// program on the console
System.out.println("File is created successfully with the content.");
}
// Catch block to handle if exception occurs
catch (IOException e) {
// Print the exception
System.out.print(e.getMessage());
}
}
}
Complete the instruction FileWriter fWriter = new FileWriter( ."); and run your program
Check the content of your file. Conclude .
Write a new java code to append a new content to your file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
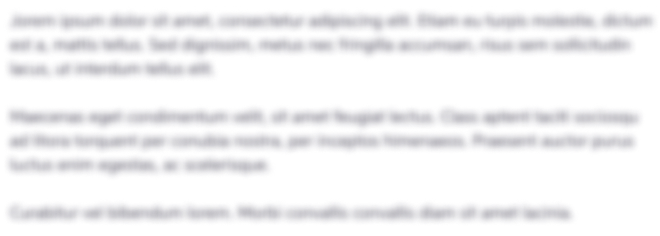
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started