Question
question Modular programming is a general programming concept. It involves separating a programs functions into independent pieces or building blocks, each containing all the parts
question
Modular programming is a general programming concept. It involves separating a programs functions into independent pieces or building blocks, each containing all the parts needed to execute a single aspect of the functionality. Together, the modules make up the executable application program. Modular programming usually makes your code easier to read because it means separating it into functions that each only deal with one aspect of the overall functionality. It can make your programs a lot easier to understand and to maintain.
Now, we will put all functions from this lab together and complete the program. Copy your solution to all the previous functions in this lab into the bottom of the answer box below. The basic structure of the main() function has been given to you. You will need to complete the while loop by invoking a few functions:
- Display the list of products by calling the show_products() function.
- Prompt the user to enter a product code by calling the get_user_selection_input() function. (This has been done for you)
- If the user entered '0' and the orders list contains at least one order, the program should display a list of orders and print the total cost. Also the program should save the updated list to a text file by calling the save_stock() function and then print the message 'Thank you for shopping with us.' at the end.
- If the user entered '0' and the orders list is empty, the program should just print the message 'Thank you for shopping with us.'
- If the user entered a valid code, the program should get the index of the item corresponding to the code item, by calling the get_item_index() function. If the index is valid, the program adds the order to the orders list by calling the add_to_orders() function and displays the list of orders by calling the show_orders() function.
For example:
Test
main()
input:
11 12 13 0 |
Result:
------------------------------ WELCOME TO THE VENDING MACHINE ------------------------------ Available Products: Code=11, Coca Cola Soft Drink 500ml, $4.00 Code=12, L & P Soft Drink Lemon & Paeroa 500ml, $4.00 Code=13, V Blue Drink can 500mL, $3.50 Code=14, V Vitalise Energy Drink 500ml, $3.50 Code=15, Pump Water NZ Spring 750ml, $2.50 Code=16, Twix Chocolate Bar 50g, $2.50 Code=17, Nestle Kit Kat Chocolate Bar 4 Finger, $2.40 Code=18, Snickers Chocolate Bar 50g, $2.00 Code=19, Cadbury Chocolate Bar Crunchie 50g, $2.00 Code=20, Cadbury Picnic Chocolate Bar 46g, $2.00 Select a product. Enter 0 to complete. Enter Code: 11 Coca Cola Soft Drink 500ml $4 Total cost $4.00 Available Products: Code=11, Coca Cola Soft Drink 500ml, $4.00 Code=12, L & P Soft Drink Lemon & Paeroa 500ml, $4.00 Code=13, V Blue Drink can 500mL, $3.50 Code=14, V Vitalise Energy Drink 500ml, $3.50 Code=15, Pump Water NZ Spring 750ml, $2.50 Code=16, Twix Chocolate Bar 50g, $2.50 Code=17, Nestle Kit Kat Chocolate Bar 4 Finger, $2.40 Code=18, Snickers Chocolate Bar 50g, $2.00 Code=19, Cadbury Chocolate Bar Crunchie 50g, $2.00 Code=20, Cadbury Picnic Chocolate Bar 46g, $2.00 Select a product. Enter 0 to complete. Enter Code: 12 Coca Cola Soft Drink 500ml $4 L & P Soft Drink Lemon & Paeroa 500ml $4 Total cost $8.00 Available Products: Code=11, Coca Cola Soft Drink 500ml, $4.00 Code=12, L & P Soft Drink Lemon & Paeroa 500ml, $4.00 Code=13, V Blue Drink can 500mL, $3.50 Code=14, V Vitalise Energy Drink 500ml, $3.50 Code=15, Pump Water NZ Spring 750ml, $2.50 Code=16, Twix Chocolate Bar 50g, $2.50 Code=17, Nestle Kit Kat Chocolate Bar 4 Finger, $2.40 Code=18, Snickers Chocolate Bar 50g, $2.00 Code=19, Cadbury Chocolate Bar Crunchie 50g, $2.00 Code=20, Cadbury Picnic Chocolate Bar 46g, $2.00 Select a product. Enter 0 to complete. Enter Code: 13 Coca Cola Soft Drink 500ml $4 L & P Soft Drink Lemon & Paeroa 500ml $4 V Blue Drink can 500mL $3.5 Total cost $11.50 Available Products: Code=11, Coca Cola Soft Drink 500ml, $4.00 Code=12, L & P Soft Drink Lemon & Paeroa 500ml, $4.00 Code=13, V Blue Drink can 500mL, $3.50 Code=14, V Vitalise Energy Drink 500ml, $3.50 Code=15, Pump Water NZ Spring 750ml, $2.50 Code=16, Twix Chocolate Bar 50g, $2.50 Code=17, Nestle Kit Kat Chocolate Bar 4 Finger, $2.40 Code=18, Snickers Chocolate Bar 50g, $2.00 Code=19, Cadbury Chocolate Bar Crunchie 50g, $2.00 Code=20, Cadbury Picnic Chocolate Bar 46g, $2.00 Select a product. Enter 0 to complete. Enter Code: 0 Coca Cola Soft Drink 500ml $4 L & P Soft Drink Lemon & Paeroa 500ml $4 V Blue Drink can 500mL $3.5 Total cost $11.50 Thank you for shopping with us.
function the question give
def main(): stock_list = load_stock("stocks.txt") max_code_of_products = 20 display_welcome_message() orders = [] option = 1 while option in range(1, max_code_of_products + 1): #complete this: display the list of products option = get_user_selection_input(0, max_code_of_products) if #complete this : check if the option is '0' if len(orders) > 0: #complete this: display the list of orders save_stock(stock_list) print("Thank you for shopping with us.") else: item_index = #complete this: get the item index if item_index != -1: #complete this: add an order to the orders list show_orders(orders) print() def display_welcome_message(): print("------------------------------") print("WELCOME TO THE VENDING MACHINE") print("------------------------------") print() # Copy all your functions from Q1 to Q7 into here(I already down this) def load_stock(filename): file=open(filename,'r') text=file.read().split(" ") file.close() return text def save_stock(stock_list): output_file = open('pzha510.txt',"w") for i in range(len(stock_list)): output_file.writelines(stock_list[i]+" ") output_file.close() return def show_products(stock_list): print('Available Products:') for x in stock_list: code, name, p, q = x.split(',') price = float(p) qty = int(q) if qty>0: print("Code=",code,", ",name,", ",'${:.2f}'.format(price),sep='') print('Select a product. Enter 0 to complete.') print() return def get_user_selection_input(minimum, maximum): number=input("Enter Code: ") number=int(number) while (number > maximum or number < minimum) and number!=0: number=input("Enter Code: ") number=int(number) return number def get_item_index(search_code, products_list): b = 1 b=int(b) for x in products: a,e,c,d=x.split(",") a=int(a) if a == search_code: b = 0 return products.index(x) if b == 1: f="The product "+str(search_code)+" does not exist."+" "+"-1" return f def add_to_orders(item_index, stock_list, orders): list=stock_list[item_index].split(",") orders.append((list[1],list[2])) list[3]=int(list[3])-1 if list[3]==0: stock_list.pop(item_index) else: string='{},{},{},{}'.format(list[0],list[1],list[2],list[3]) stock_list[item_index]=string return def show_orders(orders): total=0 for x in orders: total=float(x[1])+total print(x[0]," $",x[1],sep="") print("Total cost $","{:.2f}".format(total),sep="") return
Step by Step Solution
There are 3 Steps involved in it
Step: 1
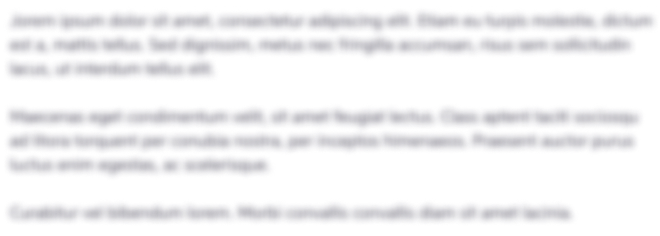
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started