Question
Question 8 0 / 1 point Given the code snippet below, what methods does an object of the Rectangle class have? class GeometricShape : def
Question 8 | 0 / 1 point |
Given the code snippet below, what methods does an object of the Rectangle class have?
class GeometricShape :
def __init__(self, x, y) :
self._x = x
self._y = y
self._fill = None
self._outline = "blue"
. . .
def getX(self) :
return self._x
def getY(self) :
return self._y
class Rectangle(GeometricShape) :
def __init__(self, x, y, width, height) :
super().__init__(x, y)
self._width = width
self._height = height
def getWidth(self) :
return self._width
def getHeight(self) :
return self._height
Question options:
getWidth(), getHeight() | |
getX(), getY(), getWidth(), getHeight() | |
getX(), getY(), setColor() | |
getX(), getY(), getWidth(), getHeight() |
Chapter 11 Consider the triangleArea function from the textbook shown below: 1. def triangleArea(sideLength) : 2. if sideLength <= 0 : 3. return 0 4. if sideLength == 1 : 5. return 1 6. smallerSideLength = sideLength - 1 7. smallerArea = triangleArea(smallerSideLength) 8. area = smallerArea + sideLength 9. return area What will happen if lines #2 and #3 are replaced with the following code segment? if sideLength <= 0 : return sideLength Question options:
Consider the following recursive function: def myPrint(n) : if n < 10 : print(n) else : m = n % 10 print(m) myPrint(n // 10) What does this function do? Question options:
Consider the triangleArea function from the textbook shown below: 1. def triangleArea(sideLength) : 2. if sideLength <= 0 : 3. return 0 4. if sideLength == 1 : 5. return 1 6. smallerSideLength = sideLength - 1 7. smallerArea = triangleArea(smallerSideLength) 8. area = smallerArea + sideLength 9. return area Assume that line #5 is changed to this: smallerArea = triangleArea(sideLength) This would cause infinite recursion for __________________________________ Question options:
Consider the following code snippet for calculating Fibonacci numbers recursively: 1. def fib(n) : 2. # assumes n >= 0 3. if n <= 1 : 4. return n 5. else : 6. return fib(n - 1) + fib(n - 2) Identify the terminating condition in this recursive function. Question options:
How many permutations are in a 5 letter word? Question options:
Recall the backtracking strategy outlined in the textbook. A similar strategy can be used to solve Sudoku puzzles. def solve(gameBoard) : status = examine(gameBoard) if status == CONTINUE : for nextBoard in extend(gameBoard) : solve(nextBoard) elif status == ACCEPT: ____________________ What code should be placed in the blank to complete the solution to this problem? Question options:
Which of the following strings is a palindrome? Question options:
How many squares are drawn by the following code segment? def tSquare(width, x, y, canvas) : canvas.drawRect(x, y, width, width) if width >= 4 : tSquare(width / 2, x, y, canvas) tSquare(width / 2, x + width / 2, canvas) tSquare(width / 2, x, y + width / 2, canvas) tSquare(width / 2, x + width / 2, y + width / 2, canvas) # Code to setup the canvas has been omitted tSquare(0, 0, 16, canvas) Question options:
Which of the following options could be used as a terminating condition for a recursive function that finds the middle character of a String with any number of characters? 1. I. the length of the String is 1 2. II. first and last String characters match 3. III. the String is not empty Question options:
Consider the function powerOfTwo shown below: 1. def powerOfTwo(n) : 2. if n == 1 : 3. return True 4. elif n % 2 == 1 : 5. return False 6. else : 7. return powerOfTwo(n / 2) What is the best interpretation of lines 2 and 3? Question options:
A unique permutation is one that is different from any other generated permutation. How many unique permutations does the string "bee" have? Question options:
The following code segment is supposed to determine whether or not a string is a palindrome, meaning that it is the same forward and backward. def isPalindrome(s) : return palindromeHelper(s, l, h) def palidromeHelper(s, l, h) : if h <= l : return True if s[l] != s[h] : return False ____________________ What line of code should be placed in the blank to achieve this goal? Question options:
What is the purpose of a recursive helper function? Question options:
|
Question 1 | 0 / 1 point |
Consider the following recursive function:
def myPrint(n) :
if n < 10 :
print(n)
else :
m = n % 10
print(m)
myPrint(n // 10)
What does this function do?
Question options:
It prints a positive value forward, digit by digit | ||
It prints a positive value backward, digit by digit | ||
It divides the number by 10 and prints out its last digit | ||
It divides the number by 10 and prints out the result | ||
Question 2 | 0 / 1 point |
Consider the following code segment:
def sumList(data) :
return sumListIndex(data, 0)
def sumListIndex(data, i) :
if i == len(data) :
return 0
else :
return data[i] + sumListIndex(data, i + 1)
This code segment contains an example of
Question options:
a recursive helper function | ||
backtracking | ||
iteration | ||
mutual recursion | ||
Question 6 | 0 / 1 point |
Consider the following recursive code snippet:
1. def mystery(n, m) :
2. if n <= 0 :
3. return 0
4. if n == 1 :
5. return m
6. return m + mystery(n - 1, m)
Identify the terminating condition(s) of function mystery?
Question options:
n <= 0 | ||
n == 1 | ||
n <= 0 or n == 1 | ||
n > 0 | ||
Question 7 | 0 / 1 point |
How many permutations are in a 5 letter word?
Question options:
5 | ||
120 | ||
12 | ||
1 | ||
Question 8 | 0 / 1 point |
A palindrome is a word or phrase spelled which reads the same forward or backward. Consider the following code snippet:
def palindrome(s) :
return isPal(s, 0, len(s) - 1)
def isPal(s, left, right) :
if left >= right :
return True
elif s[left] == s[right] :
return isPal(s, left + 1, right - 1)
else :
return False
What does the function palindrome return?
Question options:
True | ||
False | ||
a palindrome not found exception | ||
the Boolean value returned from the isPalfunction | ||
Question 9 | 0 / 1 point |
What is the purpose of a recursive helper function?
Question options:
Shield the user of the recursive function from the recursive details | ||
Speed up the execution | ||
Eliminate the recursion | ||
Add another base case | ||
Question 10 | 0 / 1 point |
Recall the permutations function from Section 11.5 in the textbook.
def permutations(word) :
result = []
if len(word) == 0 :
result.append(word)
return result
else:
____________________
shorter = word[ : i] + word[i + 1 : ]
shorterPermutations = permutations(shorter)
for string in shorterPermutations :
result.append(word[i] + string)
return result
In the textbook, the line now represented by the blank was for i in range(len(word)) :. What would happen if the blank was filled in with for i in range(len(word) - 1, -1, -1) :
Question options:
The same permutations of the word would be generated in the same order | ||
The same permutations of the word would be generated in the reverse order | ||
The same permutations of the word would be generated in another order that is not the same as the original code segment, or the reverse of the original order | ||
The modified code segment will not generate the same set of permutations | ||
Question 2 | 0 / 1 point |
Consider the triangleArea function from the textbook shown below:
1. def triangleArea(sideLength) :
2. if sideLength <= 0 :
3. return 0
4. if sideLength == 1 :
5. return 1
6. smallerSideLength = sideLength - 1
7. smallerArea = triangleArea(smallerSideLength)
8. area = smallerArea + sideLength
9. return area
What will happen if line #6 is changed to:
smallerSideLength = sideLength
Question options:
Infinite recursion will occur when sideLength is equal to 0. | ||
Infinite recursion will occur when sideLength is equal to 1. | ||
Infinite recursion will occur when sideLength is greater than or equal to 2. | ||
Infinite recursion will occur for any value of sideLength. | ||
Question 5 | 0 / 1 point |
How many squares are drawn by the following code segment?
def tSquare(width, x, y, canvas) :
canvas.drawRect(x, y, width, width)
if width >= 4 :
tSquare(width / 2, x, y, canvas)
tSquare(width / 2, x + width / 2, canvas)
tSquare(width / 2, x, y + width / 2, canvas)
tSquare(width / 2, x + width / 2, y + width / 2, canvas)
# Code to setup the canvas has been omitted
tSquare(0, 0, 8, canvas)
Question options:
1 | ||
4 | ||
5 | ||
8 | ||
Question 6 | 0 / 1 point |
Consider the following recursive function:
def myPrint(n) :
if n < 10 :
print(n)
else :
m = n % 10
print(m, end="")
myPrint(n // 10)
What is printed for the call myPrint(821)?
Question options:
10 | ||
12 | ||
128 | ||
821 | ||
Question 9 | 0 / 1 point |
Consider the following function:
1. def mystery(n, m) :
2. if n == 0 : # special case 1
3. return 0
4. if n == 1 : # special case 2
5. return m
6. return m + mystery(n - 1), m)
What will happen if lines #2 and #3 were swapped with lines #4 and #5?
Question options:
The original function and the modified function will return the same result for all integer values of n and m. | |||
The original function and the modified function will return different results for all integer value of n and m. | |||
The original function and the modified function will return the same result when n is greater than m, and different results when m is greater than n. | |||
The original function and the modified function will return the same result when n is less than m, and different results when m is less than n. | |||
Question 4 | 0 / 1 point | ||
The following code segment is supposed to determine whether or not a string is a palindrome, meaning that it is the same forward and backward.
def isPalindrome(s) :
return palindromeHelper(s, l, h)
def palidromeHelper(s, l, h) :
if h <= l :
return True
if s[l] != s[h] :
return False
____________________
What line of code should be placed in the blank to achieve this goal?
Question options:
isPalindrome(s, l, h) | ||
isPalindrome(s, l + 1, h - 1) | ||
palindromeHelper(s, l, h) | ||
palindromeHelper(s, l + 1, h - 1) | ||
Question 6 | 0 / 1 point |
Complete the code for the myFactorial recursive function shown below, which is intended to compute the factorial of the value passed to the function:
1. def myFactorial(n) :
2. if _____________________________ :
3. return 1
4. else :
5. return n * myFactorial(n - 1)
Question options:
n == 1 | ||
(n - 1) == 1 | ||
n * (n - 1) == 1 | ||
myFactorial(n) == 1 | ||
Question 10 | 0 / 1 point |
Recall the permutations function from Section 11.5 in the textbook.
def permutations(word) :
result = []
if len(word) == 0 :
result.append(word)
return result
else:
for i in range(len(word)) :
shorter = word[ : i] + word[i + 1 : ]
shorterPermutations = permutations(shorter)
for string in shorterPermutations :
result.append(word[i] + string)
return result
What is the base case for this function?
Question options:
The empty list | |
Any list containing exactly one character | |
The empty string | |
Any string containing exactly one character |
A binary search ____ a linear search.
Question options:
can't be compared to | |||
generally has a similar running time to | |||
is generally faster than | |||
is generally slower than | |||
Question 3 | 0 / 1 point | ||
Which sorting algorithm has better than O(n2) behavior, even in the worst case?
Question options:
Insertion sort | |||
Merge sort | |||
Quicksort | |||
Selection sort | |||
Question 4 | 0 / 1 point | ||
Consider the selection sort function shown below:
def selectionSort(values) :
for i in range(len(values)) :
minPos = minimumPosition(values, i)
swap(values, minPos, i)
The function works correctly in its current form. What would happen if the line calling swap was replaced with: swap(values, i, minPos)?
Question options:
The list would still be sorted, but it would take one less iteration | |||
The list would still be sorted, using the same number of iterations | |||
The list would still be sorted, but it would take one more iteration | |||
A runtime error would occur | |||
Question 5 | 0 / 1 point | ||
Consider the following function for performing a binary search:
def binarySearch(values, low, high, target) :
if low <= high :
mid = (low + high) // 2
if values[mid] == target :
return mid
elif values[mid] < target :
return binarySearch(values, mid + 1, high, target)
else :
return binarySearch(values, low, mid - 1, target)
else :
return -1
How many elements will be visited when values is [0, 1, 2, 3, 4, 5, 6, 7, 8, 9], low is 0, high is 9, and target is 2?
Question options:
1 | |||
3 | |||
5 | |||
10 | |||
Question 6 | 0 / 1 point | ||
In the textbook, we found that the number of element visits for merge sort totaled n + 5nlog2n. Lets consider sorting 1024 elements. How many visits are needed?
Question options:
1,024 | |||
6,144 | |||
51,200 | |||
52,224 | |||
Question 7 | 0 / 1 point | ||
Binary search is an ____ algorithm.
Question options:
O(n) | |||
O(n2) | |||
O(log n) | |||
O(n log n) | |||
Question 8 | 0 / 1 point | ||
Consider the following function for performing a linear search:
def linearSearch(values, target) :
for i in range(len(values)) :
if values[i] == target :
return i
return -1
How many elements will be visited when values is [1, 5, 7, 6, 2, 4, 9, 3, 8, 0] and target is 2?
Question options:
0 | |||
2 | |||
5 | |||
10 | |||
Question 10 | 0 / 1 point | ||
How many times can an list with 729 elements be cut into three equal pieces?
Question options:
4 | |
5 | |
6 | |
7 |
In the textbook, we found that the number of element visits for merge sort totaled n + 5nlog2n. Which of the following is the appropriate big-Oh notation for merge sort?
Question options:
5nlog2n | |||
n + log2 | |||
n + 5n | |||
nlog2n | |||
Question 2 | 0 / 1 point | ||
In big-Oh notation, when we consider the order of the number of visits an algorithm makes, what do we ignore?
I. Power of two terms
II. The coefficients of the terms
III. All lower order terms
Question options:
I only | |||
II only | |||
I and II only | |||
II and III only | |||
Question 3 | 0 / 1 point | ||
Consider the following variation of the selection sort algorithm:
def sort(values) :
for i in range(len(values)) :
maxPos = i
for j in range(i + 1, len(values)) :
if values[j] > values[maxPos] :
maxPos = j
temp = values[maxPos]
values[maxPos] = values[i]
values[i] = values[maxPos]
If this algorithm takes 5 seconds to sort 15,000 elements, how long would you expect it to take to sort 30,000 elements?
Question options:
5 seconds | |||
10 seconds | |||
25 seconds | |||
50 seconds | |||
Question 6 | 0 / 1 point | ||
The checklist function is shown below:
def checklist(lst) :
if(lst[0] >= lst[len(lst)-1] :
return True
return False
What can you conclude about the running time of this function?
Question options:
Its running time will be O(n). | |||
Its running time will be O(n2). | |||
Its running time will be O(log n). | |||
Its running time will be O(1). | |||
Question 8 | 0 / 1 point | ||
Consider the following function, which correctly merges two lists:
def mergeLists(first, second, values) :
iFrist = 0
iSecond = 0
j = 0
while iFirst < len(first) and iSecond < len(second) :
if first[iFirst] < second[iSecond] :
values[j] = first[iFirst]
iFirst = iFirst + 1
else :
values[j] = second[iSecond]
iSecond = iSecond + 1
j = j + 1
while iFrist < len(first) :
values[j] = first[iFirst]
iFirst = iFirst + 1
j = j + 1
while iSecond < len(second) :
values[j] = second[iSecond]
iSecond = iSecond + 1
j = j + 1
What is the big-Oh complexity of this algorithm, where n is the total number of elements in first and second?
Question options:
O(1) | |||
O(log(n)) | |||
O(n) | |||
O(n2) | |||
Question 9 | 0 / 1 point | ||
The following program is supposed to time the performance of the selectionSort function. Right now it is missing the code, startTime = time(), which records the starting time. Where should the missing code be inserted?
# Line 1
values = []
# Line 2
for i in range(10000) :
values.append(randint(1, 100))
# Line 3
selectionSort(values)
# Line 4
endTime = time()
Question options:
Line 1 | |||
Line 2 | |||
Line 3 | |||
Line 4 | |||
Question 10 | 0 / 1 point | ||
Selection sort has O(n2) complexity. If a computer can sort 1,000 elements in 4 seconds, approximately how many seconds will it take the computer to sort 1,000 times that many, or 1,000,000 elements?
Question options:
16 | |
1,000 | |
1,000,000 | |
4,000,000 |
An algorithm that cuts the work in half in each step is an ____ algorithm.
Question options:
O(n) | |||
O(n2) | |||
O(log n) | |||
O(n log n) | |||
Question 4 | 0 / 1 point | ||
Consider the following function for performing a binary search:
def binarySearch(values, low, high, target) :
if low <= high :
mid = (low + high) // 2
if values[mid] == target :
return mid
elif values[mid] < target :
return binarySearch(values, mid + 1, high, target)
else :
return binarySearch(values, low, mid - 1, target)
else :
return -1
How many elements will be visited when values is [0, 1, 2, 3, 4, 5, 6, 7, 8, 9], low is 0, high is 9, and target is 10?
Question options:
0 | |||
2 | |||
4 | |||
10 | |||
Question 5 | 0 / 1 point | ||
Suppose you have a phone number and need to find the address that it corresponds to. If there are 2,000,000 entries, how many do you expect to search in a printed phone directory before finding the address you are looking for?
Question options:
Approximately 50,000 records | |||
Approximately 75,000 records | |||
Approximately 1,000,000 records | |||
Approximately 1,200,000 records | |||
Question 6 | 0 / 1 point | ||
Consider the selection sort function and function call shown below:
def selectionSort(values) :
for i in range(len(values)) :
print(values)
minPos = minimumPosition(values, i)
swap(values, minPos, i)
data = [1, 2, 3]
selectionSort(data)
print(data)
What is displayed when this code segment executes?
Question options:
[]
| |||
[1, 2, 3]
| |||
[3, 2, 1]
| |||
[1, 2, 3] [1, 2, 3] [1, 2, 3] [1, 2, 3]
| |||
Question 9 | 0 / 1 point | ||
Which of the following statements about running times of algorithms is correct?
Question options:
An algorithm that is O(1) means that only one comparison takes place. | |
When determining the running time, constants are not taken into consideration. | |
When determining the running time, lower-order terms must be taken into consideration. | |
An algorithm that is O(n) means that the number of comparisons does not grow as the size of the list increases. |
Question 1 | 0 / 1 point |
Consider the selection sort function and function call shown below:
def selectionSort(values) :
for i in range(len(values)) :
print(values)
minPos = minimumPosition(values, i)
swap(values, minPos, i)
data = [9, 1, 7, 2]
selectionSort(data)
print(data)
What is displayed when this code segment executes?
Question options:
[1, 2, 7, 9]
| ||
[9, 1, 7, 2] [1, 9, 7, 2] [1, 2, 7, 9]
| ||
[9, 1, 7, 2] [1, 9, 7, 2] [1, 2, 7, 9] [1, 2, 7, 9]
| ||
[9, 1, 7, 2] [1, 9, 7, 2] [1, 2, 7, 9] [1, 2, 7, 9] [1, 2, 7, 9]
| ||
Question 2 | 0 / 1 point |
Consider the selection sort function and function call shown below:
def selectionSort(values) :
for i in range(len(values)) :
print(values)
minPos = minimumPosition(values, i)
swap(values, minPos, i)
data = [1, 2, 3]
selectionSort(data)
print(data)
What is displayed when this code segment executes?
Question options:
[]
| ||
[1, 2, 3]
| ||
[3, 2, 1]
| ||
[1, 2, 3] [1, 2, 3] [1, 2, 3] [1, 2, 3]
| ||
Question 9 | 0 / 1 point |
Recall the merge sort algorithm presented in the textbook. That algorithm requires T(n) = T(n / 2) + T(n / 2) + 5n visits to sort a list of n elements. The function to merge two sorted lists requires a total of 5n visits. What does T(n / 2) describe?
Question options:
The total number of visits needed to merge sort a list of n elements | ||
Half the number of visits needed to merge sort a list of n elements | ||
The total number of visits needed to merge sort a list of n / 2 elements | ||
Half the number of visits needed to merge sort a list of n / 2 elements | ||
Question 10 | 0 / 1 point |
The function findLargest examines the elements of an list lst which contains non-negative values
curLargest = -1
for i in range(len(lst)) :
if lst[i] >= curLargest :
curLargest = lst[i]
What can you conclude about the running time of this section of code?
Question options:
Its running time will be O(n). | |
Its running time will be O(n2). | |
Its running time will be O(log n). | |
Its running time will be O(n log n). |
Question 1 | 0 / 1 point |
Consider the selection sort function shown below:
def selectionSort(values) :
for i in range(len(values)) :
minPos = minimumPosition(values, i)
swap(values, minPos, i)
The function works correctly in its current form. What would happen if the line calling swap was replaced with: swap(values, i, minPos)?
Question options:
The list would still be sorted, but it would take one less iteration | ||
The list would still be sorted, using the same number of iterations | ||
The list would still be sorted, but it would take one more iteration | ||
A runtime error would occur | ||
Question 3 | 0 / 1 point |
In the textbook, we found that the number of element visits for merge sort totaled n + 5nlog2n. Lets consider sorting 1024 elements. How many visits are needed?
Question options:
1,024 | ||
6,144 | ||
51,200 | ||
52,224 | ||
Question 8 | 0 / 1 point |
Consider the swap function shown below from the SelectionSorter class. If we modified it as shown in the swap2 function shown below, what would be the effect on the sort function?
def swap(values, i, j) :
temp = values[i]
values[i] = values[j]
values[j] = temp
def swap2(values, i, j) :
values[i] = values[j]
values[j] = values[i]
Question options:
There would be no effect | |
Some list elements would be overwritten | |
It would sort the list in reverse order | |
It would still be correct, but run a little faster |
Question 2 | 0 / 1 point |
Assume we are using quicksort to sort a list into ascending order. Where does quicksort place the pivot element after partitioning the list?
Question options:
The pivot element is placed at the lowest index in the list that is still available. | ||
The pivot element is placed at the highest index in the list that is still available. | ||
The pivot element is placed in a position that is closer to its final correct location. | ||
The pivot element is placed in its final correct location. | ||
Question 7 | 0 / 1 point |
A particular algorithm visits n3 + nlog(n) + n! elements in order to perform its task on a list of n elements. Which big-Oh expression best describes the growth rate of this algorithm?
Question options:
O(n) | ||
O(n3) | ||
O(n!) | ||
O(nlog(n)) | ||
Question 8 | 0 / 1 point |
Which sorting algorithm has better than O(n2) behavior, even in the worst case?
Question options:
Insertion sort | |
Merge sort | |
Quicksort | |
Selection sort |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
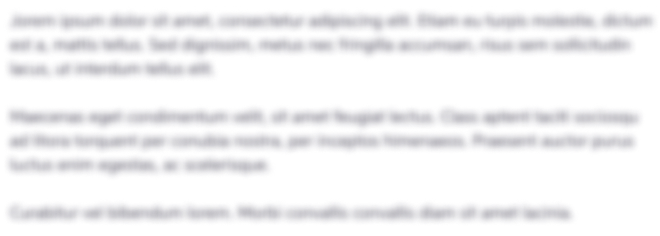
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started