Question
Quicksort for Date Objects Add an override of toString() method to the class that prints out date in the form December 25, 2016. Make Date
Quicksort for Date Objects
Add an override of toString() method to the class that prints out date in the form December 25, 2016.
Make Date class implement Comparable interface (https://docs.oracle.com/javase/8/docs/api/java/lang/Comparable.html). For comparison use an obvious rule: the earlier date is smaller than the later one.
Modify Quicksort implementation in such a way that it becomes a generic method and can work with objects that implement Comparable interface. Study source code samples from Chapter 17 folder to find a good sample code for this task.
Use loop and random number generator to create an array of 100 dates in the time range from January 1 2018 to December 31 2018.
Use Quicksort generic method to sort the dates in the array.
Print out the original array and the array after it has been sorted
Date.Java
public class Date { private String month; private int day; private int year; //a four digit number.
public Date( ) { month = "January"; day = 1; year = 1000; }
public Date(int monthInt, int day, int year) { setDate(monthInt, day, year); }
public Date(String monthString, int day, int year) { setDate(monthString, day, year); }
public Date(int year) { setDate(1, 1, year); }
public void setDate(int monthInt, int day, int year) { if (dateOK(monthInt, day, year)) { this.month = monthString(monthInt); this.day = day; this.year = year; } else { throw new IllegalArgumentException("Bad date!");
} }
public void setDate(String monthString, int day, int year) { if (dateOK(monthString, day, year)) { this.month = monthString; this.day = day; this.year = year; } else { throw new IllegalArgumentException("Bad date!"); } }
public void setDate(int year) { setDate(1, 1, year); }
public void setYear(int year) { if ( (year < 1000) || (year > 9999) ) { throw new IllegalArgumentException("Bad year!"); } else this.year = year; } public void setMonth(int monthNumber) { if ((monthNumber <= 0) || (monthNumber > 12)) { throw new IllegalArgumentException("Bad month!"); } else month = monthString(monthNumber); }
public void setDay(int day) { if ((day <= 0) || (day > 31)) { throw new IllegalArgumentException("Bad day!"); } else this.day = day; }
public int getMonth( ) { if (month.equals("January")) return 1; else if (month.equals("February")) return 2; else if (month.equalsIgnoreCase("March")) return 3; else if (month.equalsIgnoreCase("April")) return 4; else if (month.equalsIgnoreCase("May")) return 5; else if (month.equals("June")) return 6; else if (month.equalsIgnoreCase("July")) return 7; else if (month.equalsIgnoreCase("August")) return 8; else if (month.equalsIgnoreCase("September")) return 9; else if (month.equalsIgnoreCase("October")) return 10; else if (month.equals("November")) return 11; else if (month.equals("December")) return 12; else { throw new IllegalArgumentException("Bad month!"); } }
public int getDay( ) { return day; }
public int getYear( ) { return year; }
private boolean dateOK(int monthInt, int dayInt, int yearInt) { return ( (monthInt >= 1) && (monthInt <= 12) && (dayInt >= 1) && (dayInt <= 31) && (yearInt >= 1000) && (yearInt <= 9999) ); }
private boolean dateOK(String monthString, int dayInt, int yearInt) { return ( monthOK(monthString) && (dayInt >= 1) && (dayInt <= 31) && (yearInt >= 1000) && (yearInt <= 9999) ); }
private boolean monthOK(String month) { return (month.equals("January") || month.equals("February") || month.equals("March") || month.equals("April") || month.equals("May") || month.equals("June") || month.equals("July") || month.equals("August") || month.equals("September") || month.equals("October") || month.equals("November") || month.equals("December") ); }
private String monthString(int monthNumber) { switch (monthNumber) { case 1: return "January"; case 2: return "February"; case 3: return "March"; case 4: return "April"; case 5: return "May"; case 6: return "June"; case 7: return "July"; case 8: return "August"; case 9: return "September"; case 10: return "October"; case 11: return "November"; case 12: return "December"; default: throw new IllegalArgumentException("Bad month number!");
} } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
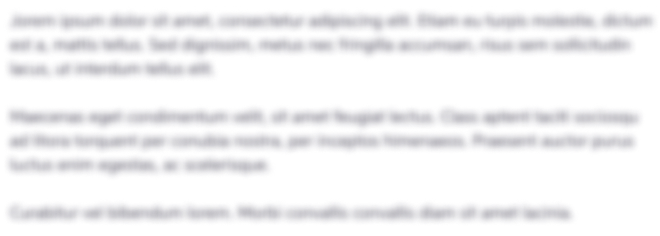
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started