Question
Question: Write the body for a function that replaces each copy of an item in a stack with another item.Use the following specification. (This function
Question: Write the body for a function that replaces each copy of an item in a stack with another item.Use the following specification. (This function is in the client program.) ReplaceItem(StackType& stack, ItemType oldItem, ItemType newItem)
Function: Replaces ALL occurrences of oldItem with newItem.Precondition: Stack has been initializedPostcondition: Each occurrence of oldItem in stack has been replaced by newItem.
// StackType.h
#include "ItemType.h"
class FullStack {
};
class EmptyStack {
};
class StackType { public: StackType(int max); StackType(); bool isFull() const; bool isEmpty() const; void Push(ItemType item); void Pop(); ItemType Top() const; void ReplaceItem(StackType &stack, ItemType olditem, ItemType newItem); ~StackType(); private: int top; int maxStack; ItemType *items; };
// StackType.cpp
#include "StackType.h"
StackType::StackType(int max) { maxStack = max; top = -1; items = new ItemType[maxStack]; }
StackType::StackType() { maxStack = 10; top = -1; items = new ItemType[maxStack]; }
bool StackType::isFull() { return (top == maxStack - 1); }
StackType::~StackType() { delete [] items; }
void StackType::Pop() { if(isEmpty()) throw EmptyStack();
top--; }
ItemType StackType::Top() const { if(isEmpty()) throw EmptyStack();
return items[top]; }
void StackType::Push(ItemType newItem) { if(isFull()) throw FullStack();
top++; items[top] = newItem; }
void StackType::isEmpty() { return (top == -1); }
void StackType::ReplaceItem(StackType &stack, ItemType oldItem, ItemType newItem) { // create a temporary stack StackType tempStack; ItemType tempItem;
while(!stack.isEmpty()) { // get the top element tempItem = stack.Top(); stack.Pop();
if(tempItem.value == oldItem.value) { // add the item to the temporary stack tempStack.Push(newItem); } else { tempStack.Push(tempItem); } }
// restore stack while(!tempStack.isEmpty()) { // get the top element tempItem = tempStack.Top(); tempStack.Pop();
// push the items back to the original stack stack.Push(tempItem) } }
// ItemType.h
class ItemType { public: ItemType(); void Initialize(int number); public: int value; };
// ItemType.cpp
#include "ItemType.h"
ItemType::ItemType() { value = 0; }
void ItemType::Initialize(int number) { value = number; }
// driver file
#include "StackType.h" #include
int main() { StackType stack; ItemType item;
cout << "Adding elements on the stack..." << endl; cout << "Pushing 1" << endl; item.Initialize(1); stack.Push(item);
cout << "Pushing 2" << endl; item.Initialize(2); stack.Push(item);
cout << "Pushing 3" << endl; item.Initialize(3); stack.Push(item);
cout << "Pushing 2" << endl; item.Initialize(2); stack.Push(item);
cout << "Pushing 5" << endl; item.Initialize(5); stack.Push(item);
cout << " Calling ReplaceItem with oldItem as 2 and newItem as 7" << endl; item.Initialize(2); Itemtype item2; item2.Initialize(7); ReplaceItem(stack, item, item2);
cout << " Stack is ";
while(!stack.isEmpty()) { cout << stack.Top() << endl; stack.Pop(); }
return 0; }
The program is incomplete, how to finish this to get an output below?
Adding elements on the stack...
Pushing 1
Pushing 2
Pushing 3
Pushing 2
Pushing 5
Calling ReplaceItem with oldItem as 2 and newItem as 7
Stack is
5
7
3
7
1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
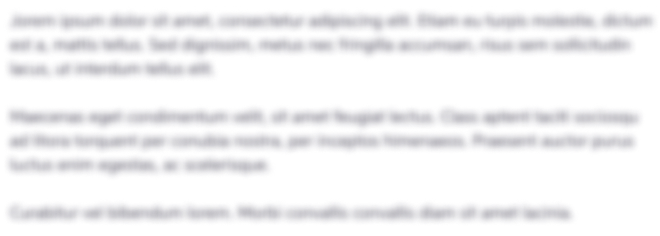
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started