Question
Read carefully. You are to write a class called Card , which will implement a playing card. Your Card class should implement the Comparable interface
Read carefully.
You are to write a class called Card, which will implement a playing card. Your Card class should implement the Comparable interface (which is built into Java). So it should be declared like this:
public class Card implements Comparable
A Card will have the following:
Data:
An int that holds the rank. Ranks will be 2-14, where 2-10 will be those actual numbers, 11 will be a Jack, 12 will be a Queen, 13 will be a King, and 14 will be an Ace. So Ace will be the high card in a suit.
A char that holds the suit. Suits will be C or H or D or S, which will represent Clubs, Hearts, Diamonds, or Spades. Note that it should be the uppercase char and also not a String.
Constructors:
A default constructor that will initialize the rank to 14 and the suit to S (the Ace of Spades).
A parameterized constructor that will receive the rank (as an int) and the suit (as a char). If the rank is outside the range 2-14, then
throw new IllegalArgumentException(
If the suit is != C and != D and != H and != S then
throw new IllegalArgumentException(
If the rank and suit are OK, then initialize the data to what is received.
A copy constructor that will receive another Card. It should initialize its data to be the same as the Card that was received.
Methods:
A toString() method that returns a String representing the Card. It should be of the form rank, then suit. If the rank is >10, it should use J, Q, K, or A instead of the number. Examples are:
4S 8D QC KS 2H AH
(for example, QC would be returned when the rank was 12 and the suit was C)
(for example, AH would be returned when the rank was 14 and the suit was H)
A method called compareTo which receives a Card and returns an int that is the result of comparing the current instance to the Card that was received.
If the current instances rank is larger than the rank of the Card that was received, then a positive int is returned.
If the current instances rank is smaller than that of the Card that was received, then a negative int is returned.
If they are the same rank, then 0 is returned.
A method called equals(Object obj), which receives an Object and returns true if its rank and suit are both the same as the rank and suit of the Object (Card) that was received. It should be implemented like we did in class.
Here's the tester!
public class CardTester
{
public static void main(String[ ] args)
{
//get the arguments for the test
java.util.Scanner kb = new java.util.Scanner(System.in);
String test = kb.nextLine();
//***************************************************
//***************************************************
if (test.equalsIgnoreCase("1. testing parameterized constructor"))
{
boolean printDescription = true;
boolean checkChanges = true;
try
{
char[ ] suitArray = { 'C', 'H', 'D', 'S' };
for (int r=2; r<=14; r++)
{
System.out.println(); //new line
if (printDescription)
System.out.println("==>Testing parameterized constructor/toString(): passing in rank of " + r + " and then each suit (one at a time)");
for (int suitIndex=0; suitIndex { char thisSuit = suitArray[suitIndex]; Card thisCard = new Card(r, thisSuit); //create new Card System.out.print("\t" + thisCard); } } } catch (Throwable ex) { //System.out.println("\t" + ex); System.out.println("\t" + ex.getClass().getName()); } } //*************************************************** //*************************************************** else if (test.equalsIgnoreCase("2. testing illegal arguments to parameterized constructor")) { boolean printDescription = true; boolean checkChanges = true; int[ ] rankArray = { 1, 2, 14, 15 }; char[ ] suitArray = { 'c', 'd', 'h', 'S', 's'}; for (int rankIndex=0; rankIndex for (int suitIndex=0; suitIndex { try { int thisRank = rankArray[rankIndex]; char thisSuit = suitArray[suitIndex]; if (printDescription) System.out.println("==>Testing parameterized constructor/toString(): passing in rank of " + thisRank + " and suit of " + thisSuit); Card thisCard = new Card(thisRank, thisSuit); //create new Card System.out.println("\t" + thisCard); } catch (Throwable ex) { //System.out.println("\t" + ex); System.out.println("\t" + ex.getClass().getName()); } } } //*************************************************** //*************************************************** else if (test.equalsIgnoreCase("3. testing other constructors")) { boolean printDescription = true; boolean checkChanges = true; try { if (printDescription) System.out.println("==>Testing default constructor/toString()"); Card theCard = new Card(); //create new Card System.out.println(theCard); } catch (Throwable ex) { System.out.println(ex.getClass().getName()); } //---------------------------- try { int[ ] rankArray = { 5, 12 }; char[ ] suitArray = { 'S', 'D' }; for (int rankIndex=0; rankIndex for (char suitIndex=0; suitIndex { int thisRank = rankArray[rankIndex]; char thisSuit = suitArray[suitIndex]; Card argCard = new Card(thisRank, thisSuit); //create argCard if (printDescription) System.out.println("==>Testing copy constructor/toString(): passing in " + argCard); Card c0 = new Card(argCard); //create new Card, copying argCard System.out.println(c0); } } catch (Throwable ex) { System.out.println(ex.getClass().getName()); } } //*************************************************** //*************************************************** else if (test.equalsIgnoreCase("4. testing compareTo")) { boolean printDescription = true; boolean checkChanges = true; boolean mistake = false; boolean cardChanged = false; int numPassed = 0; int numFailed = 0; try { char[ ] suitArray = { 'C', 'H', 'D', 'S' }; for (int r=2; r<=14; r++) for (char s=0; s { //create a card int theCardRank = r; char theCardSuit = suitArray[s]; Card theCard = new Card(theCardRank, theCardSuit); String theCardOrigToString = theCard.toString(); //check to see if .compareTo a bigger Card works for (int biggerRank=r+1; biggerRank<=14; biggerRank++) for (int suitIndex=0; suitIndex { Card biggerCard = new Card(biggerRank, suitArray[suitIndex]); String biggerCardOrigToString = biggerCard.toString(); int result = theCard.compareTo(biggerCard); if (result < 0) numPassed++; else { numFailed++; // System.out.println("testing " + theCard + ".compareTo(" + biggerCard + ") result = " + result); } if (!theCardOrigToString.equals(theCard.toString()) || !biggerCardOrigToString.equals(biggerCard.toString())) cardChanged = true; } //check to see if .compareTo a bigger Card works for (int smallerRank=2; smallerRank for (int suitIndex=0; suitIndex { Card smallerCard = new Card(smallerRank, suitArray[suitIndex]); String smallerCardOrigToString = smallerCard.toString(); int result = theCard.compareTo(smallerCard); if (result > 0) numPassed++; else { numFailed++; // System.out.println("testing " + theCard + ".compareTo(" + smallerCard + ") result = " + result); } if (!theCardOrigToString.equals(theCard.toString()) || !smallerCardOrigToString.equals(smallerCard.toString())) cardChanged = true; } //check to see if .compareTo the same rank works for (int suitIndex=0; suitIndex { Card sameCard = new Card(r, suitArray[suitIndex]); String sameCardOrigToString = sameCard.toString(); int result = theCard.compareTo(sameCard); if (result == 0) numPassed++; else { numFailed++; // System.out.println("testing " + theCard + ".compareTo(" + sameCard + ") result = " + result); } if (!theCardOrigToString.equals(theCard.toString()) || !sameCardOrigToString.equals(sameCard.toString())) cardChanged = true; } } } catch(Throwable ex) { System.out.println(ex); // System.out.println(ex.getClass().getName()); mistake = true; } //print results System.out.println("Tested every card .compareTo every other card"); System.out.println("Tests Passed = " + numPassed + " Tests Failed = " + numFailed); if (cardChanged) System.out.println("...BUT the original Card and/or the argument Card changed in some test(s)!"); } //*************************************************** //*************************************************** else if (test.equalsIgnoreCase("5. testing equals")) { boolean printDescription = true; boolean checkChanges = true; int rankArg1 = 8; char[ ] suitArray = { 'C', 'D', 'H', 'S' }; int suitIndex = 0; char suitArg2 = suitArray[suitIndex]; Card c0 = new Card(rankArg1, suitArg2); String c0OrigtoString = c0.toString(); if (printDescription) System.out.println("create " + c0 + " and then ask it if it equals: \t\tnull, " + " \t\tthe STRING " + c0 + " \t\ta Card with the same rank but different suit " + " \t\ta Card with a different rank but same suit " + " \t\ta Card with different rank and different suit " + " \t\ta Card with the same rank and same suit "); Object[ ] argList = { null, c0.toString(), new Card(rankArg1, suitArray[suitIndex+1]), new Card(rankArg1+2, suitArg2), new Card(rankArg1+1, suitArray[suitIndex+1]), new Card(rankArg1, suitArg2) }; for (int argIndex=0; argIndex { System.out.println("Part " + argIndex + ": ask " + c0 + " if it equals " + argList[argIndex] + "\t\t"); String argOrigtoString = ""; if (argList[argIndex] != null) argOrigtoString = argList[argIndex].toString(); try { boolean ans = c0.equals(argList[argIndex]); System.out.println(ans); } catch(Throwable ex) { System.out.println(ex.getClass().getName()); } finally { if (argList[argIndex] != null) { if (!c0.toString().equals(c0OrigtoString) || !argList[argIndex].toString().equals(argOrigtoString)) System.out.println("...but the original Card and/or the argument were changed."); } else if (!c0.toString().equals(c0OrigtoString)) System.out.println("...but the original Card was changed."); } } } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
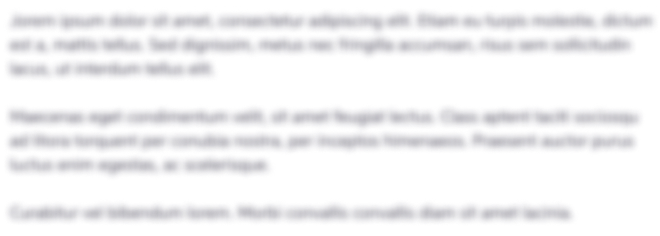
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started