Question
Question Description CarSorter.java import java.util.ArrayList; import java.util.Collections; public class CarSorter { public static void main(String[] args) { ArrayList list = new ArrayList(); Car car; car
Question Description
CarSorter.java
import java.util.ArrayList;
import java.util.Collections;
public class CarSorter
{
public static void main(String[] args)
{
ArrayList list = new ArrayList();
Car car;
car = new Car("BMW",5/100.0); // 5 Liters per 100 KM
car.addGas(20.0); // 20 Liters
list.add(car);
car = new Car("Audi",12/100.0); // 5 Liters per 100 KM
car.addGas(10.0); // 20 Liters
list.add(car);
car = new Car("Mercedes",1/100.0); // 5 Liters per 100 KM
car.addGas(50.0); // 20 Liters
list.add(car);
car = new Car("Prius",25/100.0); // 5 Liters per 100 KM
car.addGas(25.0); // 20 Liters
list.add(car);
car = new Car("Corolla",17/100.0); // 5 Liters per 100 KM
car.addGas(43.0); // 20 Liters
list.add(car);
for (Car c: list)
{
System.out.println(c);
}
System.out.println("After sorting based on fuel efficiency:");
Collections.sort(list);
for (Car c: list)
{
System.out.println(c);
}
System.out.println("Expected:");
System.out.println("Name: Mercedes Gas Level: 50.0 FuelEfficiency: 0.01");
System.out.println("Name: BMW Gas Level: 20.0 FuelEfficiency: 0.05");
System.out.println("Name: Audi Gas Level: 10.0 FuelEfficiency: 0.12");
System.out.println("Name: Corolla Gas Level: 43.0 FuelEfficiency: 0.17");
System.out.println("Name: Prius Gas Level: 25.0 FuelEfficiency: 0.25");
}
}
Car.java
/**
A class suitable for the simulating a car driving.
*/
public class Car implements Comparable
{
private String name;
private double fuelEfficiency;
private double gasLevel;
/**
Initializes a car with a given fuel efficiency
@param fuelEfficiency the default fuel efficiency
*/
public Car(String name, double fuelEfficiency)
{
this.name = name;
this.fuelEfficiency = fuelEfficiency;
gasLevel = 0;
}
/**
Puts gas in the tank.
@param gas amount of gas to add
*/
public void addGas(double gas)
{
this.gasLevel = gasLevel + gas;
}
/**
Simulates driving the car and thus reducing the gas in the tank
@param distance miles driven
*/
public void drive(double distance)
{
gasLevel -= distance * fuelEfficiency;
}
/**
Returns the current gas level.
@return current gas level
*/
public double getGasLevel()
{
return gasLevel;
}
//-----------Start below here. To do: approximate lines of code = 4
// write a method to implement the Comparable interface
// The method should compare two cars based on fuel efficiency (higher fuel efficiency is better)
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
public String toString()
{
return "Name: " + name + " Gas Level: " + gasLevel + " FuelEfficiency: " + fuelEfficiency;
}
}
P.s: please besides the code, also include a screenshot of code, and the output to confirm code doesn't get edited out and the output satisfies the problem!!
See the following files: CarSorter.java * Car.java (has todo) Approximate total lines of code required: 4
Step by Step Solution
There are 3 Steps involved in it
Step: 1
The detailed answer for the above question is provided below Your Answer CarSorterjava import javautilArrayList import javautilCollections public clas...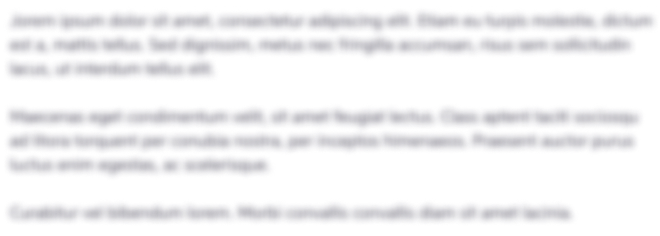
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started