Question
Question 2: You are given an abstract class named Engine with the following private fields: i) serial ( String type) cannot be null . A
Question 2:
You are given an abstract class named Engine with the following private fields:
i) serial (String type) cannot be null. A String with 6 small case letters exactly.
ii) type (String type) cannot be null. A String that can be petrol, hybrid or electric.
iii) capacity (double type) cannot be negative or zero.
iv) env_friend (boolean type) must be true of type = hybrid or type = electric and false otherwise.
Task 1: Complete the full-argument constructor and make sure that the input arguments satisfy the above requirements:
public Engine(______________________________________________) {
} |
Task 2: Complete the copy constructor and make sure to call the full-argument constructor using Objects.requireNonNull() method:
public Engine(______________________________________________) {
} |
Task 3: Complete the toString() method to return a String containing a similar content:
Engine(ABXCAV, petrol, 3.45 CC, environment-unfriendly)
when the serial, type and capacity are set to ABXCAV, petrol and 3.45, respectively.
Engine(YDBAFA, hybrid, 1.33 CC, environment-friendly)
when the serial, type and capacity are set to YDBAFA, hybrid and 1.33333, respectively.
Important Note: The capacity field is printed using 2 decimals only!
@Override public ______ toString(________________________________) {
} |
Task 4: Complete the equals() method to return true if the current Engine object and the argument object have equal serial and type fields and false otherwise.
@Override public ______ equals(________________________________) {
} |
Task 5: Complete the hashCode() method to return an int value equal to the bit-wise and of the hash codes of the serial and type fields.
@Override public ______ hashCode(________________________________) {
} |
Task 6: Add the declarations of the following abstract methods to the Engine class:
i) increaseCapacity(): This method takes a double argument that will be added to the capacity field if the type is set to petrol or hybrid. The input argument must be positive. The method will return true if the capacity field has been updated and false otherwise.
ii) makeEnvFriendly(): This no-argument method sets the type field to hybrid if it is initially set to petrol and to electric if it is initially set to hybrid. Otherwise, the method does not do anything!
public abstract class Engine {
} |
Task 7: Let the class Engine implement the Comparable interface. The compareTo() will return an int that represents the sum of the differences of the serial and type fields.
public abstract class Engine _____________________________{ @Override public ______ compareTo(______________________________){
} } |
Question 3:
Let the JetEngine class be a concrete class that extends the Engine class. The JetEngine class has an additional private field:
i) duration (double type) cannot be negative or zero.
Task 1: Complete the full-argument constructor of the JetEngine class. Make sure to call the full-argument constructor of the Engine class and validate the input arguments.
public class JetEngine _____________________________{ public JetEngine(______________________________){
} } |
Task 2: Override the toString() method to include the duration field. Make sure to call the toString() method of the Engine class and print the duration using 1 decimal only. The returned String should look like:
JetEngine(ABXCAV, petrol, 3.45 CC, environment-unfriendly, 25000.9 flight hours)
@Override public ______________ toString(_____________) {
}
|
Task 3:
i) Create an ArrayList of JetEngine objects. Set the initial capacity of the ArrayList to 100. Let jets_arr be the reference to the ArrayLitst.
ii) Read the JetEngine data from a file named engines.txt that has the following structure:
ABXCAV#petrol#3.459119#25000.987
.
.
public class CS102FinalExamSample { public static void main(String[] args)____________________{ ______________ jets_arr = _____________________
} }
|
Task 4:
Print the average capacity of the JetEngine stored in the ArrayList.
|
Task 5:
i) Print the details of the JetEngine with the smallest duration field.
ii) Print the details of the JetEngine with the largest duration field.
|
Task 6:
Sort the ArrayList of the JetEngine objects using the natural decreasing order. You should call one of the two methods: Arrays.sort() or Collections.sort().
|
Task 7:
Define a class JetEngineCapacityComparator that implements the Comparator interface. Let the compare() method return an int value that represents the difference of the capacity fields of the argument JetEngine objects.
|
Task 8:
Sort ArrayList of the JetEngine objects using the JetEngineCapacityComparator from smallest to largest based on the capacity field.
|
Task 9:
Store the all JetEngine objects with a capacity less or equal to 100 into an ArrayList referred by a reference named small_jets_arr.
|
Task 10:
Use an iterator to find the JetEngine object with the largest capacity in the ArrayList small_jets_arr.
|
Task 11:
Use the same iterator as above to find the JetEngine object with the smallest capacity in the ArrayList small_jets_arr.
|
Step by Step Solution
There are 3 Steps involved in it
Step: 1
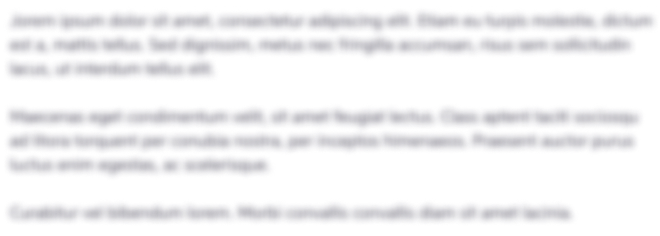
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started