Question
read and write text files containing the names of several large companies, their stock symbols, and the price of their stocks: 1. Create a text
read and write text files containing the names of several large companies, their stock symbols, and the price of their stocks:
1. Create a text file containing the company name (for example, Apple), its stock symbol (APPL), and the price of its stock for several companies on a stock exchange.
2. Create a class named Stock with instance variables to hold the company name, the stock symbol, and the stocks price for each company in the text file
3. Create an array which can hold (up to) 100 Stock objects
4. Write a method which uses either a Scanner or a BufferedReader to read a text file containing a list of stock names, symbols, and prices into an array of Stock objects.
5. Decrease all of the stock prices by 1/3.
6. Write another method which uses a PrintWriter to write the same data onto a second text file.
7. Clear the array of Stock objects
8. Use the same method from step 4 above to read the data just written (with the decreased prices) into the array of Stock objects again
9. Increase the prices of the stocks back to their original values (plus or minus a penny)
10. Use the method from step 6 to write them back onto a third text file.
Step 1: Create the text file
First, create a new workspace, project, and program named Lab4 in JCreator. This should create a src and a classes folder for your project.
In the classes folder, create a text file name stocks1.txt with the following contents:
Line 1: Apple,AAPL,152.70
Line 2: Alphabet,GOOGL,873.96
Line 3: IBM,IBM,194.37
Line 4: Microsoft,MSFT,65.67
Line 5: Oracle,ORCL,62.82
Important: Make sure that you dont have any spaces before or after the commas.
Step 2: Create a class named Stock
In the same project, create a new class named Stock. It should have 3 instance variables
1. a String containing the company name (e.g., Apple)
2. a String containing the stock symbol (e.g., AAPL)
3. a double containing the stock price
Add a full constructor, all getters and setters, equals, and toString.
Step 3: Create an array of 100 Stock objects
Leave the array empty
Step 4: Read the text file containing the list of stocks using either a Scanner or a BufferedReader and put into the array of Stock objects
4a: If using a Scanner
Write and invoke a method named readStocksWithScanner which populates the array with the contents of the file stocks1.txt created above in step 1.
Its two parameters are the name of the file (stocks1.txt) and the array of Stock objects
This method will use a Scanner object to read data from the file
o We cant simply pass the file name to the Scanners constructor, as that String would then be used by the Scanner (rather than being interpreted as the name of a text file).
o Instead, create a FileInputStream that opens the file. You should use a try/catch statement in case the file cant be opened
o Once opened, assign the FileInputStream object to the Scanner
To read each line in the file:
o set the delimiter of the Scanner to a comma: useDelimiter(",")
o read the company name using next()
o read the stock symbol using next()
o set the delimiter to white space: useDelimiter("[,\\s]")
o read the stocks price using nextDouble()
o use nextLine() to remove the dangling
o create a Stock object with this information and add to the array of Stock objects
Use the hasNextLine() method to read all of the stocks from the file
Close the FileInputStream when done
4b: If using a BufferedReader
Write and invoke a method named readStocksWithBufferedReader that populates the array using a BufferedReader object to read the contents of the file named stocks1.txt
Its two parameters are the name of the file and the array of Stock objects
It will use a BufferedReader object to read data from the file
o Create a FileReader that opens the file. You should use a try/catch statement in case the file cant be opened
o Once opened, assign the BufferedReader object using the FileReader
To read each line in the file:
o Use the readLine method to read each entire line into a String
o Create a StringTokenizer object on the String just read, using a comma as the delimiter
o Use the nextToken method of the StringTokenizer to return the company name
o Use the nextToken method again to return the stock symbol
o Use the nextToken method one more time to return the price of the stock in a String, then use Double.parseDouble (or any other method of your choice) to convert the String to a double.
o Create a new Stock object using the above data and add to the array of Stock objects
Stop reading lines when readLine returns a null
Close the FileReader when done
Note: The BufferedReader, FileReader, and all associated exceptions are in java.io. Please import all classes individually, (i.e., do not use the blanket import statement import java.io.*) .
Step 5: Decrease the prices of all of the stocks by 1/3
Create a method named updateStockPrices which updates the prices of all stocks in the array by a specified multiplier
Its two parameters are a double multiplier and the array of Stock objects.
It should skip over any element in the array that hasnt been instantiated
All prices should be rounded to 2 decimal places. (Hint: use the Math.round method)
Invoke this method so that the price of every stock in the array is reduced by 1/3.
Step 6: Write the stocks onto a new file named stock2.txt
Write and invoke a method named writeStocks which creates a new file name stocks2.txt with the new stock prices. Use the same format as used in the stocks1.txt file.
This methods two parameters are the name of the target file (stocks2.txt) and the array of Stock objects
Use a PrintWriter to create the new file with the stock names, symbols, and new prices.
Since you arent appending to an existing file, simply use the constructor which accepts a String containing the name of the file to be written onto by the PrintWriter.
Prices should be printed to 2 decimal places
Close the PrintWriter when done
Step 7: Clear the array of Stock objects
Set all elements in the array to null
Step 8: Repopulate the array
Invoke the method you wrote in Step 4 again, except read from the stock2.txt file.
Extra credit: Write and invoke the alternate method of reading from the file in this step. (Do this last!)
Step 9: Restore the price of each stock back to its original value
Invoke the method you wrote in Step 5 again, this time increasing the prices of the stocks:
Use a multiplier that will restore the prices to their original values (plus or minus a penny).
What will this multiplier be? Please document this in your code.
Step 10: Write the stocks onto a new file named stocks3.txt
Invoke the method you wrote in Step 6 again using a file name stocks3.txt with the new stock prices. The contents of this file should be identical to the contents of the original stocks1.txt file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
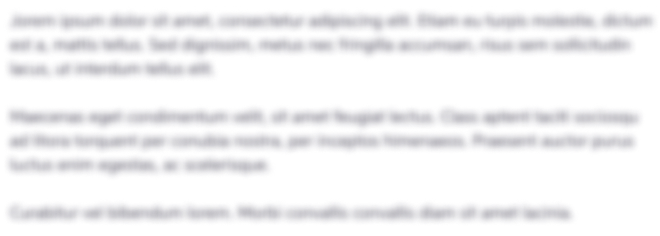
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started