Question
***Question part: You need to implement the same class as GIVEN IN EXAMPLE BELOW, but this time implement the STACK AS A SINGLE LINKED LIST.
***Question part:
You need to implement the same class as GIVEN IN EXAMPLE BELOW, but this time implement the STACK AS A SINGLE LINKED LIST. The test of the class should be done with the SAME MAIN FUNCTION as in the example. Please do not change the main function to avoid losing points.
Please place head comments AND comments to each function just like in the example for full credit
"HighestGPAData.txt"
3.4 Randy 3.2 Kathy 2.5 Colt 3.4 Tom 3.8 Ron 3.8 Mickey 3.6 Peter 3.5 Donald 3.8 Cindy 3.7 Dome 3.9 Andy 3.8 Fox 3.9 Minnie 2.7 Gilda 3.9 Vinay 3.4 Danny
Question part ENDS here ********
/// This program uses the class myStack to determine the
/// highest GPA from a list of students with their GPA.
/// The program also outputs the names of the students
/// who received the highest GPA.
///*********************************************************
///Header file: myStack.h
//#ifndef H_StackType
//#define H_StackType
#include
#include
#include
#include
#include
///#include "stackADT.h"
using namespace std;
template
class stackType /*: public stackADT
{
public:
const stackType
///Overload the assignment operator.
void initializeStack();
///Function to initialize the stack to an empty state.
///Postcondition: stackTop = 0
bool isEmptyStack() const;
///Function to determine whether the stack is empty.
///Postcondition: Returns true if the stack is empty,
/// otherwise returns false.
bool isFullStack() const;
///Function to determine whether the stack is full.
///Postcondition: Returns true if the stack is full,
/// otherwise returns false.
void push(const Type& newItem);
///Function to add newItem to the stack.
///Precondition: The stack exists and is not full.
///Postcondition: The stack is changed and newItem
/// is added to the top of the stack.
Type top() const;
///Function to return the top element of the stack.
///Precondition: The stack exists and is not empty.
///Postcondition: If the stack is empty, the program
/// terminates; otherwise, the top element
/// of the stack is returned.
void pop();
///Function to remove the top element of the stack.
///Precondition: The stack exists and is not empty.
///Postcondition: The stack is changed and the top
/// element is removed from the stack.
stackType(int stackSize = 100);
///constructor
///Create an array of the size stackSize to hold
///the stack elements. The default stack size is 100.
///Postcondition: The variable list contains the base
/// address of the array, stackTop = 0, and
/// maxStackSize = stackSize.
stackType(const stackType
//copy constructor
~stackType();
///destructor
///Remove all the elements from the stack.
///Postcondition: The array (list) holding the stack
/// elements is deleted.
private:
int maxStackSize; ///variable to store the maximum stack size
int stackTop; ///variable to point to the top of the stack
Type *list; ///pointer to the array that holds the
///stack elements
void copyStack(const stackType
///Function to make a copy of otherStack.
///Postcondition: A copy of otherStack is created and
/// assigned to this stack.
};
template
void stackType
{
stackTop = 0;
}///end initializeStack
template
bool stackType
{
return (stackTop == 0);
}///end isEmptyStack
template
bool stackType
{
return (stackTop == maxStackSize);
} ///end isFullStack
template
void stackType
{
if (!isFullStack())
{
list[stackTop] = newItem; ///add newItem to the
///top of the stack
stackTop++; ///increment stackTop
}
else
cout << "Cannot add to a full stack." << endl;
}///end push
template
Type stackType
{
assert(stackTop != 0); ///if stack is empty,
///terminate the program
return list[stackTop - 1]; ///return the element of the
///stack indicated by
///stackTop - 1
}///end top
template
void stackType
{
if (!isEmptyStack())
stackTop--; //decrement stackTop
else
cout << "Cannot remove from an empty stack." << endl;
}///end pop
template
stackType
{
if (stackSize <= 0)
{
/// note that cin and cout are not giof candidates to be ghere----done for the sake of simplicity.......
cout << "Size of the array to hold the stack must "
<< "be positive." << endl;
cout << "Creating an array of size 100." << endl;
maxStackSize = 100;
}
else
maxStackSize = stackSize; ///set the stack size to
///the value specified by
///the parameter stackSize
stackTop = 0; ///set stackTop to 0
list = new Type[maxStackSize]; ///create the array to
///hold the stack elements
}///end constructor
template
stackType
{
delete [] list; ///deallocate the memory occupied
///by the array
}///end destructor
template
void stackType
(const stackType
{
delete [] list;
maxStackSize = otherStack.maxStackSize;
stackTop = otherStack.stackTop;
list = new Type[maxStackSize];
///copy otherStack into this stack
for (int j = 0; j < stackTop; j++)
list[j] = otherStack.list[j];
} ///end copyStack
template
stackType
{
list = nullptr;
copyStack(otherStack);
}///end copy constructor
template
const stackType
(const stackType
{
if (this != &otherStack) ///avoid self-copy
copyStack(otherStack);
return *this;
} ///end operator=
//#endif
//#include
//#include
//#include
//#include
//#include "myStack.h"
using namespace std;
int main()
{
///Step 1
double GPA;
double highestGPA;
string name;
stackType
ifstream infile;
infile.open("HighestGPAData.txt"); //Step 2
if (!infile) //Step 3
{
cout << "The input file does not "
<< "exist. Program terminates!"
<< endl;
return 1;
}
cout << fixed << showpoint; //Step 4
cout << setprecision(2); //Step 4
infile >> GPA >> name; //Step 5
highestGPA = GPA; //Step 6
while (infile) //Step 7
{
if (GPA > highestGPA) //Step 7.1
{
stack.initializeStack(); //Step 7.1.1
if (!stack.isFullStack()) //Step 7.1.2
stack.push(name);
highestGPA = GPA; //Step 7.1.3
}
else if (GPA == highestGPA) //Step 7.2
if (!stack.isFullStack())
stack.push(name);
else
{
cout << "Stack overflows. "
<< "Program terminates!"
<< endl;
return 1; //exit program
}
infile >> GPA >> name; //Step 7.3
}
cout << "Highest GPA = " << highestGPA
<< endl; //Step 8
cout << "The students holding the "
<< "highest GPA are:" << endl;
while (!stack.isEmptyStack()) //Step 9
{
cout << stack.top() << endl;
stack.pop();
}
cout << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
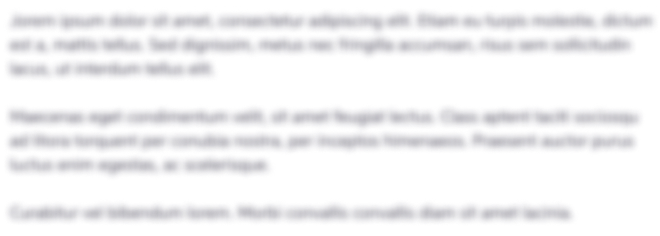
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started