Question
QUESTION : There are a variety of games possible with a deck of cards. For example, a deck of cards has four different suits, i.e.
QUESTION: There are a variety of games possible with a deck of cards. For example, a deck of cards has four different suits, i.e. spades (), clubs (), diamonds (), hearts (), and thirteen values for each suit. These decks of cards all have different number of categories and each category usually has the same number of cards.
Now write a function in python that uses list and for loop to create a deck of cards. Each element in the list will be a card, which is represented by a list containing the category as a string and the value as an int.
First, write a function called deck() that generates the deck of cards that contains all category in the categories list and each category should contain number_of_cards_per_cat starting from 1 to number_of_cards_per_cat as described in the doc string. You must follow the order of cards as listed in categories variable. If number_of_cards_per_cat is smaller than or equal to zero, then return an empty list.
Then write another function random_shuffle() that receives the list representing the deck of cards and return a randomly shuffled list but preserves the order of the categories. Your shuffled list should not be the same as the one generated from deck().
Finally, write a function reverse() that receives the list representing the deck of cards returns the list in reverse order.
TO DO: Download the file lab5.py, complete the functions inside according to their descriptions IMPORTANT: Do not change the file name or function names. Do not use input() or print(). Your file should not contain any additional lines of code outside the function definitions. Some example test cases are included in the docstrings.
HINTS:
You can generate N random integers between 1 and Max with the following lines of code: First, you should import the random library:
import random
then:
random.sample(range(1, Max), N)
return a list of N random numbers between 1 and Max
Couple of clarification points made:
1) In lab 5 random_shuffle() function, it is required to return a list that is not the same as the list passed in. For list that only has one item, namely, if in each category, you only have one card, then you don't need to shuffle that. You should return the same list as it is.
You can assume that the format of the input to random_shuffle() and reverse() are the same as the one generated from deck(), but the cards are not necessarily in order (e.g. [["spade", 1], ["spade", 3], ["spade", 2], ["heart", 3], ["heart", 1], ["heart", 2]]).
2) In random_shuffle() function, some of you randomize your list on the original list instead of creating a new list. This causes Markus to think that you return the same list instead of a shuffled list because we are comparing the input to your output. The lab handout does not explicitly state this is required, so we change our marking script to accommodate this situation. However, normally we don't want to change user inputs to a function (try this on your own if you did not implement it this way).
There is a small change to lab5 handout - we crossed out list comprehension - this was not taught so far, and we do not expect you to solve this lab with list comprehension (for loop and list methods will be just enough).
Finally, This is the initial code given:
import random
def deck(categories, num_of_cards_per_cat): """ (list, int) -> list Given a list of strings representation as category names and an integer indicating the number of cards in one category, return a deck of cards as a list. For example, with ["spades", "hearts"], 2, return a list of list: [["spades", 1],["spades",2], ["hearts", 1], ["hearts", 2]], where 1 and 2 are the index of the cards. >> deck(["spades", "hearts"], 2) [["spades", 1],["spades",2], ["hearts", 1], ["hearts", 2]] >> deck(["spades", "hearts"], -5) [] """
def random_shuffle(lst): """ (list) -> list Receives a deck of cards and returns a randomly ordered list containing all of the same elements. The returned list should preserve the order of the categories. See below console outputs as an example, where "s" and "d" stay in the same order as the input and only the numbers are shuffled. >> random_shuffle([["s",1],["s",2],["s",3],["d",1],["d",2],["d",3]]) [["s",3],["s",1],["s",2],["d",2],["d",1],["d",3]] """
def reverse(lst): """ (list) -> list Receives a list and returns a reverse ordered list containing all of the same elements. >> reverse([["spades", 1],["spades",2], ["hearts", 1], ["hearts", 2]]) [["hearts", 2], ["hearts", 1], ["spades", 2],["spades",1]] """
Step by Step Solution
There are 3 Steps involved in it
Step: 1
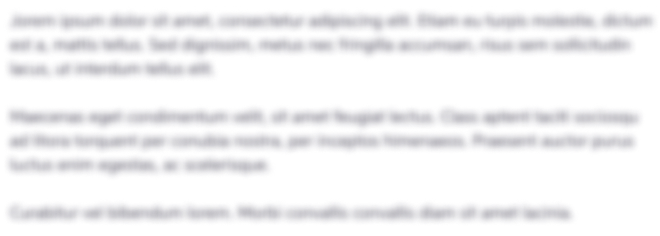
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started