Question
Question Write Pseudocode for this code. # Import matplotlib,pyplot import matplotlib.pyplot as plt # Import random import random # Function used to simulate the dice
Question
Write Pseudocode for this code.
# Import matplotlib,pyplot import matplotlib.pyplot as plt
# Import random import random
# Function used to simulate the dice from number of rolls and number of dice def dice_simulation(num_rolls, num_dice): # Create an empty dictionary to store the count of roll roll_count = {}
# Loop for each value of i from number of dice to 6 times number of dice for i in range(num_dice, (num_dice*6) + 1): # Store the 'i' as key and 0 as value roll_count[i] = 0
# Loop for each roll i from 0 to rolls-1 for i in range(num_rolls): # Generate a random outcome of roll between number of dice and 6 times number of dice num_rolls = random.randint(num_dice, num_dice*6)
# Increase the roll count by 1 roll_count[num_rolls] += 1
# Return the dictionary with key as roll and value as count return roll_count
# Call function to simulate the roll dice for 1 dice 100 times Roll100Dice1 = dice_simulation(100, 1)
# Call function to simulate the roll dice for 4 dice 100 times Roll100Dice4 = dice_simulation(100, 4)
# Call function to simulate the roll dice for 1 dice 1000 times Roll1000Dice1 = dice_simulation(1000, 1)
# Call function to simulate the roll dice for 4 dice 100 times Roll1000Dice4 = dice_simulation(1000, 4)
# Create figure with 4 subplots figure, axis = plt.subplots(2, 2, figsize=(15, 10))
# Create a list of rolls outcome from keys of dictionary for 1 dice 100 times x = list(Roll100Dice1.keys())
# Create a list of rolls outcome from values of dictionary for 1 dice 100 times y = list(Roll100Dice1.values())
# Plot the bar chart after 100 simulation of 1 dice axis[0, 0].bar(x, y) axis[0, 0].set_title('After 100 simulation of 1 dice')
# Create a list of rolls outcome from keys of dictionary for 4 dice 100 times x = list(Roll100Dice4.keys())
# Create a list of rolls outcome from values of dictionary for 4 dice 100 times y = list(Roll100Dice4.values())
# Plot the bar chart after 100 simulation of 4 dice axis[1, 0].bar(x, y) axis[1, 0].set_title('After 100 simulation of 4 dice')
# Create a list of rolls outcome from keys of dictionary for 1 dice 1000 times x = list(Roll1000Dice1.keys())
# Create a list of rolls outcome from values of dictionary for 1 dice 1000 times y = list(Roll1000Dice1.values())
# Plot the bar chart after 1000 simulation of 1 dice axis[0, 1].bar(x, y) axis[0, 1].set_title('After 1000 simulation of 1 dice')
# Create a list of rolls outcome from keys of dictionary for 4 dice 1000 times x = list(Roll1000Dice4.keys())
# Create a list of rolls outcome from values of dictionary for 4 dice 1000 times y = list(Roll1000Dice4.values())
# Plot the bar chart after 1000 simulation of 4 dice axis[1, 1].bar(x, y) axis[1, 1].set_title('After 1000 simulation of 4 dice')
# Show the plot plt.show()
# Used to store the total count of even outcome of 100 simulation of 1 dice even_total = 0
# Used to store the total count of odd outcome of 100 simulation of 1 dice odd_total = 0
for x in Roll100Dice1: # Loop for each outcome x of 1000 simulation of 1 dice
if x % 2 == 0: # If the outcome x is even
even_total += Roll100Dice1[x] # Add the count with total even
else: # If the outcome x is odd
odd_total += Roll100Dice1[x] # Add the count with total odd
# Compute the probability of outcome is even for 1 dice roll Roll100Dice1EvenProb = even_total / 100
# Compute the probability of outcome is odd for 1 dice roll Roll100Dice1OddProb = odd_total / 100
# Used to store the total count of even outcome of 100 simulation of 4 dice even_total = 0
# Used to store the total count of odd outcome of 100 simulation of 4 dice odd_total = 0
for x in Roll100Dice4: # Loop for each outcome x of 100 simulation of 4 dice
if x % 2 == 0: # If the outcome x is even
even_total += Roll100Dice4[x] # Add the count with total even
else: # If the outcome x is odd
odd_total += Roll100Dice4[x] # Add the count with total odd
# Compute the probability of outcome is even for 4 dice roll Roll100Dice4EvenProb = even_total / 100
# Compute the probability of outcome is odd for 4 dice roll Roll100Dice4OddProb = odd_total / 100
# Used to store the total count of even outcome of 1000 simulation of 1 dice even_total = 0
# Used to store the total count of odd outcome of 1000 simulation of 1 dice odd_total = 0
for x in Roll1000Dice1: # Loop for each outcome x of 1000 simulation of 1 dice
if x % 2 == 0: # If the outcome x is even
even_total += Roll1000Dice1[x] # Add the count with total even
else: # If the outcome x is odd
odd_total += Roll1000Dice1[x] # Add the count with total odd
# Compute the probability of outcome is even for 1 dice roll Roll1000Dice1EvenProb = even_total / 1000
# Compute the probability of outcome is odd for 1 dice roll Roll1000Dice1OddProb = odd_total / 1000
# Used to store the total count of even outcome of 1000 simulation of 4 dice even_total = 0
# Used to store the total count of odd outcome of 1000 simulation of 4 dice odd_total = 0
for x in Roll1000Dice4: # Loop for each outcome x of 1000 simulation of 4 dice
if x % 2 == 0: # If the outcome x is even
even_total += Roll1000Dice4[x] # Add the count with total even
else: # If the outcome x is odd
odd_total += Roll1000Dice4[x] # Add the count with total odd
# Compute the probability of outcome is even for 4 dice roll Roll1000Dice4EvenProb = even_total / 1000
# Compute the probability of outcome is odd for 4 dice roll Roll1000Dice4OddProb = odd_total / 1000
# Print the probability of outcome is even for 1 dice roll print('The probability of outcome is even for 1 dice roll:', Roll100Dice1EvenProb)
# Print the probability of outcome is odd for 1 dice roll print('The probability of outcome is odd for 1 dice roll:', Roll100Dice1OddProb)
# Print the probability of outcome is even for 4 dice roll print('The probability of outcome is even for 4 dice roll:', Roll100Dice4EvenProb)
# Print the probability of outcome is even for 4 dice roll print('The probability of outcome is odd for 4 dice roll:', Roll100Dice4OddProb)
# Print the probability of outcome is even for 1 dice roll print('The probability of outcome is even for 1 dice roll:', Roll1000Dice1EvenProb)
# Print the probability of outcome is odd for 1 dice roll print('The probability of outcome is odd for 1 dice roll:', Roll1000Dice1OddProb)
# Print the probability of outcome is even for 4 dice roll print('The probability of outcome is even for 4 dice roll:', Roll1000Dice4EvenProb)
# Print the probability of outcome is even for 4 dice roll print('The probability of outcome is odd for 4 dice roll:', Roll1000Dice4OddProb)
print("This is the end of the program.") # End message for the program
Step by Step Solution
There are 3 Steps involved in it
Step: 1
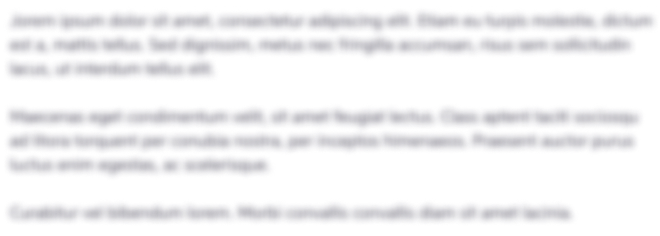
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started