Question
Queue using two stacks ( in Java) Implement a MyQueue class which implements a queue using two stacks . private Stack stack1; private Stack stack2;
Queue using two stacks ( in Java)
Implement a MyQueue class which implements a queue using two stacks.
private Stack
private Stack
Note:
You can use java.util.Stack but you are not allowed to use java.util.Queue and any of its methods
Your Queue should not accept null or empty String or space as an input
Trim your inputs first
for example value = " b " should convert to value = "b"
You can not add any custom test case for this problem. 10 different test cases already provided in the QueueTest main method which your code will be run against them
You need to implement the following methods using stack1 and stack2 and also you can add more methods as well:
public int size()
public void insert(String value)
public String peek()
public String remove()
private void shiftStacks()
public boolean isEmpty()
Sample Test:
/*
* Test case number: SampleTest1
* Test case values: push 1,2,3,4 into a queue, and pop two times, then push 5,6 into the queue
* Expected output (Post-state): [3, 4, 5, 6]
*/
public String SampleTest1() {
MyQueue q = new MyQueue();
q.insert("1");
q.insert("2");
q.insert("3");
q.insert("4");
q.remove();
q.remove();
q.insert("5");
q.insert("6");
return q.toString();
}
/*
* Test case number: SampleTest2
* Test case values: push 1, 2 and 3 into a queue with 2 spaces
* Expected output (Post-state): [3]
*/
public String SampleTest2(){
MyQueue q = new MyQueue();
q.insert("1 ");
q.insert("2");
q.insert("3");
q.insert("");
q.insert(" ");
q.remove();
q.remove();
return q.toString();
}
The following section of code is the main function, this section cannot be modified at all.
class QueueTest{ public static void main(String args[]){ Scanner input = new Scanner(System.in); String oracle = input.nextLine(); int i = input.nextInt();
Class qTest = QueueTest.class; Object grader = null; try { grader = qTest.newInstance(); } catch (Exception e1) { e1.printStackTrace(); } String test = "test" + i; try{ Method actualOutput = qTest.getMethod(test, null); if(grader!=null){ if (actualOutput.invoke(grader, null).equals(oracle)) { System.out.println("Test " + i + " Passed"); }else{ System.out.println("Test " + i + " failed"); } } }catch(Exception e){ System.out.println("Test " + i + " failed"); }
}
/* * Test case number: 1 * Test case values: push 1 and 2 into the queue * Expected output (Post-state): [1, 2] */
public String test1(){ MyQueue q = new MyQueue(); q.insert("1"); q.insert("2"); return q.toString(); }
/* * Test case number: 2 * Test case values: push 1 and 2 into the queue, then pop the first item * Expected output (Post-state): [2] */
public String test2(){ MyQueue q = new MyQueue(); q.insert("1"); q.insert("2"); q.remove(); return q.toString(); }
/* * Test case number: 3 * Test case values: push 1, 2 and 3 into a queue with 2 spaces * Expected output (Post-state): [3] */ public String test3(){ MyQueue q = new MyQueue(); q.insert("1"); q.insert("2"); q.insert("3"); q.insert(""); q.insert(""); q.remove(); q.remove(); return q.toString(); }
/* * Test case number: 4 * Test case values: push null value into the queue * Expected output (Post-state): [] */ public String test4() { MyQueue q = new MyQueue(); q.insert(null); return q.toString(); }
/* * Test case number: 5 * Test case values: push 1 into a queue with 2 spaces, check if the queue is empty * Expected output (Post-state): return false, the queue is not empty */
public String test5() { MyQueue q = new MyQueue(); q.insert("1"); q.insert(" "); q.insert(" "); return String.valueOf(q.isEmpty()); }
/* * Test case number: 6 * Test case values: push 1 into a queue with 2 spaces, check if the queue size is zero * Expected output (Post-state): */
public String test6() { MyQueue q = new MyQueue(); q.insert("1"); q.insert(" "); q.insert(" ");
return String.valueOf(q.size() == 0); }
/* * Test case number: 7 * Test case values: initialize a new queue, check if the queue is empty * Expected output (Post-state): */
public String test7() { MyQueue q = new MyQueue(); return String.valueOf(q.isEmpty()); }
/* * Test case number: 8 * Test case values: push 1,2,3,4 into a queue, and pop the first item * check the peek of the queue * Expected output (Post-state): */ public String test8() { MyQueue q = new MyQueue(); q.insert("1"); q.insert("2"); q.insert("3"); q.insert("4"); q.remove();
return q.peek(); }
/* * Test case number: 9 * Test case values: push 1,2,3,4 into a queue, and pop four times, then push 5 into the queue * check the peek of the queue * Expected output (Post-state): */ public String test9() { MyQueue q = new MyQueue(); q.insert("1"); q.insert("2"); q.insert("3"); q.insert("4"); q.remove(); q.remove(); q.remove(); q.remove(); q.insert("5");
return q.peek(); }
/* * Test case number: 10 * Test case values: push 1,2,3,4 into a queue, and pop two times, then push 5,6 into the queue * Expected output (Post-state): */ public String test10() { MyQueue q = new MyQueue(); q.insert("1"); q.insert("2"); q.insert("3"); q.insert("4"); q.remove(); q.remove(); q.insert("5"); q.insert("6");
return q.toString(); }
}
MyQueue, referenced from main is as follows.
The code is to be written in the MyQueue class.
class MyQueue{ private Stack
Step by Step Solution
There are 3 Steps involved in it
Step: 1
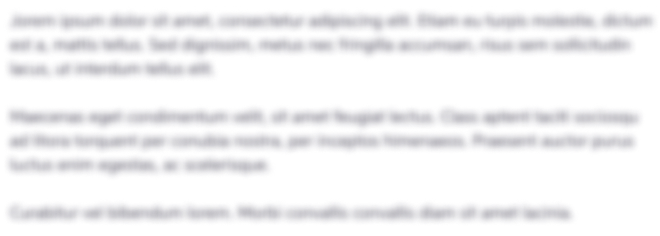
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started