Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Question 1)From a programmers perspective, what is the most important difference between the user interface of a console application and a graphical application? R10.5What
Question 1)"From a programmers perspective, what is the most important difference between the user interface of a console application and a graphical application?"
" R10.5What is an event object? An event source and event listener
" R10.13What is the difference between a label, a text field, and a text area?"
" R10.8How would you add a counter to the program in Section 10.2.1 that prints how often the button has been clicked? Where is the counter updated?"
99 10.2.1 Listening to Events User-interface events include Whenever the user of a graphical program types characters or key presses, mouse moves, uses the mouse anywhere inside one of the windows of the button clicks, menu selections, program, the program receives a notification that an event and so on. has occurred. For example, whenever the mouse moves a tiny interval over a window, a "mouse move" event is generated. Clicking a button or selecting a menu item generates an "action" event. Most programs don't want to be flooded by irrelevant events. For example, when a button is clicked with the mouse, the mouse moves over the button, then the mouse button is pressed, and finally the button is released. Rather than receiving all these mouse events, a program can indicate that it only cares about button clicks, not about the underlying mouse events. On the Seriy Tryapitsyn/iStockphoto. other hand, if the mouse input is used for drawing shapes on a virtual canvas, a program needs In an event-driven user interface, the to closely track mouse events. program receives an event whenever the user manipulates an input component. An event listener belongs to a Every program must indicate which events it needs to receive. class created by the application It does that by installing event listener objects. These objects programmer. Its methods are instances of classes that you must provide. The methods of describe the actions to be your event listener classes contain the instructions that you want to have executed when the events taken when an event occurs. occur. To install a listener, you need to know the event source. The event source is the user-interface component, such as a button, that generates a particular event. You add an event listener object to the appropriate event sources. Whenever the event occurs, the event source calls the appropriate methods of all attached event listeners. Event sources report on events. When an event occurs, the event source notifies all event listeners. This sounds somewhat abstract, so let's run through an extremely simple program that prints a message whenever a button is clicked. Button listeners must belong to a class that implements the ActionListener interface: public interface ActionListener { void actionPerformed(ActionEvent event); } This particular interface has a single method, actionPerformed. It is your job to supply a class whose actionPerformed method contains the instructions that you want executed whenever the button is clicked. Here is a very simple example of such a listener class: Listener.java 1 import java.awt.event. ActionEvent; 2 import java.awt.event. ActionListener; 3 5 An action listener that prints a message. 6 */ 7 public class ClickListener implements ActionListener 8 1 9 public void actionPerformed (ActionEvent event) 10 { 11 System.out.println("I was clicked."); 12 } 13) We ignore the event parameter variable of the actionPerformed methodit contains additional details about the event, such as the time at which it occurred. Note that the event handling classes are defined in the java.awt.event package. (AWT is the Abstract Window Toolkit, the Java library for dealing with windows and events.) p.900 Once the listener class has been declared, we need to construct an object of the class and add it to the button: Attach an ActionListener to each button so that your program can react to button clicks ActionListener listener = new ClickListener(); button.addActionListener(listener); Whenever the button is clicked, the Java event handling library calls listener.actionPerformed(event); As a result, the message is printed. You can test this program out by opening a console window, starting the Buttonviewer1 program from that console window, clicking the button, and watching the messages in the console window (see Figure 3). Terminal -/books/bjlo/code/ch10/seco2_016 java ButtonVieweri I was clicked I was clicked I was clicked Clickmal Figure 3 Implementing an Action Listener seco2_01/Button Framel.java 1 import java.awt.event.ActionListener; 2 import javax.swing.JButton; 3 import javax.swing.JFrame; 4 import javax.swing.JPanel; 5 7 This frame demonstrates how to install an action listener. 8 / 9 public class ButtonFramel extends JFrame 10 101 11 private static final int FRAME WIDTH = 100; 12 private static final int FRAME HEIGHT = 60; 13 14 public ButtonFramel() 15 { 16 createComponents(); 17 setSize(FRAME WIDTH, FRAME_HEIGHT); 1 19 20 private void createComponents() 21 { 18 Figure 3 Implementing an Action Listener seco2_01/Button Frame1.java 1 import java.awt.event.ActionListener; 2 import javax.swing. JButton; 3 import javax.swing.JFrame; 4 import javax.swing.JPanel; 5 7 11 13 14 19 This frame demonstrates how to install an action listener. 9 public class ButtonFramel extends JFrame 10 ( private static final int FRAME WIDTH = 100; 12 private static final int FRAME_HEIGHT = 60; public ButtonFramel() 15 { 16 createComponents(); 17 setSize(FRAME WIDTH, FRAME HEIGHT); 18 } 20 private void createComponents() C JButton button = new JButton("click me!"); JPanel panel = new JPanel(); panel.add(button); 25 add (panel); 26 ActionListener listener = new ClickListener(); button.addActionListener(listener); 29 30) seco2_01/ButtonVie wer1.java i import javax.swing.JFrame; 21 22 23 24 27 28 2 4 This program demonstrates how to install an action listener. 5 */ 6 public class ButtonViewer1 7 { 8 public static void main(String[] args) { 10 JFrame frame = new ButtonFrame1(); 11 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 12 frame.setVisible(true); 9 E 13 14 99 10.2.1 Listening to Events User-interface events include Whenever the user of a graphical program types characters or key presses, mouse moves, uses the mouse anywhere inside one of the windows of the button clicks, menu selections, program, the program receives a notification that an event and so on. has occurred. For example, whenever the mouse moves a tiny interval over a window, a "mouse move" event is generated. Clicking a button or selecting a menu item generates an "action" event. Most programs don't want to be flooded by irrelevant events. For example, when a button is clicked with the mouse, the mouse moves over the button, then the mouse button is pressed, and finally the button is released. Rather than receiving all these mouse events, a program can indicate that it only cares about button clicks, not about the underlying mouse events. On the Seriy Tryapitsyn/iStockphoto. other hand, if the mouse input is used for drawing shapes on a virtual canvas, a program needs In an event-driven user interface, the to closely track mouse events. program receives an event whenever the user manipulates an input component. An event listener belongs to a Every program must indicate which events it needs to receive. class created by the application It does that by installing event listener objects. These objects programmer. Its methods are instances of classes that you must provide. The methods of describe the actions to be your event listener classes contain the instructions that you want to have executed when the events taken when an event occurs. occur. To install a listener, you need to know the event source. The event source is the user-interface component, such as a button, that generates a particular event. You add an event listener object to the appropriate event sources. Whenever the event occurs, the event source calls the appropriate methods of all attached event listeners. Event sources report on events. When an event occurs, the event source notifies all event listeners. This sounds somewhat abstract, so let's run through an extremely simple program that prints a message whenever a button is clicked. Button listeners must belong to a class that implements the ActionListener interface: public interface ActionListener { void actionPerformed(ActionEvent event); } This particular interface has a single method, actionPerformed. It is your job to supply a class whose actionPerformed method contains the instructions that you want executed whenever the button is clicked. Here is a very simple example of such a listener class: Listener.java 1 import java.awt.event. ActionEvent; 2 import java.awt.event. ActionListener; 3 5 An action listener that prints a message. 6 */ 7 public class ClickListener implements ActionListener 8 1 9 public void actionPerformed (ActionEvent event) 10 { 11 System.out.println("I was clicked."); 12 } 13) We ignore the event parameter variable of the actionPerformed methodit contains additional details about the event, such as the time at which it occurred. Note that the event handling classes are defined in the java.awt.event package. (AWT is the Abstract Window Toolkit, the Java library for dealing with windows and events.) p.900 Once the listener class has been declared, we need to construct an object of the class and add it to the button: Attach an ActionListener to each button so that your program can react to button clicks ActionListener listener = new ClickListener(); button.addActionListener(listener); Whenever the button is clicked, the Java event handling library calls listener.actionPerformed(event); As a result, the message is printed. You can test this program out by opening a console window, starting the Buttonviewer1 program from that console window, clicking the button, and watching the messages in the console window (see Figure 3). Terminal -/books/bjlo/code/ch10/seco2_016 java ButtonVieweri I was clicked I was clicked I was clicked Clickmal Figure 3 Implementing an Action Listener seco2_01/Button Framel.java 1 import java.awt.event.ActionListener; 2 import javax.swing.JButton; 3 import javax.swing.JFrame; 4 import javax.swing.JPanel; 5 7 This frame demonstrates how to install an action listener. 8 / 9 public class ButtonFramel extends JFrame 10 101 11 private static final int FRAME WIDTH = 100; 12 private static final int FRAME HEIGHT = 60; 13 14 public ButtonFramel() 15 { 16 createComponents(); 17 setSize(FRAME WIDTH, FRAME_HEIGHT); 1 19 20 private void createComponents() 21 { 18 Figure 3 Implementing an Action Listener seco2_01/Button Frame1.java 1 import java.awt.event.ActionListener; 2 import javax.swing. JButton; 3 import javax.swing.JFrame; 4 import javax.swing.JPanel; 5 7 11 13 14 19 This frame demonstrates how to install an action listener. 9 public class ButtonFramel extends JFrame 10 ( private static final int FRAME WIDTH = 100; 12 private static final int FRAME_HEIGHT = 60; public ButtonFramel() 15 { 16 createComponents(); 17 setSize(FRAME WIDTH, FRAME HEIGHT); 18 } 20 private void createComponents() C JButton button = new JButton("click me!"); JPanel panel = new JPanel(); panel.add(button); 25 add (panel); 26 ActionListener listener = new ClickListener(); button.addActionListener(listener); 29 30) seco2_01/ButtonVie wer1.java i import javax.swing.JFrame; 21 22 23 24 27 28 2 4 This program demonstrates how to install an action listener. 5 */ 6 public class ButtonViewer1 7 { 8 public static void main(String[] args) { 10 JFrame frame = new ButtonFrame1(); 11 frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 12 frame.setVisible(true); 9 E 13 14
Step by Step Solution
There are 3 Steps involved in it
Step: 1
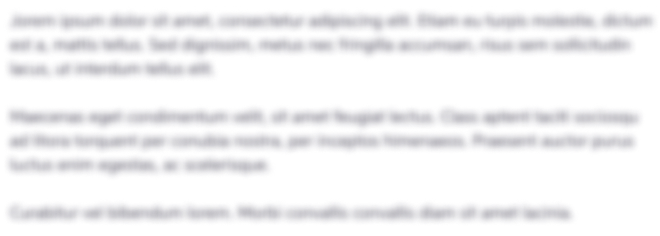
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started